GIT
Advanced Git Commands
In this chapter, we will explore some of the advanced Git commands that allow you to handle more complex situations in your projects. We will cover the use of git cherry-pick
to select specific commits, git bisect
to find errors, and discuss how to use submodules and subtrees in Git to manage repositories within other repositories.
Cherry-Pick: Select Specific Commits
The git cherry-pick
command allows you to apply a specific commit from one branch to another. This is useful if you want to integrate a change into a branch without merging all commits.
Suppose you have made an important commit in a branch called feature-X
and you want to apply it to main
without doing a full merge. First, get the commit identifier:
bash
Then, use git cherry-pick
to apply that commit to main
:
bash
If any conflict arises during the cherry-pick, Git will prompt you to resolve it before continuing.
Bisect: Find Errors in the Code
The git bisect
command helps you find the cause of an error efficiently by performing a binary search in the commit history. If you know that an error appeared at some point between two commits, you can use git bisect
to narrow down the search and find the problematic commit.
To start the bisect process, first tell Git which commit worked correctly:
bash
Git will begin selecting commits between those two points. After each selection, you need to tell Git if the current commit is good or bad using the commands git bisect good
or git bisect bad
. Git will continue narrowing down the search until you identify the commit that introduced the error.
To end the search, use:
bash
Submodules in Git
Submodules allow you to include a repository within another. This is useful when you want to maintain independent projects but need to integrate them into a main repository. For example, you could have a shared library that is used in several projects, and you want to keep it as a submodule instead of duplicating it.
To add a submodule to your repository, use the following command:
bash
To initialize and update the submodules after cloning a repository, use:
bash
Subtrees: Alternative to Submodules
Subtrees are another way to include a repository within another. Unlike submodules, subtrees do not require additional steps to initialize or update, as the content of the included repository is stored directly in the main repository.
To add a subtree, use:
bash
To update the subtree later:
bash
Conclusion
In this chapter, we have explored advanced Git commands that allow you to handle complex situations in your projects. git cherry-pick
allows you to select specific commits, git bisect
helps you find errors efficiently, and submodules and subtrees allow you to include repositories within others in an organized manner. In the next chapter, we will see how to work with hooks in Git to automate processes and improve productivity in your workflow.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
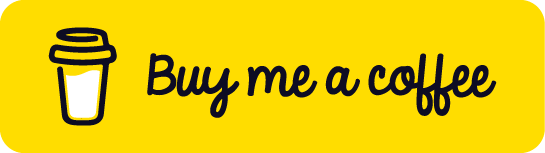
Chat with Chuck
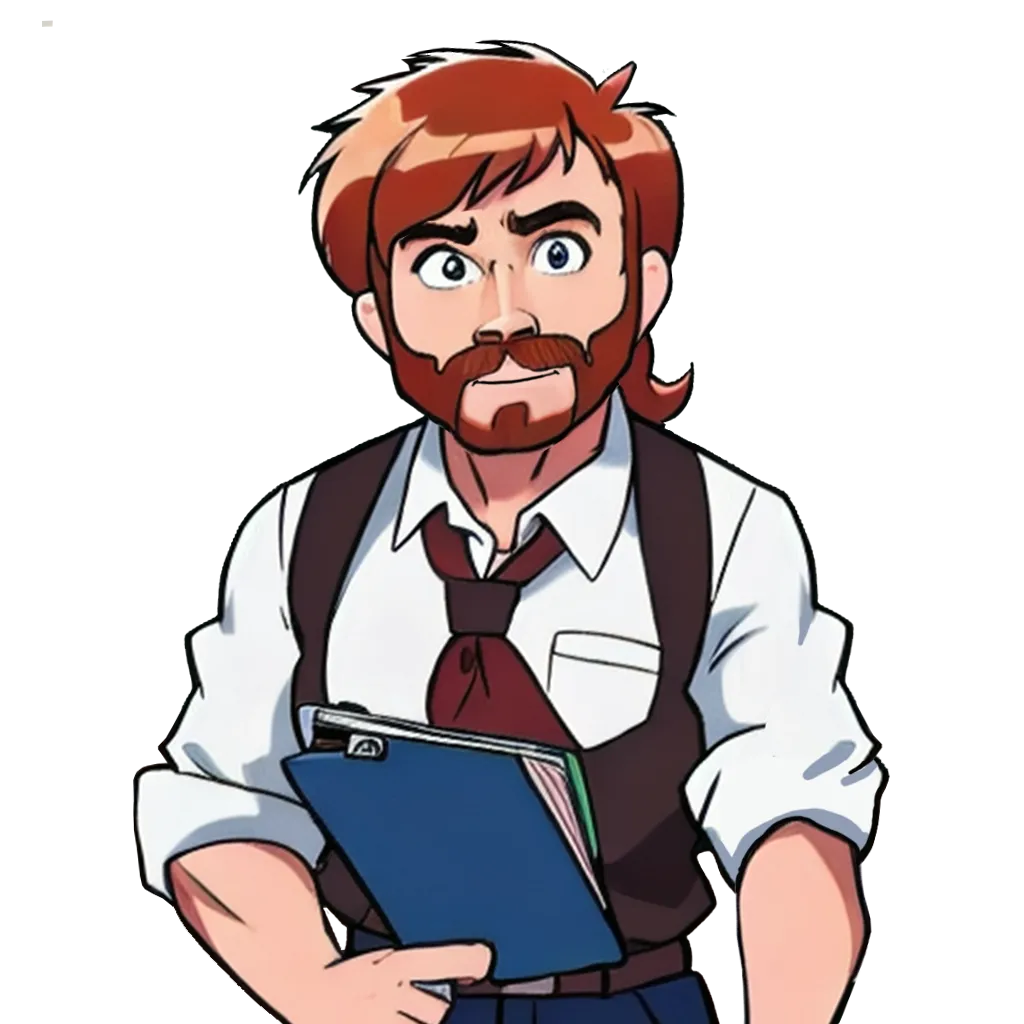
- Introduction to Git and Version Control
- Installation of Git and Initial Setup
- Understanding Repositories
- Basic Workflow in Git
- Working with Branches in Git
- Collaborating with Other Developers
- Undoing Changes in Git
- Working with Tags in Git
- Rebase and Squash in Git
- Stashing and Cleaning in Git
- Advanced Git Commands
- Hooks and Automation in Git
- GitHub and Repository Management
- Best Practices in Git
- Conclusion and Final Tips