WebSockets with Node
Creating a WebSocket Server using Node.js
Creating a WebSocket Server using Node.js
Now that we have our environment set up, it is time to dive into how to create a WebSocket server using Node.js. In this chapter, we will create a basic WebSocket server and understand how to manage connections and messages.
Basic WebSocket Server Setup
In the server.js
file we created earlier, we have the basic structure of a WebSocket server. Let's go over that code line by line to understand it thoroughly.
Importing the ws
Library
javascript
First, we import the ws
library, which provides the necessary tools to work with WebSockets.
Creating an Instance of the WebSocket Server
javascript
We create a new instance of WebSocket Server and configure the server to listen on port 8080.
Handling New Connections
javascript
The on
method allows us to set up an event handler for incoming connections. Each time a client connects, this block of code will be executed, where ws
represents the connected client's WebSocket.
Listening and Sending Messages
Within the connection block, we set up two events:
-
Listening for Client Messages:
javascriptHere, we set up a
message
event on the client's WebSocket to listen to any message the client sends. Then, we simply log the message to the server console. -
Sending Messages to the Client:
javascriptWe use the
send
method to send a message back to the client when the connection is established.
Starting the Server
Finally, we start the WebSocket server with the command
bash
The server will start running and be ready to receive connections at ws://localhost:8080
.
Image Placeholder: [Diagram illustrating the connection and communication flow between the client and WebSocket server.]
Testing the WebSocket Server
To test our WebSocket server, we can use a simple WebSocket client tool like wscat
or a client implemented in JavaScript in the browser.
Using wscat
-
Install
wscat
: Run the following command to installwscat
globally on your machine.bash -
Connecting to the WebSocket Server: Once installed, use the following command to connect to your WebSocket server at
ws://localhost:8080
.bashYou should see the message 'Hello! Message From Server!!', indicating that you have successfully connected. You can send messages from
wscat
which will be logged in your server terminal.
Using a Browser
You can also use the following code to connect to your server from the browser:
html
Save this code as index.html
and open it in your browser. You should see messages in the browser console and in the server terminal.
Image Placeholder: [Screenshot of the browser console showing messages sent and received from the WebSocket server.]
With this, we have created and tested our first WebSocket server using Node.js. In the upcoming chapters, we will explore how to handle events and messages in a more advanced manner. Let's keep moving forward! 🚀
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
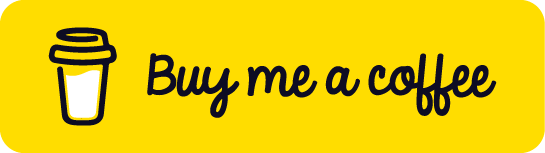
Chat with Chuck
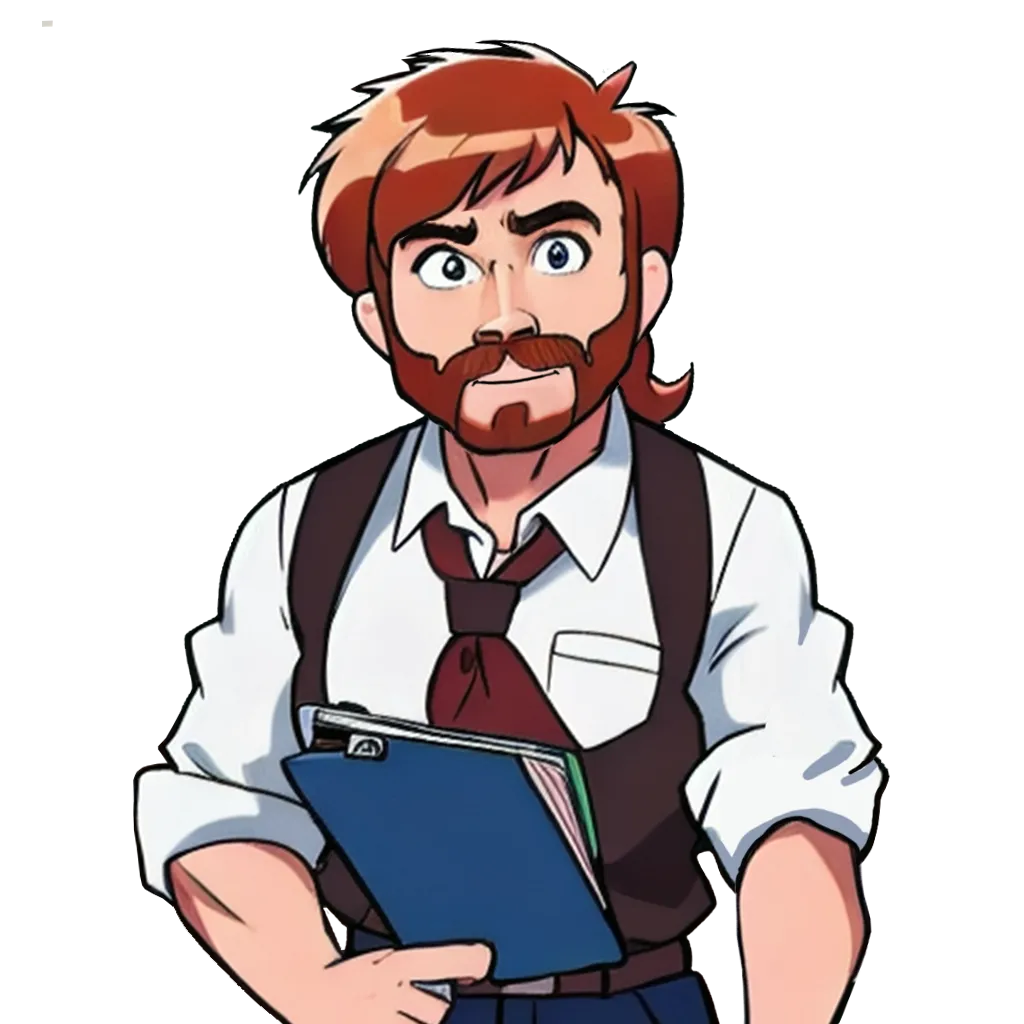