Performance in React
Resource Loading Optimization
Resource Loading Optimization
Optimizing resource loading is crucial for improving the performance of your React application. These resources can include images, scripts, CSS files, and more. Below, we will discuss various strategies to optimize how and when these resources are loaded.
Defer Loading
Defer loading allows resources to be loaded once the essential content of the page has been loaded and rendered.
Example
For scripts, you can use the defer
attribute.
html
Conditional Loading
Conditional loading is useful for loading resources only when they are actually needed. This can be done using components that are only imported when required.
Example with React
You can use React.lazy and Suspense to load components conditionally.
javascript
Image Compression
Images are generally the heaviest resources on a web page. Compressing the images can significantly reduce the load time.
Tools and Techniques
- Online Tools: such as TinyPNG or JPEG-Optimizer
- Webpack Plugins: such as image-webpack-loader
javascript
Conditional CSS File Import
Another way to optimize resource loading is by conditionally importing CSS files as needed.
Example
javascript
Using Service Workers
Service Workers can be used to cache resources and serve them quickly without the need for a new network request.
Basic Example
javascript
mobile-image: Placeholder for an image showing how defer loading and conditional resource loading work.
Conclusion
Optimizing resource loading is an essential strategy for improving the performance of React applications. By implementing techniques like defer loading, conditional loading, image compression, and the use of Service Workers, you can significantly reduce load times and improve user experience. In the next topic, we'll explore Lazy Loading techniques in React applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
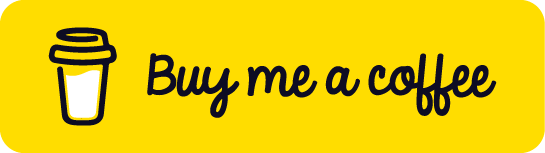
Chat with Chuck
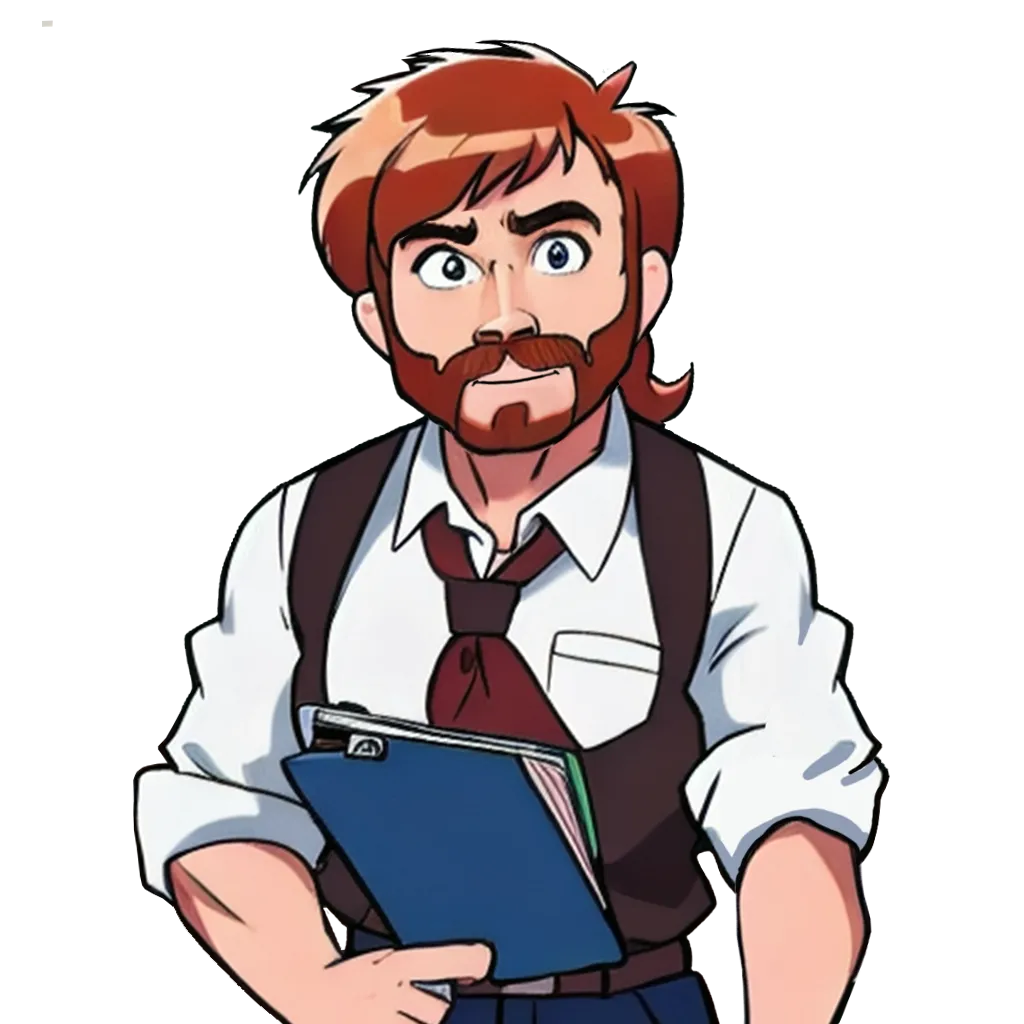