Streaming and Buffering in Node
Transform Streams in Node.js
What is a Transform Stream?
A transform stream is a special type of stream that is both readable and writable, and it can modify or transform the data that passes through it. It is useful in situations where you need to modify data in real-time while you are reading or writing it.
Comparable with Duplex Streams
Although transform streams are technically duplex streams, their particularity is that the data written in them is transformed when read. Common examples include data compression and encryption.
Creating a Transform Stream
To create a transform stream, you can extend the Transform
class from the stream
module and override the _transform
method.
javascript
In this example, any data that passes through MyTransformStream
will be converted to uppercase.
Using Transform Streams
Below is how to use MyTransformStream
in combination with other streams.
javascript
Practical Example: Data Compression
A common application of a transform stream is data compression. Here is an example using zlib
to compress data in real-time.
javascript
Benefits of Using Transform Streams
- Flexibility: Allows dynamically modifying data as it passes through the stream.
- Efficiency: Does not require storing large amounts of data in memory, which is ideal for real-time operations.
- Combinability: Can be easily combined with other types of streams using pipes.
Summary
Transform streams provide a powerful and efficient way to manipulate data in real-time in Node.js. Learning to use them will enable you to perform more complex data processing operations with ease.
Transform Streams Explained
- Introduction to the Course on Streaming and Buffering in Node.js
- Understanding Streams in Node.js
- Understanding Buffering in Node.js
- Using Pipes with Streams in Node.js
- Transform Streams in Node.js
- Leyendo Archivos Usando Streams en Node.js
- Writing Files Using Streams in Node.js
- Duplex Streams in Node.js
- Error Handling in Streams in Node.js
- Backpressure in Node.js Streams
- Network Connection Streaming in Node.js
- Streaming Large Files in Node.js
- Streams with Compression in Node.js
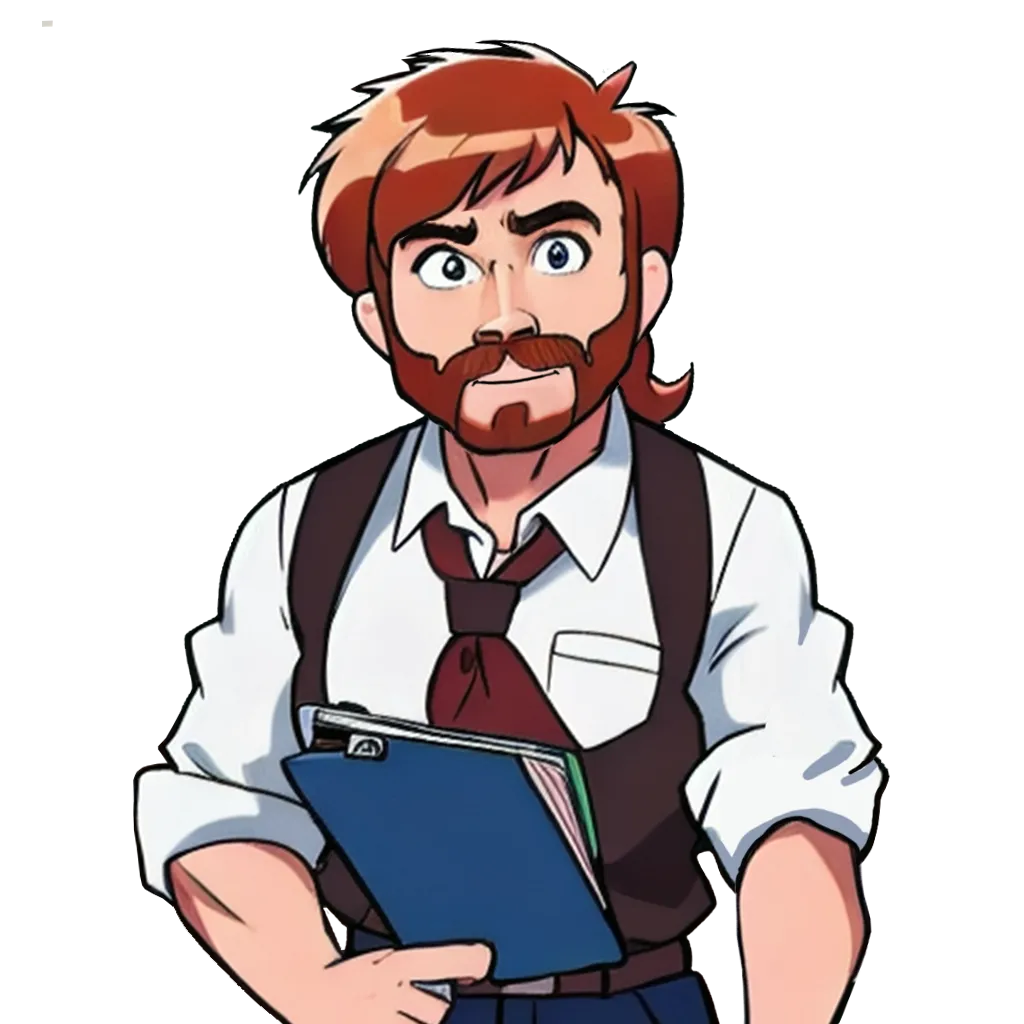