Progressive Web Apps with HTML5 (PWA)
Service Workers in Depth
In this chapter, we will delve into service workers, the core of Progressive Web Apps (PWAs). Service workers are scripts that act as intermediaries between the application, the browser, and the network, enabling features such as caching, request handling, and push notifications. Understanding their lifecycle and how to implement them correctly is essential to leveraging their full potential.
What are Service Workers?
A service worker is a JavaScript file that runs in the background and does not have direct access to the DOM. It acts as a proxy between the application and the network, allowing it to intercept requests, store data in cache, and handle events like push notifications.
Service Worker Lifecycle
The lifecycle of a service worker is key to understanding how they work and how to implement updates without interrupting the user experience.
- Installation: Occurs when the browser detects a new file or changes in the service worker.
- Waiting: The new service worker waits until the previous one stops controlling open pages.
- Activation: The new service worker activates and takes control of the pages.
- Interception: Intercepts requests and handles tasks like responding from the cache.
Technical Example: Service worker lifecycle
javascript
Cache Strategies
Service workers enable the implementation of various caching strategies depending on the application's needs:
- Cache First: Searches the cache first, and if the resource is not found, requests it from the network.
- Network First: Attempts to get the resource from the network and stores it in cache for future requests.
- Stale While Revalidate: Returns the resource from the cache and updates the version in the background.
Technical Example: Cache First Strategy
javascript
Service Worker Update
Updating a service worker involves registering a new one and carefully managing the transition to avoid disrupting users. During activation, it is essential to delete obsolete caches to optimize performance.
Debugging Service Workers
Browser development tools, like Chrome DevTools, offer a dedicated service worker tab. From there, you can:
- View registered service workers.
- Update them manually.
- Delete obsolete caches.
- Simulate an offline application.
Conclusion
Service workers are a powerful tool that allows PWAs to offer advanced features such as offline support and efficient caching. In the next chapter, we will explore how to make the most of manifest files to customize the behavior and appearance of your PWA. Keep learning to build high-performance web applications!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
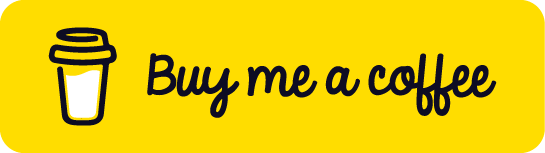
Chat with Chuck
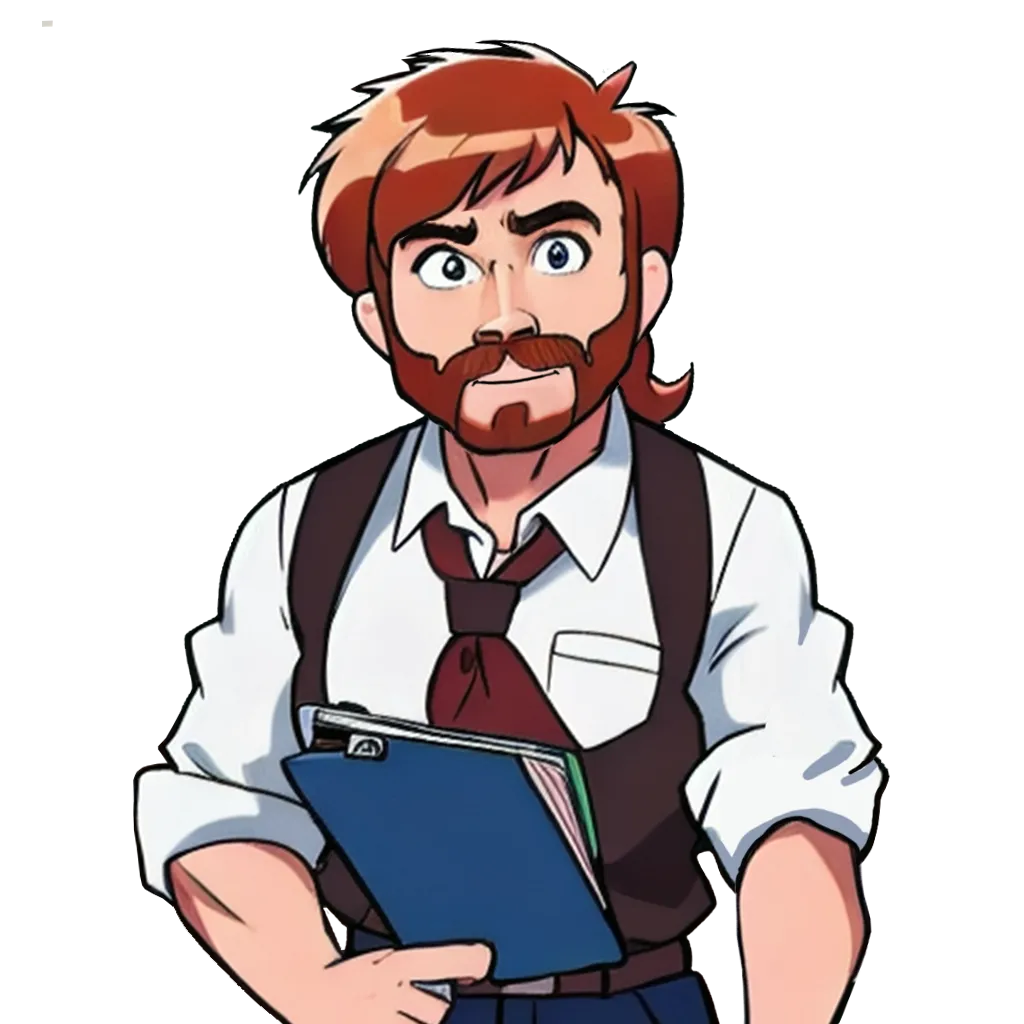
- Introduction to Progressive Web Apps (PWAs)
- Core Concepts of Progressive Web Apps
- Setting Up Your First Progressive Web App
- Service Workers in Depth
- The Manifest File of PWAs
- Offline Functionality in Progressive Web Apps
- Performance Optimization in Progressive Web Apps
- Advanced Features in Progressive Web Apps
- Deploying and Distributing Your Progressive Web App
- Integration of PWAs with Other Web APIs
- Debugging and Testing Progressive Web Apps
- The Future of Progressive Web Apps
- Conclusion of the Progressive Web Apps Course