WebSockets with Node
Authentication and Security
Authentication and Security
Authentication and security are crucial components for any web application. In this chapter, we will address how to implement authentication and security measures on your WebSocket server using Node.js.
Importance of Security in WebSockets
WebSockets, like other network communication technologies, are susceptible to various vulnerabilities such as data interceptions and injection attacks. Securing our application is vital to protect it against these threats.
Image Placeholder: [Illustration showing potential security threats in WebSocket connections.]
Authentication via Tokens
A common way to authenticate WebSocket connections is through tokens, such as JSON Web Tokens (JWT). Tokens can be validated when a client attempts to establish a connection.
Installing Required Libraries
First, we install the necessary libraries to handle JWT.
bash
Configuring the Server to Validate Tokens
Let's modify our WebSocket server to validate JWT during the connection:
javascript
In this code, we verify the JWT token provided in the WebSocket connection URL. If the token is valid, we allow the connection; otherwise, we reject it.
Token Generation
To test this configuration, we need to generate a token:
javascript
Use this token in the client's WebSocket connection URL:
Image Placeholder: [Screenshot of the console showing a generated token and an accepted/rejected connection based on the token.]
Data Encryption with WSS (WebSocket Secure)
To secure data transmission, it is recommended to use WebSocket over TLS (WSS).
WSS Configuration
First, we need to obtain TLS certificates. For development, we can use openssl
to generate self-signed certificates.
bash
Updating the Server to Use WSS
We modify our server to use https
and ws
over TLS:
javascript
WebSocket Client Using WSS
Ensure that your WebSocket client connects correctly using wss
:
html
Additional Protections
Ping/Pong Activity Detection
Keep connections active by periodically validating communication with pings and pongs:
javascript
Limiting Message Size
Protect the server from large message attacks by limiting the maximum size of incoming messages:
javascript
Image Placeholder: [Diagram showing security mechanisms in action, such as JWT authentication, pings/pongs, and WSS encryption.]
With these concepts and techniques, you can ensure that WebSocket connections in your Node.js application are secure and protected against common vulnerabilities. Let's keep improving our application in the next chapters! 🚀
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
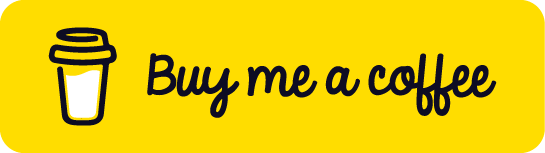
Chat with Chuck
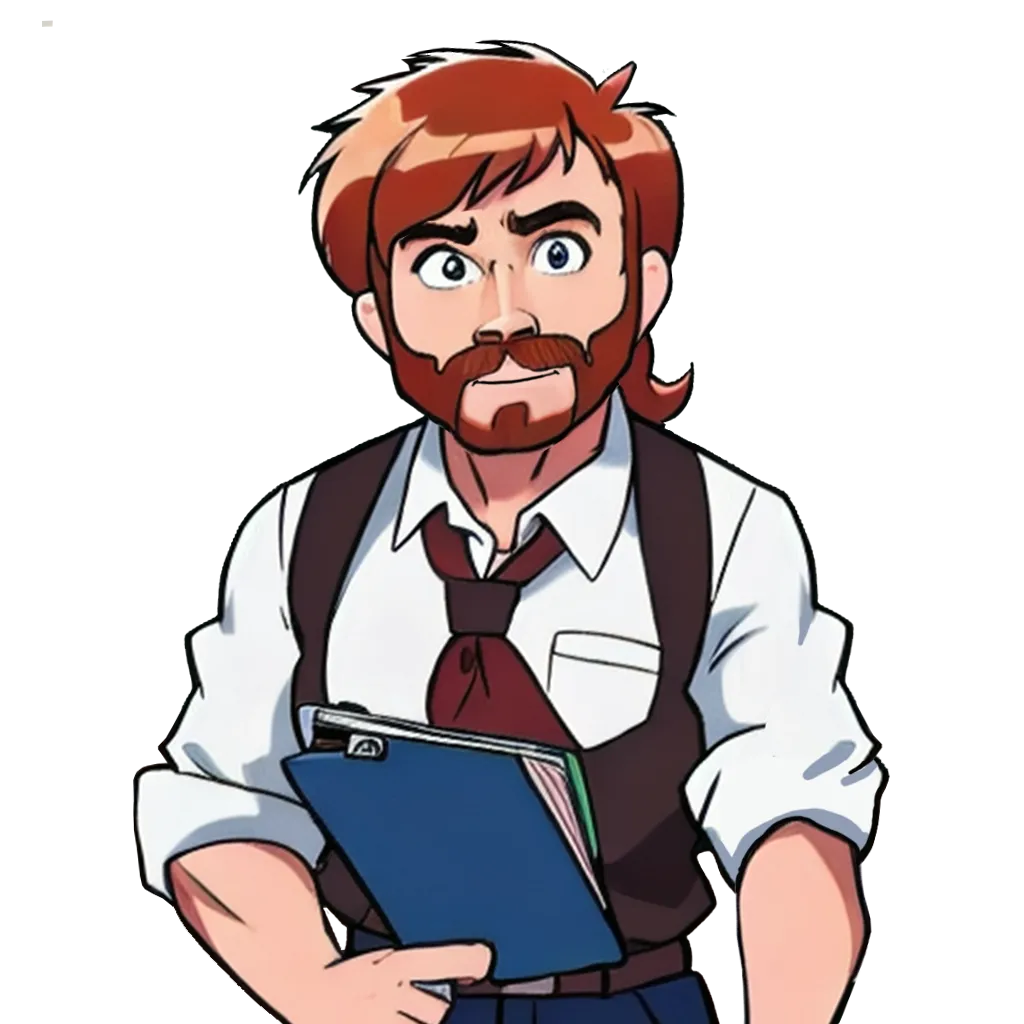