Streaming and Buffering in Node
Backpressure in Node.js Streams
What is Backpressure?
Backpressure is a crucial concept in stream management, referring to the situation where the writable stream cannot handle the speed at which a readable stream is sending data. Backpressure helps to prevent memory overload and maintain a balanced data flow between the producer (readable stream) and the consumer (writable stream).
How Backpressure Works in Node.js
In Node.js, streams manage backpressure automatically. If a writable stream cannot write data as quickly as it receives it, the readable stream is paused until the writable stream can process more data.
Example of Backpressure in Streams
Below is an example where a readable stream tries to send data faster than a writable stream can handle.
javascript
In this example, FastReadable
produces data very quickly, while SlowWritable
simulates a slow writing process. Backpressure is handled automatically, pausing FastReadable
when SlowWritable
is busy.
Manual Backpressure Control
In addition to automatic handling, you can also manage backpressure manually by checking the return value of the writable stream's write
method.
javascript
In this example, customReadable
pauses data production if customWritable
cannot handle more data (write
returns false
) and resumes when the drain
event is emitted.
Summary
Backpressure is an essential feature for maintaining efficiency and stability in data flow in stream-based systems. Understanding and managing backpressure will allow you to create scalable and efficient applications in Node.js.
Backpressure Diagram
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
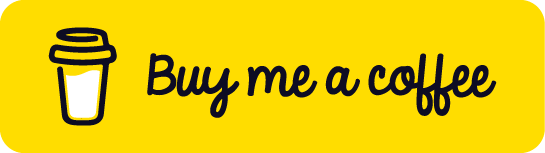
Chat with Chuck
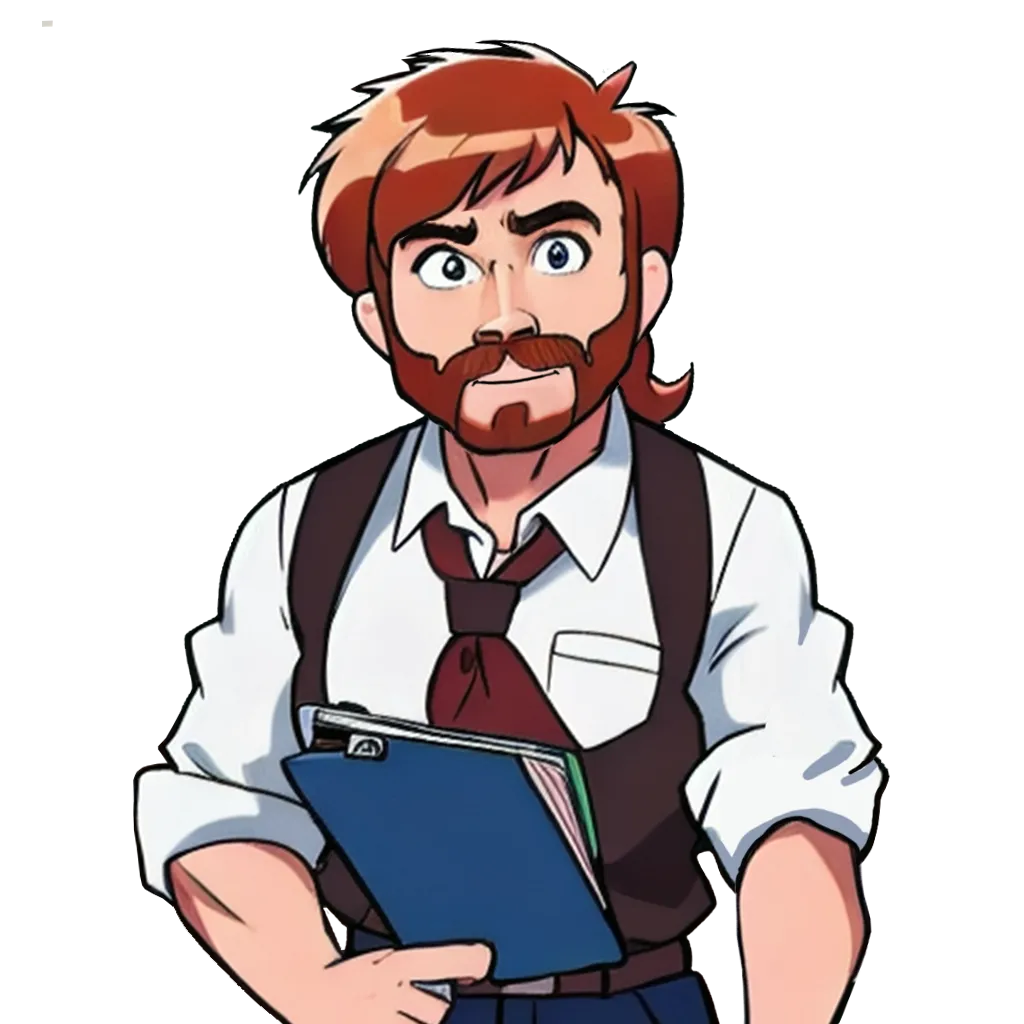
- Introduction to the Course on Streaming and Buffering in Node.js
- Understanding Streams in Node.js
- Understanding Buffering in Node.js
- Using Pipes with Streams in Node.js
- Transform Streams in Node.js
- Leyendo Archivos Usando Streams en Node.js
- Writing Files Using Streams in Node.js
- Duplex Streams in Node.js
- Error Handling in Streams in Node.js
- Backpressure in Node.js Streams
- Network Connection Streaming in Node.js
- Streaming Large Files in Node.js
- Streams with Compression in Node.js