What's New in HTML5
Web Workers API
The Web Workers API in HTML5 allows scripts to run in the background in parallel with the browser's main execution thread. This means you can perform computationally intensive tasks without blocking the user interface, significantly improving performance and the user experience in web applications.
What are Web Workers?
Web Workers are background scripts that the browser runs separately from the main thread of the page. This allows heavy operations to not block the user interface thread, keeping the page responsive to the user.
Types of Web Workers
- Dedicated Workers: These are specific to a single page and cannot be shared between multiple scripts.
- Shared Workers: These can be accessed by multiple scripts and pages, allowing communication between different windows and tabs of the same application.
Creating a Dedicated Worker
To create and use a Dedicated Worker
, you first need a JavaScript file that will act as the "worker". Here’s how to do it:
Worker Code (worker.js)
javascript
Main Script Code (index.html)
html
[Placeholder Image: Diagram showing communication between the main script and a Web Worker, indicating message sending and receiving]
Communicating with Web Workers
Communication between the main script and Web Workers is achieved through the postMessage()
method and the onmessage
event.
Sending Messages to the Worker
Use the postMessage()
method to send data to the Worker:
javascript
Receiving Messages from the Worker
Capture messages from the Worker by handling the onmessage
event:
javascript
Using Shared Workers
Shared Workers
allow multiple scripts and windows to access them simultaneously. Here’s how to use them:
Shared Worker Code (sharedWorker.js)
javascript
Main Script Code (index.html)
html
[Placeholder Image: Diagram showing communication between multiple windows and a Shared Worker]
Security Considerations
- Limited Scope: Web Workers cannot directly manipulate the DOM. This ensures that heavy operations do not affect the user interface.
- Cross-Origin Policies: Web Workers adhere to the same cross-origin policies that apply to the
XMLHttpRequest
object. - Data Security: Ensure that you validate and sanitize data transferred between the main script and Web Workers.
Practical Applications of Web Workers
- Data Processing: Process large volumes of data without blocking the user interface.
- Complex Calculations: Execute complex mathematical calculations or simulations.
- File Handling: Manipulate large data files in the background.
Conclusion
The Web Workers API provides an efficient way to perform background tasks without affecting user interface performance. By using Dedicated Workers and Shared Workers, you can create faster and more responsive web applications, thereby improving the user experience.
- Introduction to HTML5
- Estructura básica de un documento HTML5
- New Semantic Elements
- Advanced Forms in HTML5
- Multimedia in HTML5: audio and video
- Graphics and Animations with Canvas and SVG
- Web Storage: localStorage and sessionStorage
- Geolocation API
- WebSockets API
- Web Workers API
- Drag and Drop in HTML5
- Accessibility Improvements with HTML5
- HTML5 and SEO
- HTML5 and CSS3: integrations and new possibilities
- Conclusion and Future Trends of HTML5
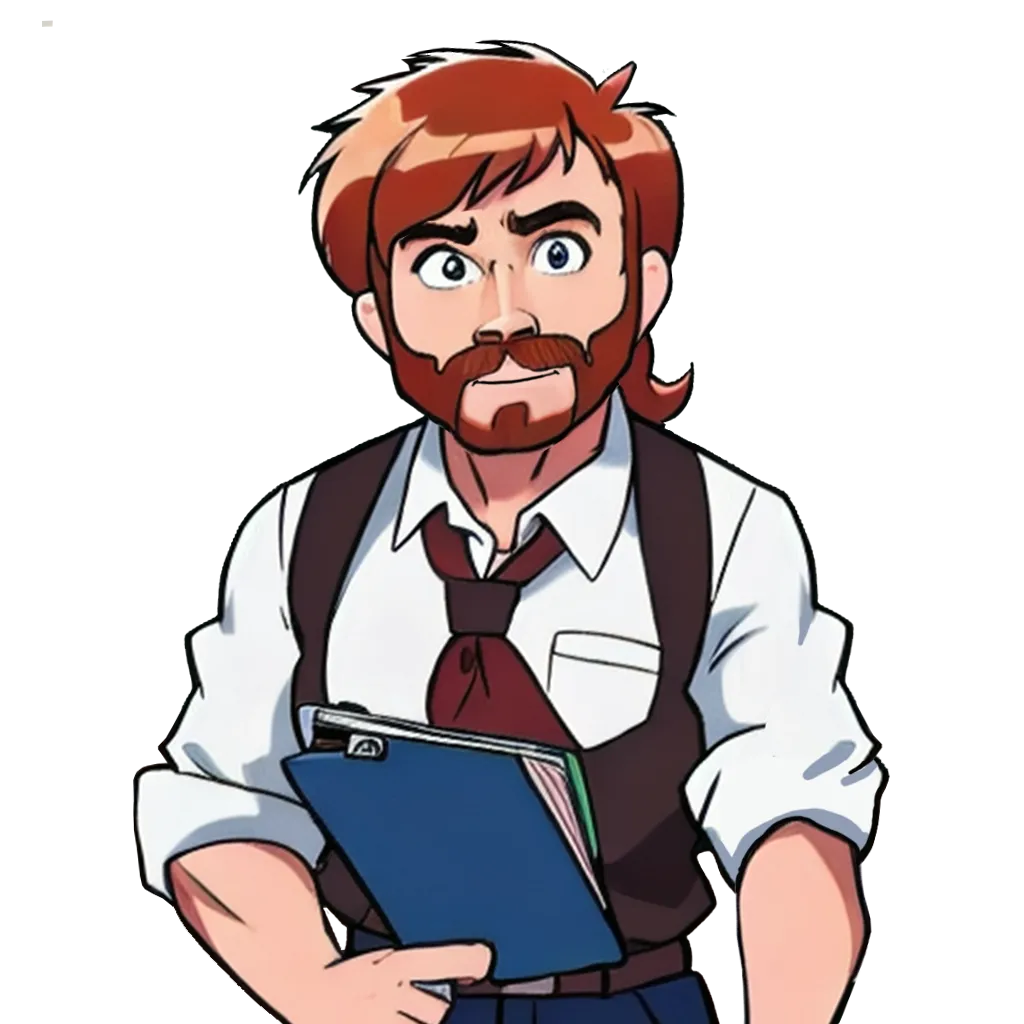