HTML5 Drag & Drop API
Making Draggable Elements
In the previous chapter, we learned the basics of the Drag and Drop API and set up an initial environment. Now we will dive deeper into how to enable draggable elements in HTML using the draggable
attribute and manage the dragstart
event to initiate interaction.
Enabling a Draggable Element
Any HTML element can be made draggable by adding the attribute draggable="true"
. This attribute indicates that the element can be moved within the page.
Example of a Draggable Element
html
It is important to note that while some elements like images and links are draggable by default, other elements such as div
or span
explicitly require the draggable="true"
attribute.
Introduction to the dragstart
Event
The dragstart
event is the first step in a drag-and-drop operation. This event is triggered when the user starts dragging an element, and it is the ideal time to prepare data transfer.
Code to Manage the dragstart
Event
javascript
Explanation of the DataTransfer
Object
The DataTransfer
object is fundamental in the Drag and Drop API. It allows storing data that can be retrieved later during the interaction.
setData
Method: Stores data in a specific format liketext/plain
orapplication/json
.getData
Method: Retrieves stored data.
javascript
Enhancing User Experience
The user experience can be improved by applying dynamic styles to the element while it is being dragged.
Change the Style During Dragging
javascript
Additional Validations
It is possible to add validations to ensure that only certain elements are draggable.
Validate Draggable Elements
javascript
Practical Exercise
Create a page with multiple elements, some enabled to be dragged and others not. Use the draggable
attribute and add validations so only selected elements can initiate the dragstart
event.
Conclusion
In this chapter, we learned how to enable draggable elements with the draggable
attribute and manage the dragstart
event to initiate interaction. We also explored how to use the DataTransfer
object to store data and how to enhance user experience with dynamic styles.
In the next chapter, we will explore the additional events that occur during the drag-and-drop cycle, such as drag
, dragover
, and drop
. Join us to continue building this functionality!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
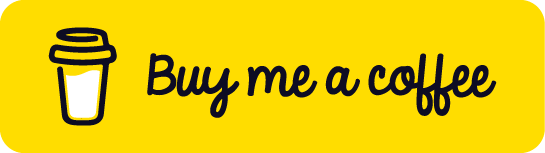
Chat with Chuck
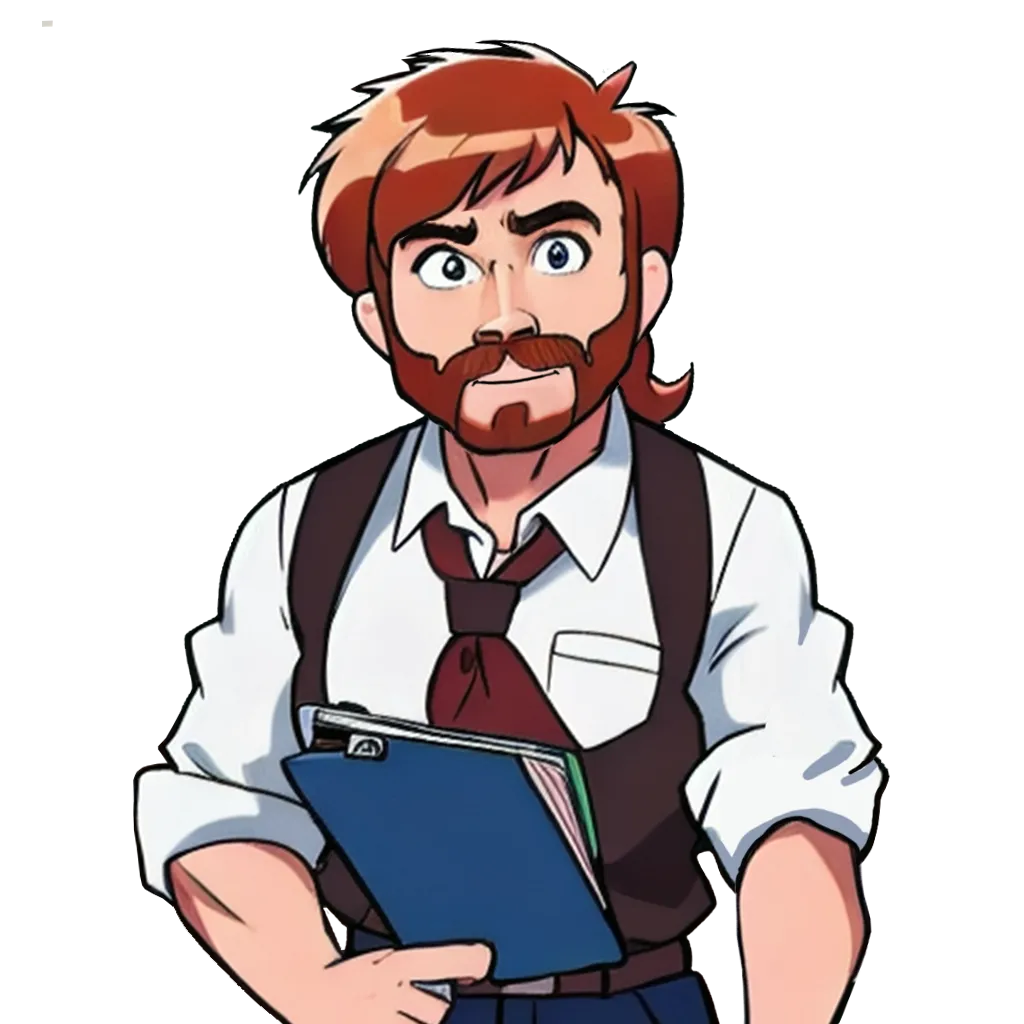
- Introduction to the Drag and Drop API
- Making Draggable Elements
- Handling Drag Events
- Defining Drop Zones
- Styling Drag and Drop Interactions
- Advanced Drag and Drop Techniques
- Practical Example: Building a Drag and Drop Application
- Integrating Drag and Drop with Other APIs
- Best Practices and Accessibility
- Conclusion and Next Steps