Working with Images in Node
Basics of File Handling in Node.js
File handling is an essential skill in any programming language, and in Node.js, we have a robust set of modules that allow us to perform file operations efficiently. In this chapter, we will explore the basics of file handling in Node.js, including how to read, write, and manipulate files in the file system.
The fs
Module
Node.js provides the fs
(file system) module to interact with the file system. This module includes both synchronous and asynchronous methods for handling files, although it is recommended to use the asynchronous versions to avoid blocking the event loop.
Reading Files
Let's start with a basic example of how to read a file using the fs
module:
Synchronous Reading
Synchronous reading is straightforward, but remember that it can block the event loop, which is not ideal for production applications:
javascript
Asynchronous Reading
The recommended version is asynchronous reading, which will not block the event loop and will allow other operations to continue executing:
javascript
Writing Files
Similar to reading files, we can write files both synchronously and asynchronously.
Synchronous Writing
javascript
Asynchronous Writing
javascript
File Manipulation
In addition to reading and writing files, the fs
module provides methods for manipulating files such as renaming, deleting, and copying files.
Renaming Files
javascript
Deleting Files
javascript
Practical Example: Handling Image Files
To connect these fundamentals with working with images, let's give a practical example of how to read and write images using the fs
module.
javascript
[Here you could add an image showing a directory structure with text files and images, highlighting the operations of reading, writing, renaming, and deleting]
With these fundamentals, you will be prepared to perform file handling operations in Node.js, which is essential for the upcoming chapters where we will focus on the specific manipulation of image files.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
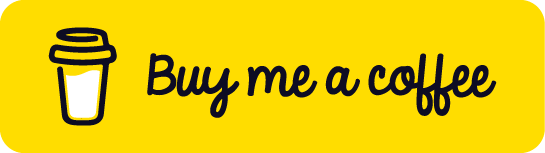
Chat with Chuck
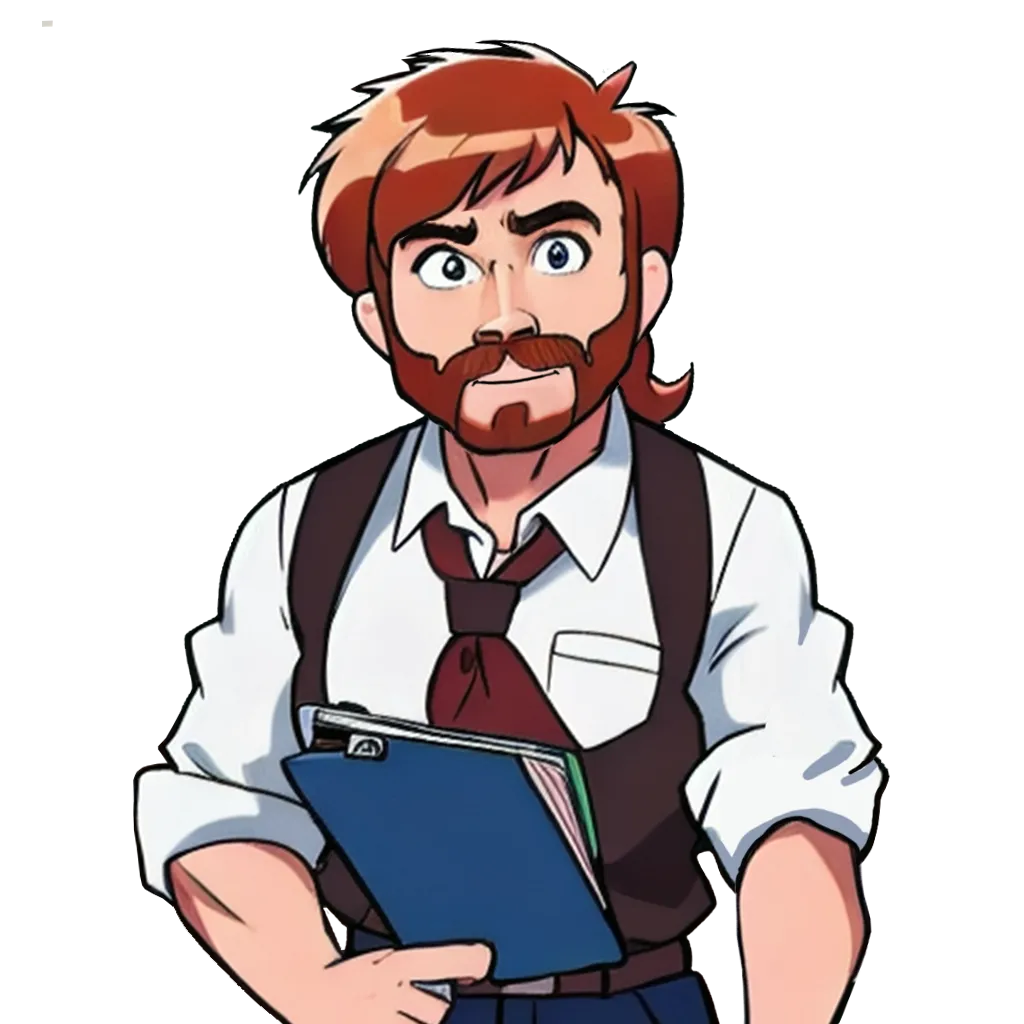
- Introducción al trabajo con imágenes en Node.js
- Setting Up the Development Environment
- Basics of File Handling in Node.js
- Installation and Use of Image Manipulation Modules
- Reading and Writing Images
- Image Format Conversion
- Image Resizing and Cropping
- Applying Filters and Effects
- Working with Images in Different Resolutions
- Image Compression and Optimization
- Automatic Thumbnail Creation
- Generation of Graphs and Visualizations
- Using Images in Web Applications with Node.js
- Cloud Storage Services Integration
- Conclusion and Best Practices