Streaming and Buffering in Node
Writing Files Using Streams in Node.js
Introduction to Writing Files with Streams
Writing files using streams is an efficient technique for handling large volumes of data effectively in Node.js. Unlike traditional synchronous or asynchronous writing, writable streams allow you to write files in parts or 'chunks', which can significantly improve performance and reduce memory usage.
Creating a Writable Stream
To write a file using streams, you use the createWriteStream
method from the fs
module.
Basic Example
javascript
In this example:
- A writable stream for the
file.txt
file is created. - An 'utf8' encoding is specified to make the written data text strings.
Event Handling
Writable streams emit several important events:
finish
: Emitted when all the data has been written.error
: Emitted in case of an error during writing.
Writing Data in Chunks
Writing data in chunks can be extremely useful when dealing with large volumes of data.
javascript
Full Usage Example
Below is a more complete example that includes writing data in chunks and event handling.
javascript
Advantages of Using Streams for Writing Files
- Memory Efficiency: You don't need to load all the data into memory before writing.
- Speed: Allows writing data as it is generated, improving processing speed.
- Flexibility: Can be used to handle real-time data flow.
Summary
Writing files using streams in Node.js is an efficient technique that improves memory management and allows you to process and write large volumes of data effectively. This knowledge is essential for working with Node.js in a professional and optimized manner.
Writing Files with Streams Diagram
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
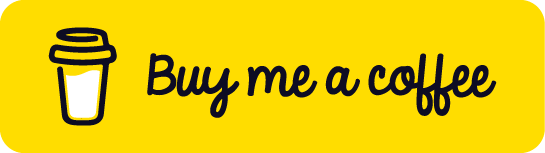
Chat with Chuck
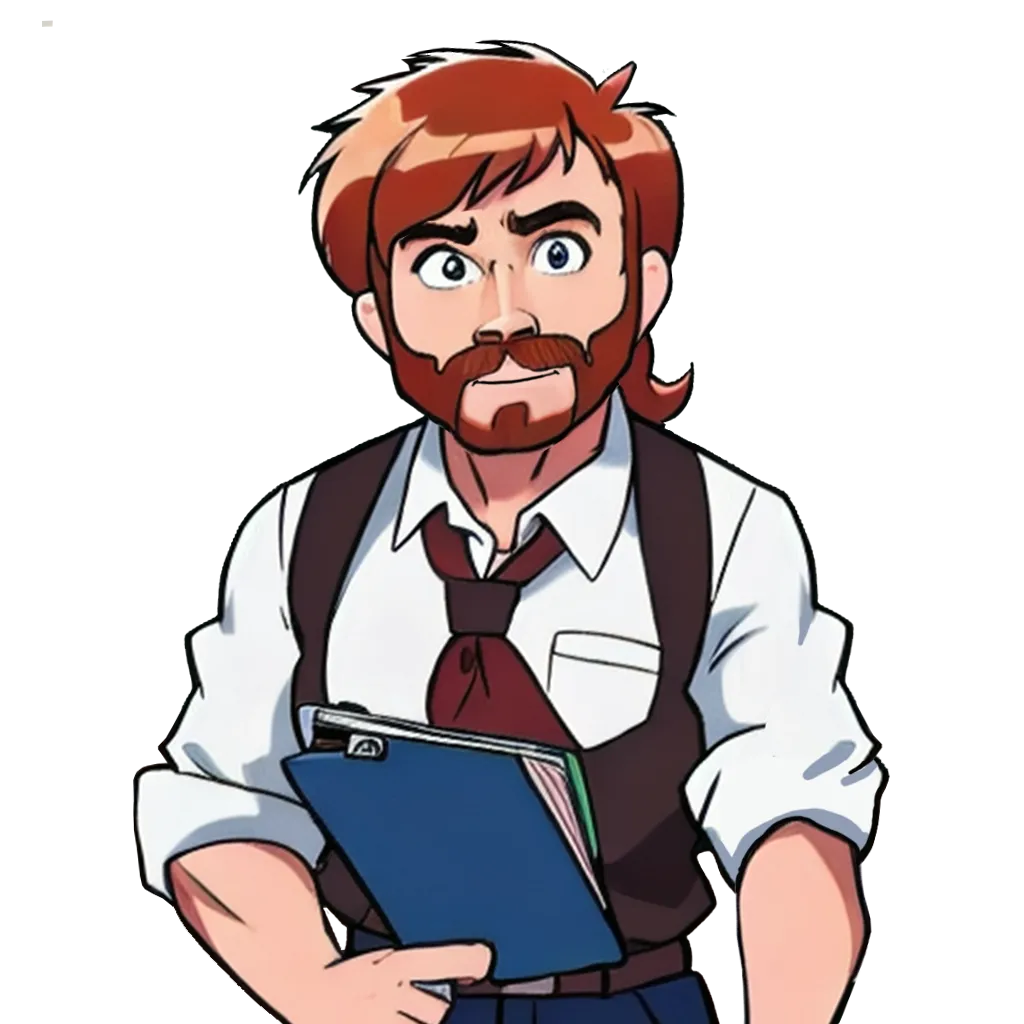
- Introduction to the Course on Streaming and Buffering in Node.js
- Understanding Streams in Node.js
- Understanding Buffering in Node.js
- Using Pipes with Streams in Node.js
- Transform Streams in Node.js
- Leyendo Archivos Usando Streams en Node.js
- Writing Files Using Streams in Node.js
- Duplex Streams in Node.js
- Error Handling in Streams in Node.js
- Backpressure in Node.js Streams
- Network Connection Streaming in Node.js
- Streaming Large Files in Node.js
- Streams with Compression in Node.js