Chuck's Debugger: Fix your code faster
Error in React component
Programming Languages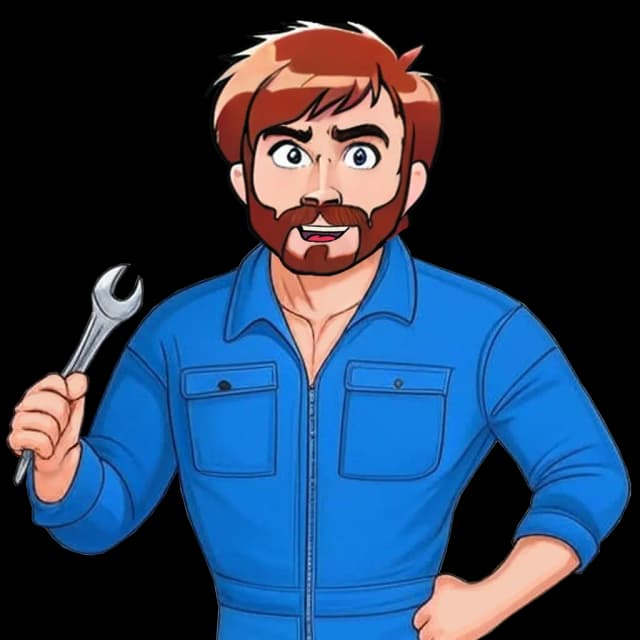
Home.js:27 Uncaught TypeError: Cannot read properties of undefined (reading 'results') at Home (Home.js:27) at renderWithHooks (react-dom.development.js:14985) at mountIndeterminateComponent (react-dom.development.js:17811) at beginWork (react-dom.development.js:19049)
Suggested Fix
Possible Solution for "Uncaught TypeError: Cannot read properties of undefined"
This error indicates that the code is trying to read a property from an undefined or null value. In the provided code snippet, the error is occurring on line 27 of Home.js
where it's trying to access the results
property of an object that seems to be undefined.
To fix this issue, you should ensure that the state
object is always initialized with the initialState
object in the useHomeFetch
custom hook. It seems that the state
is never initialized with any value, causing the results
property to be undefined.
You can update the useState
hook to initialize state
with initialState
:
javascript
By initializing the state
with the initialState
object, you ensure that the results
property is available when accessing state.results
. This should resolve the "Uncaught TypeError" issue in your React component.
Did you find this answer useful?
Chuck's Debugger is a free resource and we rely on donations to keep it running.
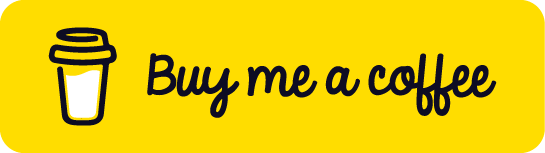
Do you still have issues?
You can use Chuck's Debugger yourself to find a solution. Just enter the error message or problem and our AI will analyze and suggest a solution!
Use our AI debugger