WebSockets with Node
Event Handling and Messaging
Event Handling and Messaging
So far, we have set up a basic WebSocket server and tested the connections between the server and the client. In this chapter, we will delve deeper into how to handle various events and messages to build a more robust and functional application.
WebSocket Events
There are multiple events you can use to manage WebSocket connections. Here are some of the most common ones:
connection
: Triggered when a client connects to the server.message
: Triggered when a message is received from a client.close
: Triggered when a connection is closed.error
: Triggered when an error occurs in the connection.
We will adjust our server to handle these events appropriately.
Handling the Connection Event
The connection
event is handled as we did in the previous chapter. We can add additional features, such as tracking active connections:
javascript
Here, we maintain a Set
of connected clients and update this set when a client connects or disconnects.
Handling the Message Event
We handle incoming communications through the message
event. For example, we can broadcast messages to all connected clients:
javascript
In this code, we broadcast received messages to all clients except the sender. This is useful for applications like real-time chats.
Handling the Close Event
Client disconnection management was partially covered earlier. Here it is more elaborated:
javascript
The disconnected client is removed from the Set
of active clients and the disconnection is logged.
Handling the Error Event
To handle errors in connections:
javascript
With this, we can log errors that occur during the WebSocket connection.
Business Logic
We can manage the business logic that we need to apply to these events according to the use case of our application. For example:
Message Broadcasting
We can adjust our message handling to include a broadcasting mechanism:
javascript
Placeholder Image: [Flowchart showing the process of handling events and broadcasting messages to multiple clients.]
Targeted Messaging
We can send messages to specific clients using unique identifiers. For example:
javascript
Pings and Pongs
We can use pings and pongs to check the health of the connections:
javascript
With all this, we are able to effectively manage events and messages to build robust applications with WebSockets and Node.js. Let's keep exploring more features in the next chapter! 🚀
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
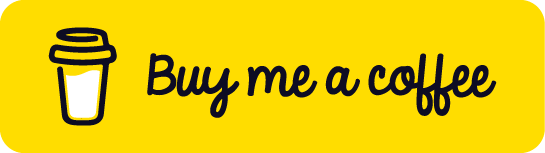
Chat with Chuck
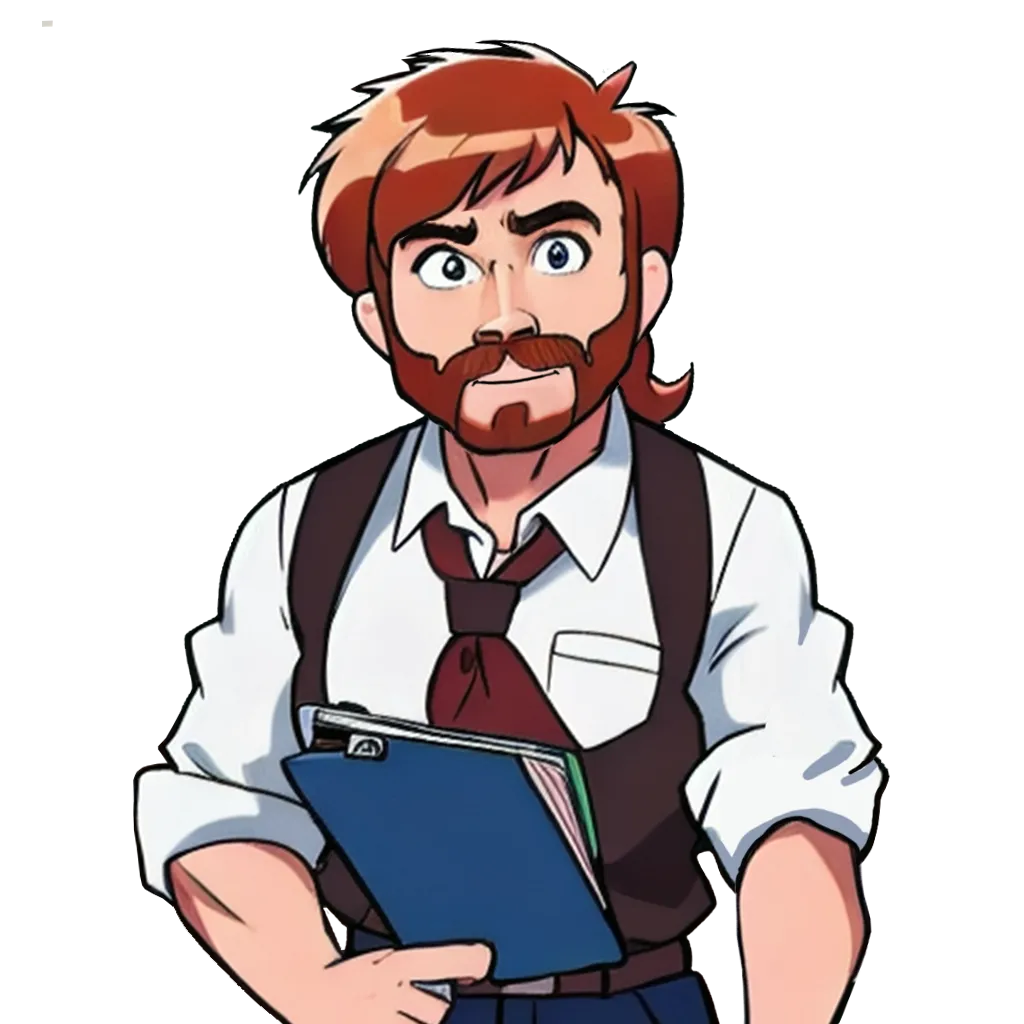