Performance in React
Component Rendering Optimization
Component Rendering Optimization
One of the first steps to improve the performance of a React application is to optimize how and when components are rendered. Here we will look at some techniques to achieve that.
Conditional Re-rendering
Conditional re-rendering ensures that a component only renders if there are significant changes in the data that affect it. For this, you can use the shouldComponentUpdate
method in class components.
javascript
In functional components, you can use React.memo
to memoize the component and avoid unnecessary renderings.
javascript
Using PureComponent
PureComponent
is a version of a class component that implements shouldComponentUpdate
with a shallow comparison of props and state.
javascript
Fragments to Avoid Unnecessary Elements
By using React.Fragment
, you can avoid creating additional DOM nodes, which can improve performance.
javascript
Avoid Unnecessary State Updates
Make sure not to update the state of a component if it is not necessary. React will re-render the component when the state changes, so you should perform updates only when needed.
javascript
In this example, the increment
function uses the previous state to update the count, avoiding unnecessary re-renderings.
Conclusion
By applying these techniques, you can ensure that your components only re-render when absolutely necessary, thereby improving the overall performance of your application. In the next topic, we will explore how memoization can benefit component performance in React.
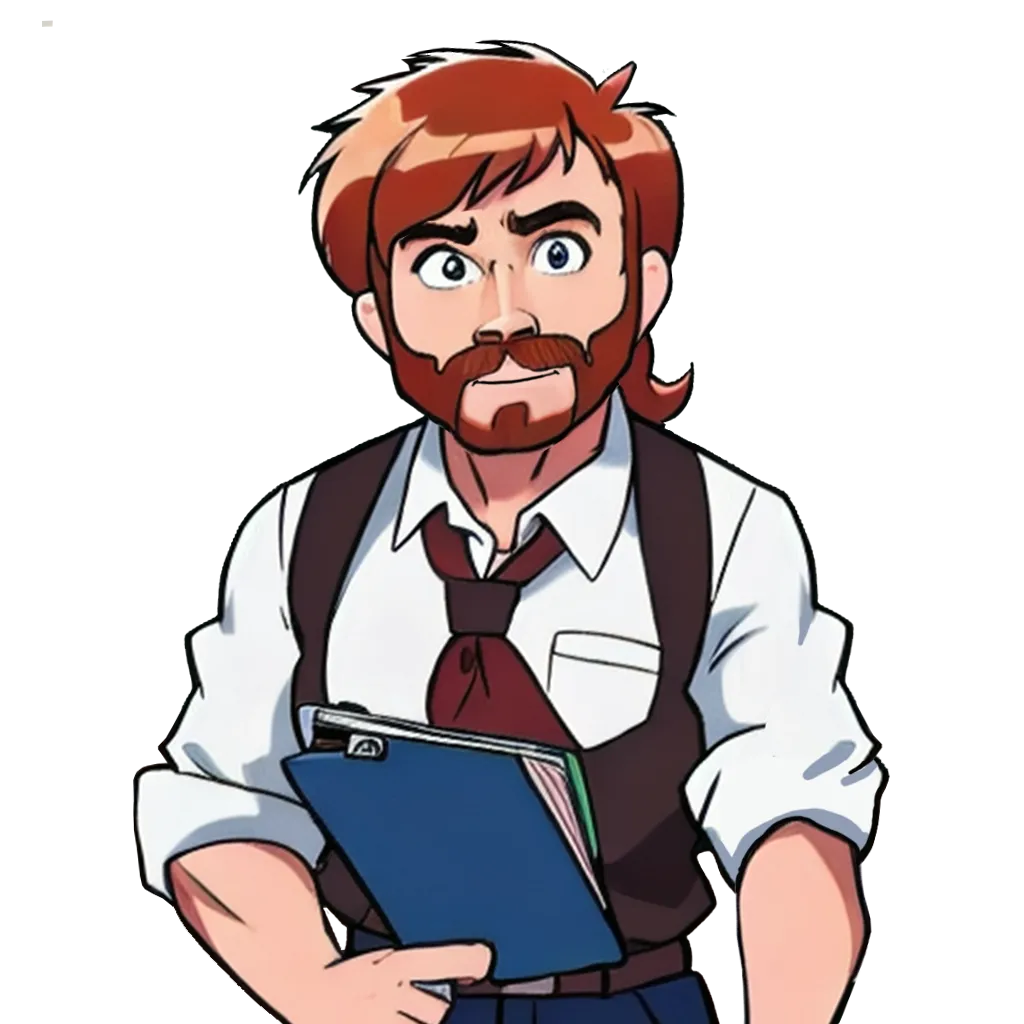