Integration of TypeScript in Existing Projects
Handling Libraries and Dependencies in TypeScript
Handling Libraries and Dependencies in TypeScript
When working on a TypeScript project, it is common to need to use external libraries and packages. In this section, we will learn how to manage these dependencies and ensure that TypeScript recognizes them correctly.
Installing Type Definitions
Many JavaScript libraries do not include type definitions. To solve this, the DefinitelyTyped project provides type definitions that can be installed via npm using the @types
prefix.
Example with lodash
Suppose you need to use the lodash
library in your TypeScript project.
- Install the
lodash
library and its corresponding type definition:
sh
- Now you can import and use
lodash
with type safety:
ts
Libraries without Type Definitions
If a library does not have available type definitions, you can create your own definitions or use declare
to add basic types.
Example with a fictitious library
Suppose we are using a library called fancy-library
, which does not have type definitions.
- Install the library:
sh
- Create a type definition file for the library named
fancy-library.d.ts
in an@types
directory:
ts
- Now you can import and use
fancy-library
in your TypeScript code:
ts
Using CommonJS and ES6 Modules
TypeScript allows the use of both CommonJS and ES6 modules. Make sure to adjust your tsconfig.json
so that the module system is compatible with the environment you are working in.
Configuration for Node.js (CommonJS)
json
Configuration for Browsers (ES6 Modules)
json
Ambient Types
TypeScript allows you to declare ambient types for global libraries or any other types you want to declare at a global level. This is commonly done in d.ts
files.
Example of Global Declarations
Create a globals.d.ts
file:
ts
Now you can use myGlobalFunction
anywhere in your TypeScript project without the compiler throwing errors.
ts
Types for JSON and Other Resources
Sometimes you might need to import JSON resources or other types of files into your TypeScript project. You can declare types for these resources as well.
Example for JSON Files
Create a json.d.ts
file:
ts
Now you can import JSON files in your TypeScript project:
ts
Working with libraries and dependencies in TypeScript may seem a bit complicated at first, but understanding these basic concepts will help you smoothly integrate TypeScript into your existing project. In the next section, we will learn about code refactoring and type improvements.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
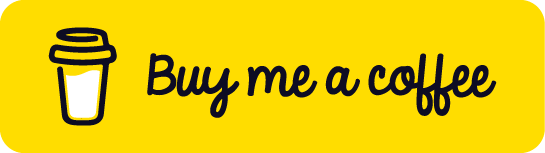
Chat with Chuck
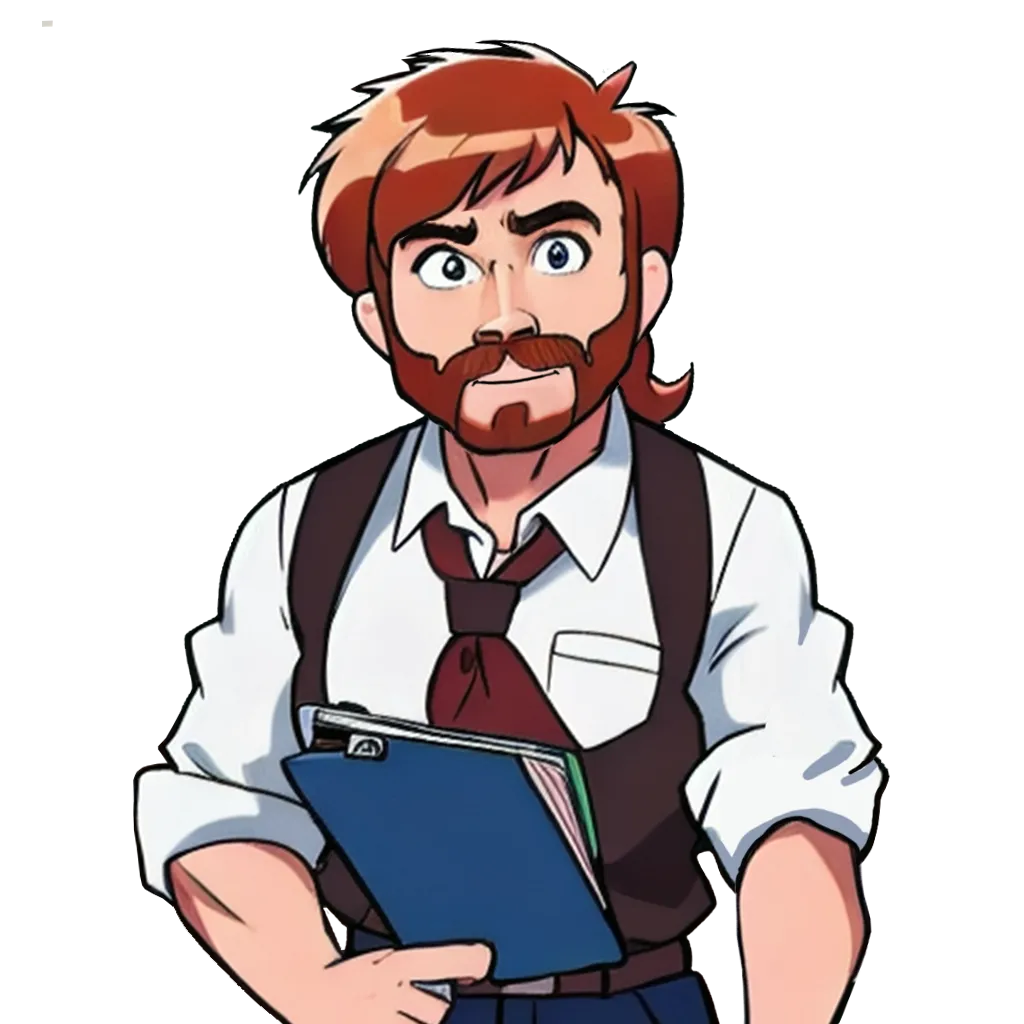