Integration of TypeScript in Existing Projects
Setting Up TypeScript in Existing Projects
Setting Up TypeScript in Existing Projects
In this module, we will learn how to set up TypeScript in an existing JavaScript project. We will see the steps to install TypeScript, create a tsconfig.json
configuration file, and configure our development environment to work with TypeScript.
Installing TypeScript
To start, we need to install TypeScript and its global type dependencies (@types/node
). Make sure Node.js and npm are installed on your system.
sh
Creating the tsconfig.json File
The tsconfig.json
file is where we configure TypeScript compiler options. You can create this file manually or generate it with the following command:
sh
This command will generate a tsconfig.json
file in the root of your project with a basic configuration. Here is an example of an initial tsconfig.json
file:
json
Important Parameters in tsconfig.json
- target: Specifies which version of JavaScript TypeScript should compile to. For example,
es6
,es5
. - module: Defines the module system to be used. The
commonjs
value is suitable for Node.js. - strict: Enables TypeScript's strict mode, which activates all type safety checks.
- esModuleInterop: Allows easier interoperability to import CommonJS and ES6 modules.
- skipLibCheck: Skips type checking on declaration files.
- forceConsistentCasingInFileNames: Ensures consistent file naming, especially useful on case-insensitive file systems.
Integrating with the Development Environment
It is useful to configure your development environment to work optimally with TypeScript. If you use Visual Studio Code, we recommend installing the following extensions:
- TypeScript Extension Pack: Includes a set of useful extensions for working with TypeScript.
- ESLint: To maintain clean and consistent code.
Configuring ESLint for TypeScript
ESLint can help maintain consistent and clean code. To configure ESLint with TypeScript, install the following dependencies:
sh
Add or update your .eslintrc.json
file to include the necessary configurations for TypeScript:
json
Placeholder for an image showing the process of installing dependencies and configuring the tsconfig.json file in Visual Studio Code
Creating the First TypeScript File (hello.ts)
Create a src/hello.ts
file and add the following code to ensure everything is set up correctly:
ts
To compile the file, run:
sh
The compiler will generate a hello.js
file in the same directory if everything is configured correctly.
Congratulations! You have set up TypeScript in your existing project. In the next module, we will see how to start migrating JavaScript files to TypeScript.
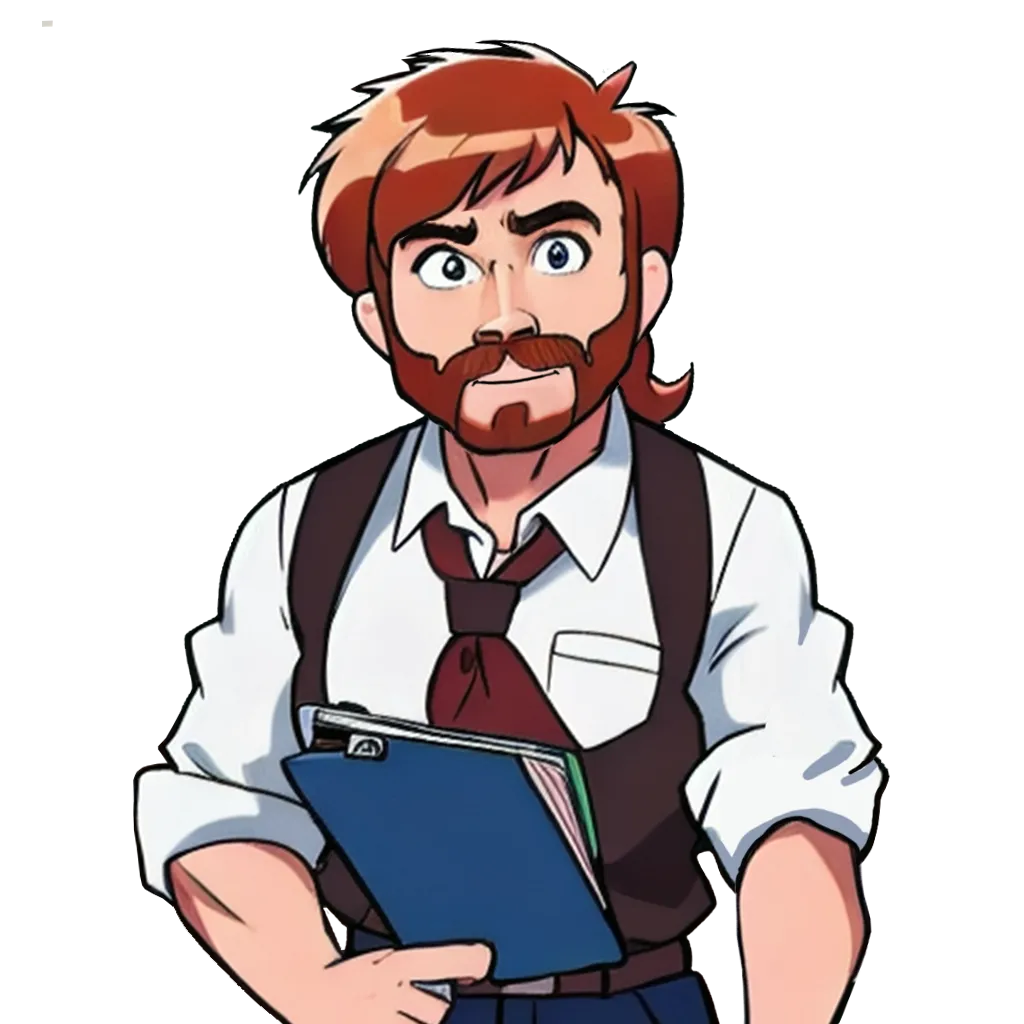