Accessibility in HTML
Accessibility in Custom Components
Custom components are a fundamental part of modern development, especially in dynamic applications using frameworks like React, Angular, or Vue. However, these components can be problematic from an accessibility perspective if not implemented correctly.
In this chapter, we will explore how to design and develop accessible custom components using best practices, semantic HTML, and ARIA.
Why can custom components be problematic?
Custom components, like those created with <div>
or <span>
, lack the semantics and native behaviors that assistive technologies need to interpret them correctly. For example:
- A button made with
<div>
is not automatically keyboard accessible. - A custom dropdown menu may lack logical navigation or defined roles.
Principles for accessible components
- Use native labels whenever possible: Prefer
<button>
,<input>
, and<select>
instead of reinventing their functionality. - Add ARIA roles when necessary: Use roles to describe the function of the custom component.
- Manage focus and navigation: Ensure the component is keyboard operable.
- Provide visual feedback: Use clear indicators for states like focus, selection, or activation.
Example: Custom button
A custom button must be keyboard operable (Enter
or Space
) and provide an adequate role.
Non-accessible code:
html
Accessible code:
html
Example: Accessible dropdown menu
Dropdown menus need roles and states indicating whether they are open or closed.
Accessible code:
html
Using frameworks for accessible components
Many frameworks offer libraries that help create accessible components, such as:
- React ARIA: Provides hooks for accessibility in React components.
- Angular Material: Includes accessible components by default.
- Vue A11y: Plugins to improve accessibility in Vue.
Example with React:
javascript
Testing and tools for custom components
Test with tools like:
- Axe DevTools: Analyzes the accessibility of your components in real-time.
- Storybook: Test components in isolation.
- Testing Library: Write unit tests to verify accessibility.
Testing example with Testing Library:
javascript
Conclusion
Creating accessible custom components requires a deep understanding of semantic HTML, ARIA, and user needs. By following best practices and conducting thorough testing, you can ensure that even the most advanced components are inclusive.
This content complements the course and reinforces the importance of accessibility throughout the development process. Keep creating inclusive digital experiences!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
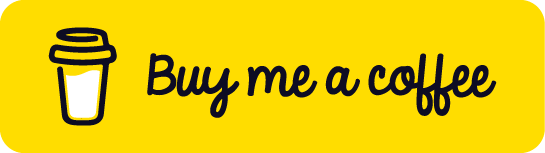
Chat with Chuck
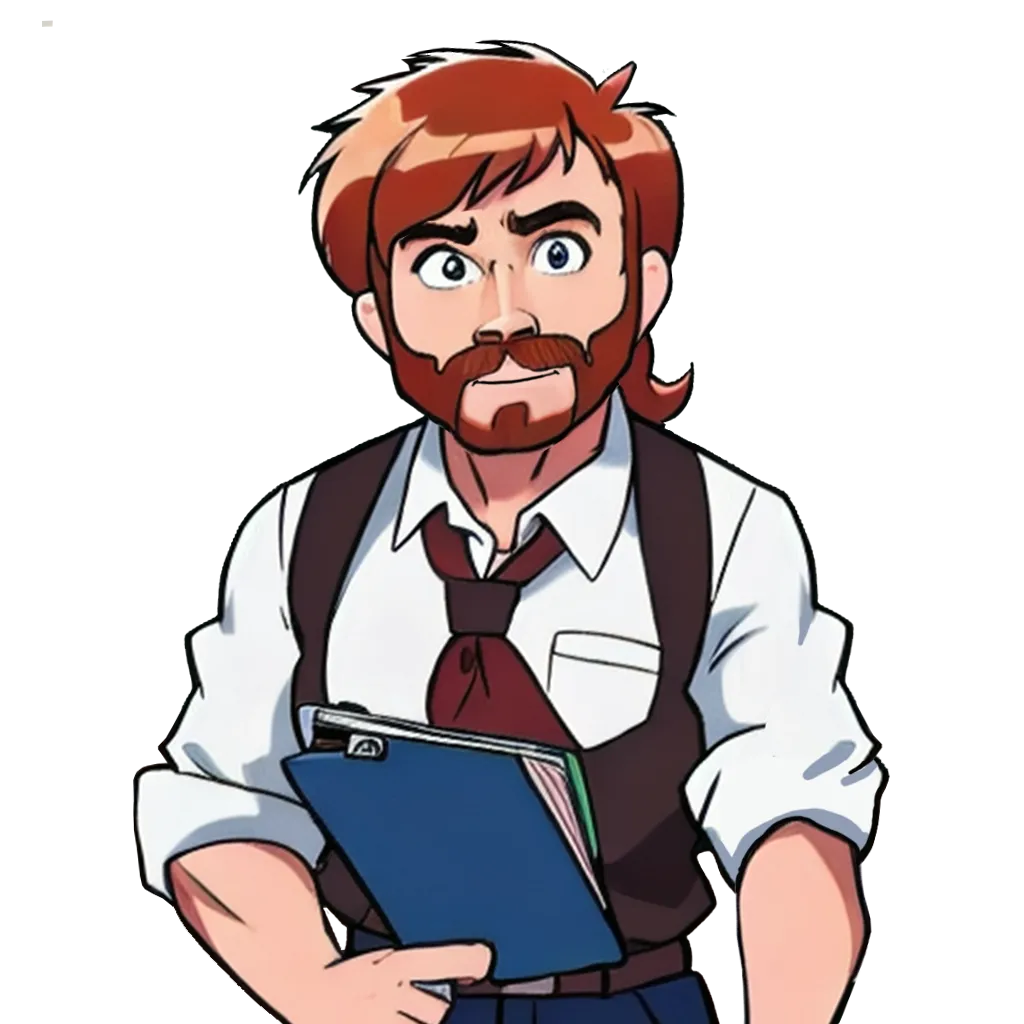
- Introduction to Accessibility in HTML
- Web Content Accessibility Guidelines (WCAG)
- Semantic HTML for Accessibility
- Accessible Forms and Inputs
- Accessible Images, Media, and Graphics
- Accessible Navigation and Focus Management
- ARIA: Accessible Rich Internet Applications
- Accessibility in Custom Components
- Evaluación y Pruebas de Accesibilidad
- Relationship Between Performance and Accessibility
- Creating an Accessible Workflow
- Course Conclusion: Accessibility in HTML