HTML5 Drag & Drop API
Handling Drag Events
In the previous chapters, we enabled draggable elements and set up the dragstart
event to initiate the interaction. Now we will explore the events that form the complete cycle of the drag and drop process, including drag
, dragover
, drop
, and dragend
. These events allow us to manage each stage of the interaction precisely.
The Drag and Drop Event Cycle
The Drag and Drop API cycle consists of several key events:
- dragstart: Occurs when an element starts to be dragged. Here, data transfer is initialized.
- drag: Activated while the element is being dragged.
- dragover: Occurs while the dragged element is over a valid drop zone.
- drop: Triggered when the element is dropped in a valid zone.
- dragend: Marks the end of the drag process, whether the element is dropped or not.
Handling the drag
Event
The drag
event runs continuously while the user drags an element. This event can be useful for updating information in real-time.
Example of Using the drag
Event
javascript
Allowing Drop: The dragover
Event
For an element to be dropped in a drop zone, it is necessary to handle the dragover
event and call the event.preventDefault()
method. This indicates that the zone is ready to receive the element.
Setting Up the dragover
Event
javascript
Dropping an Element: The drop
Event
The drop
event is triggered when an element is dropped in a valid zone. This is the moment we can retrieve the transferred data and process the action.
Retrieving Data with drop
javascript
Ending the Cycle: The dragend
Event
The dragend
event is triggered at the end of the drag operation, regardless of whether the element was dropped or not. It is useful for cleaning up styles or temporary resources.
Using the dragend
Event
javascript
Practical Exercise
Implement a page where:
- Elements are dragged and log their position in real-time.
- A drop zone validates the
dragover
event and displays a message when the element is dropped. - The
dragend
event cleans up any temporary styles applied to the element.
Conclusion
In this chapter, we explored the main events of the Drag and Drop API and how to handle them to create dynamic interactions. Now you can manage the entire lifecycle of a dragged element, from start to finish.
In the next chapter, we will learn how to define specific drop zones and validate them to accept only certain types of elements. Join us as we continue to build more advanced functionalities!
- Introduction to the Drag and Drop API
- Making Draggable Elements
- Handling Drag Events
- Defining Drop Zones
- Styling Drag and Drop Interactions
- Advanced Drag and Drop Techniques
- Practical Example: Building a Drag and Drop Application
- Integrating Drag and Drop with Other APIs
- Best Practices and Accessibility
- Conclusion and Next Steps
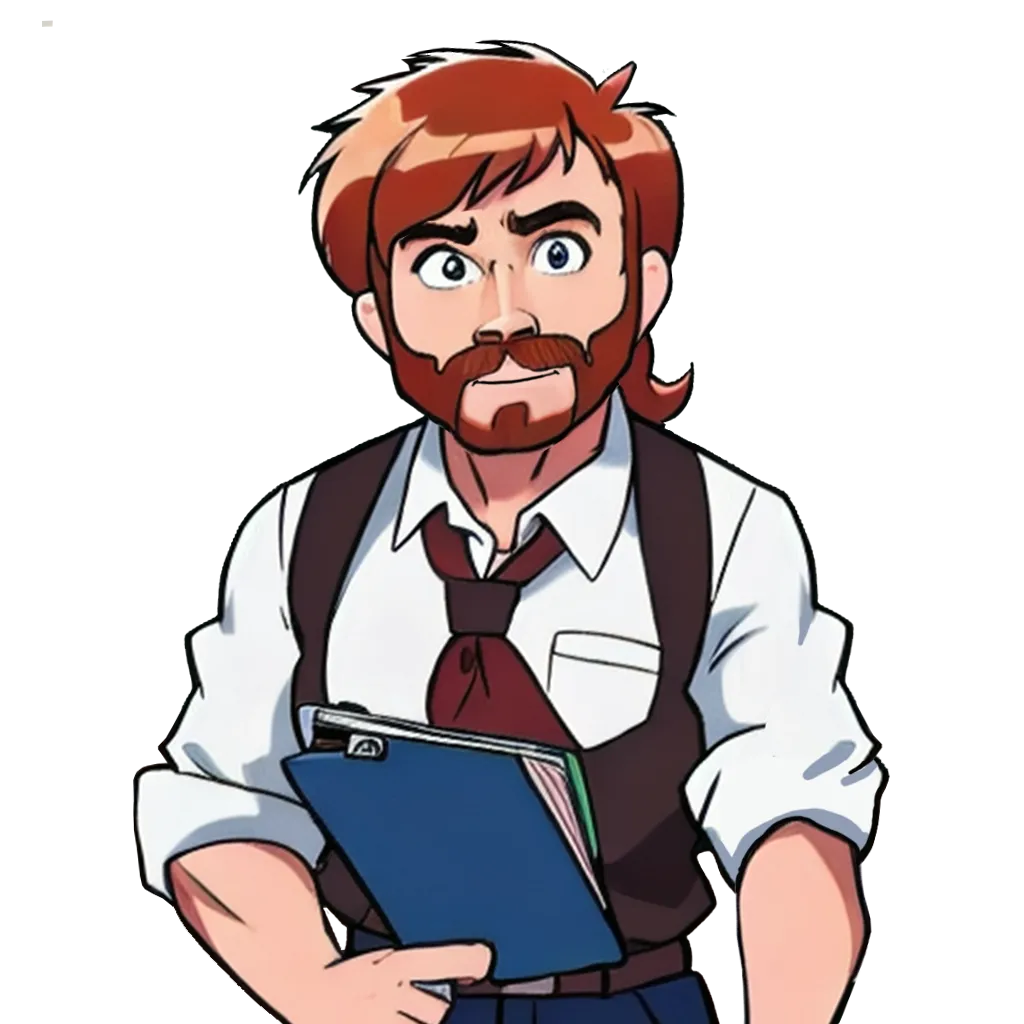