Performance in React
Using Memoization
Using Memoization
Memoization is an optimization technique that stores the results of expensive function calls and returns the cached result when the same inputs occur again. In React, memoization can help avoid unnecessary re-renders and calculations.
React.memo
React.memo
is a higher-order component that you can use to memoize a component. This means that the component will only re-render if its props change.
Basic Example
javascript
In this example, ExpensiveComponent
only renders if its value
prop changes, thanks to React.memo
.
useMemo
useMemo
is a hook that memoizes the value of a function. It is useful for memoizing derived values that are expensive to compute.
Basic Example
javascript
In this example, expensiveCalculation
only recalculates when count
changes, thanks to useMemo
.
useCallback
useCallback
is a hook similar to useMemo
, but it's designed to memoize functions. It is useful for passing functions to child components without creating new instances of the function on every render.
Basic Example
javascript
In this example, handleClick
is only recreated if count
changes, thanks to useCallback
.
Conclusion
Memoization is a powerful technique to optimize components and calculations in React. By using React.memo
, useMemo
, and useCallback
, you can improve your application's performance by avoiding unnecessary re-renders and calculations. In the next topic, we will explore how to optimize resource loading in React applications.
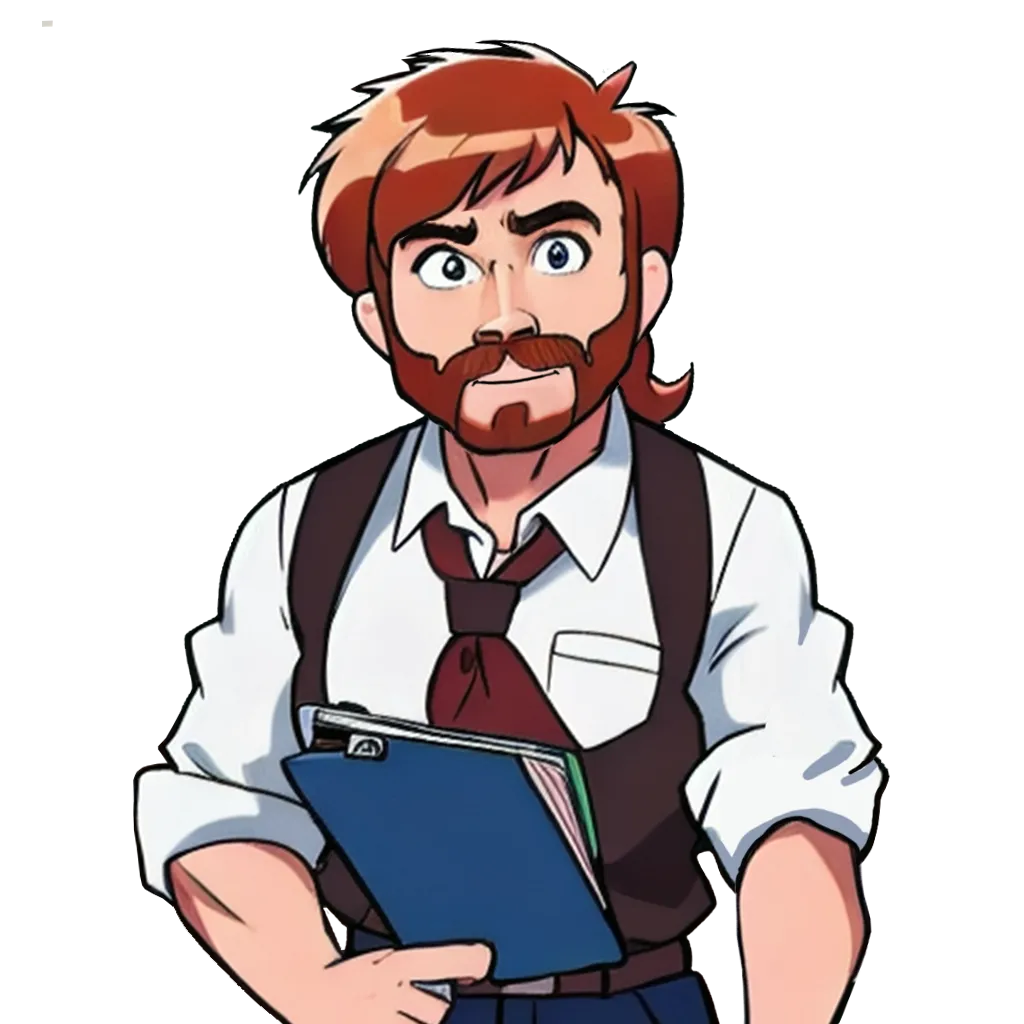