Streaming and Buffering in Node
Streaming Large Files in Node.js
Introduction to Streaming Large Files
Handling large files can be challenging, especially if you try to load all the file's content into memory. Node.js, with its event-driven architecture and streams, provides an efficient solution for streaming large files for reading and writing without consuming much memory.
Reading Large Files with Streams
To read a large file, you can use fs.createReadStream()
, which reads the file in small chunks, allowing you to process each chunk of data without loading the entire file into memory.
Basic Example
javascript
In this example, the file is read in 16 KB chunks, which avoids fully loading the file into memory.
Writing Large Files with Streams
To write large amounts of data to a file, you can use fs.createWriteStream()
, which allows you to write data in chunks.
Basic Example
javascript
Copying Large Files Using Streams
One of the most common operations is copying large files. Using streams, you can efficiently read from one file and write to another.
File Copy Example
javascript
Benefits of Streaming Large Files
- Memory Efficiency: Using streams avoids loading the entire file into memory, which is crucial for large files.
- Speed: Streams allow data to be processed as it is read/written, improving speed compared to reading/writing the entire file at once.
- Error Handling: Streams provide events to efficiently handle read/write errors.
Summary
Streaming large files in Node.js is an effective and efficient technique for handling large volumes of data without compromising the application's memory and performance. Using streams, you can read and write large files efficiently and handle common operations such as copying large files with ease.
Large Files Streaming Diagram
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
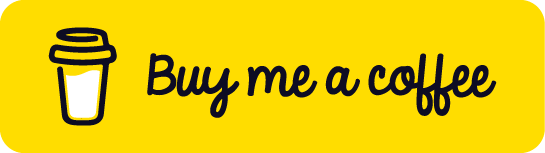
Chat with Chuck
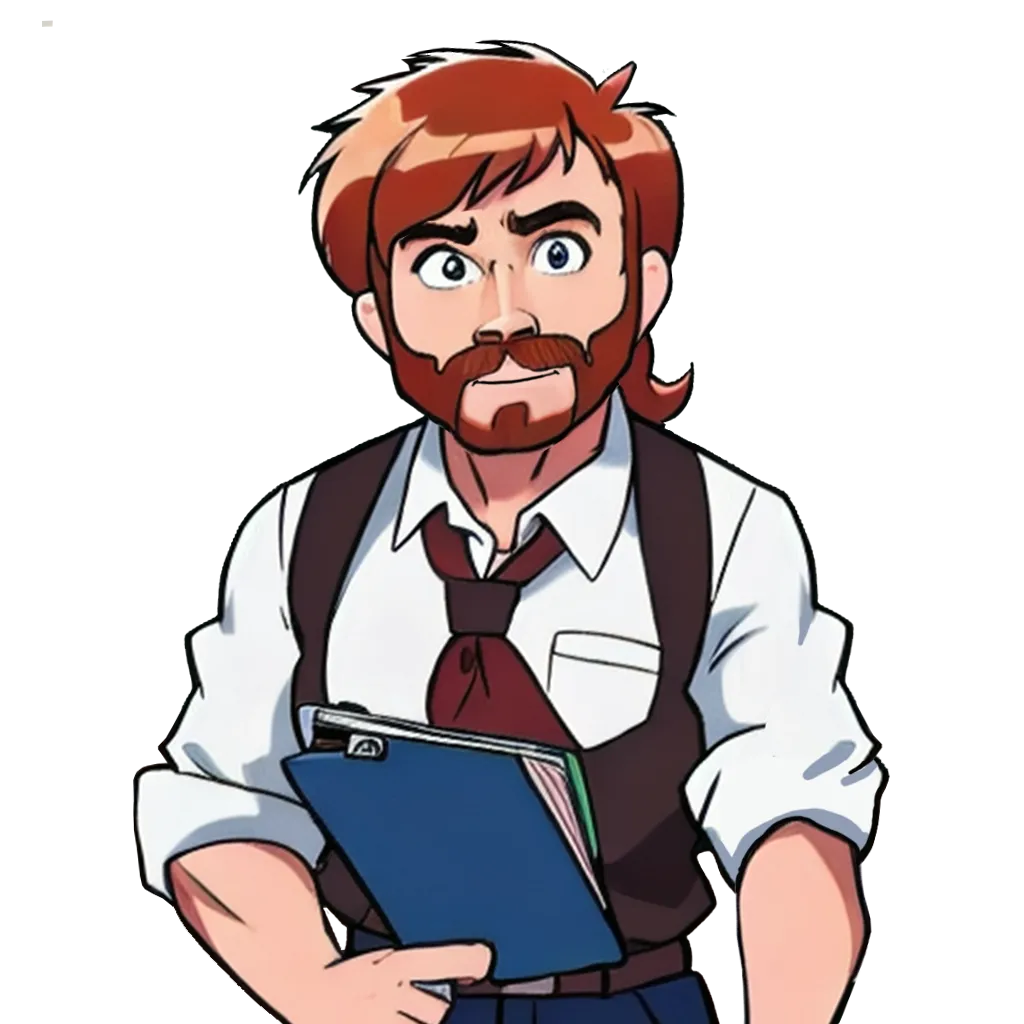
- Introduction to the Course on Streaming and Buffering in Node.js
- Understanding Streams in Node.js
- Understanding Buffering in Node.js
- Using Pipes with Streams in Node.js
- Transform Streams in Node.js
- Leyendo Archivos Usando Streams en Node.js
- Writing Files Using Streams in Node.js
- Duplex Streams in Node.js
- Error Handling in Streams in Node.js
- Backpressure in Node.js Streams
- Network Connection Streaming in Node.js
- Streaming Large Files in Node.js
- Streams with Compression in Node.js