Graphs with D3
Binding Data to Elements in D3
One of the most powerful features of D3 is the ability to bind data to DOM elements and manipulate those elements based on the data. This process is called "data binding" and is fundamental for creating dynamic and efficient visualizations. In this chapter, we will explore how D3 manages data binding and how you can leverage this functionality to build complex visualizations.
Basic Data Binding
data
and enter
D3's data
method allows you to bind a set of data to a selection of DOM elements. When the data is bound, we can use the enter
method to create new elements for each datum in the set.
javascript
In this example:
selectAll("p")
tries to select all<p>
elements in the body, even if they don’t exist.data(data)
binds thedata
set to the selection.enter()
selects the data that doesn't have corresponding elements in the DOM.append("p")
creates a new paragraph for each datum.text(d => ...)
sets the paragraph's text based on the datum.
Using Keys for Data Matching
You can use keys to match data and DOM elements. This is useful when working with data that has unique identifiers.
javascript
Updating and Removing Elements
D3 also provides methods to handle data and element updates and deletions:
- Update: To update existing elements when the data set changes.
- Exit: To handle elements that no longer have corresponding data and remove them.
javascript
Binding Data to Graphic Elements
Example: Bars in a Chart
Let's use data binding to create a simple bar chart.
javascript
In this example:
data(data)
binds thedata
set to the rectangles (rect
).enter()
creates a rectangle for each datum that doesn't have a corresponding element.attr
sets the position and size attributes of each rectangle based on the data.
Summary
Data binding in D3 is a powerful feature that allows you to dynamically create, update, and remove DOM elements based on your data. This capability is essential for building interactive and dynamic data visualizations.
In this chapter, we saw how to bind data sets to DOM elements, how to use keys for precise matching, and how to handle the creation, updating, and removal of elements based on data. In the upcoming chapters, we will explore how to use these techniques to create more advanced charts and customize their appearance and behavior.
- Introduction to D3
- Installation and Configuration of D3
- Selection and Manipulation of Elements in D3
- Binding Data to Elements in D3
- Scales and Axes in D3
- Creation of Bar Charts
- Line Chart Creation
- Creating Area Charts
- Creating Scatter Plots
- Hierarchical Data Visualization with Tree Graphs
- Creating Pie and Donut Charts
- Animations and Transitions in D3
- Interactivity in Graphics with D3
- Integration of D3 with Other Libraries and Frameworks
- Conclusion and Next Steps
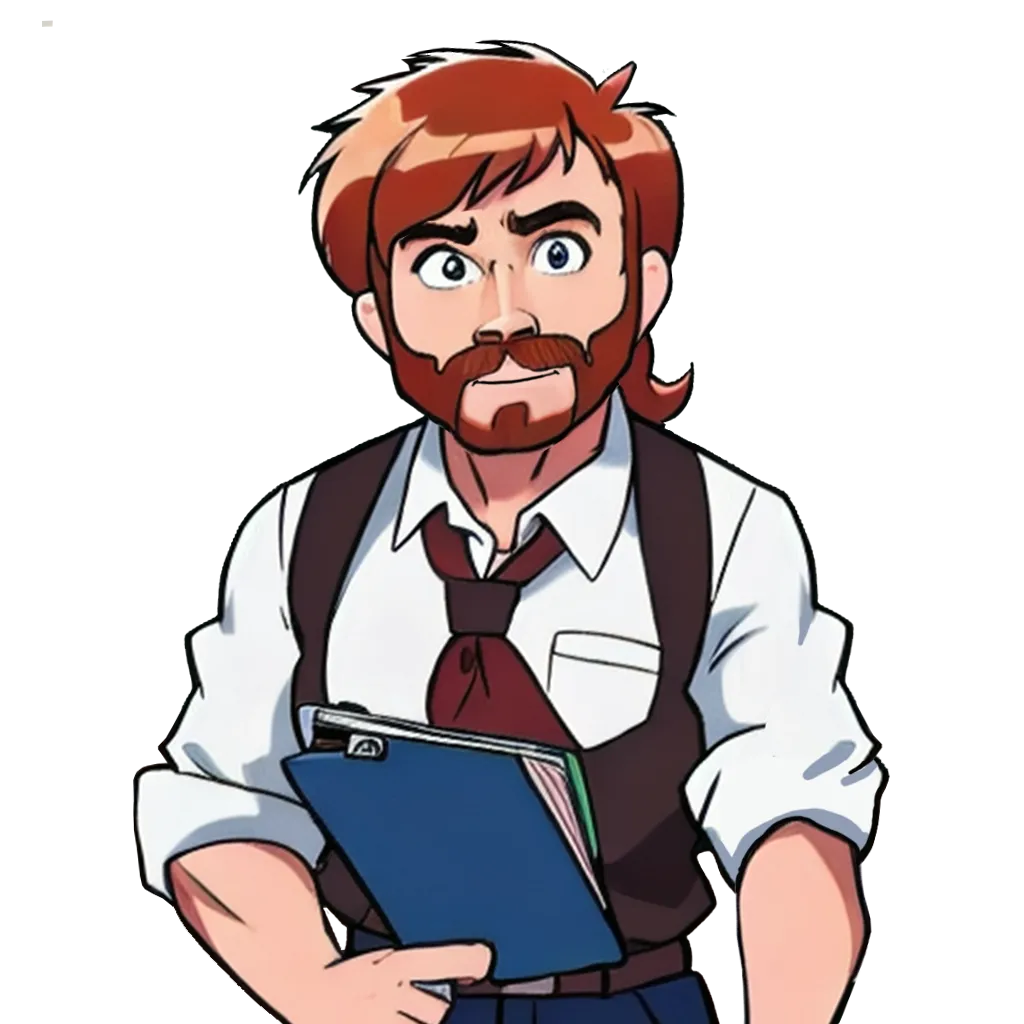