Integration of TypeScript in Existing Projects
Code Refactoring and Type Improvements
Code Refactoring and Type Improvements
Once you have migrated your files to TypeScript and handled your dependencies, the next step is to improve and refactor your code to take full advantage of TypeScript's capabilities. In this section, we will learn how to do this effectively.
Using Advanced Types
TypeScript offers a variety of advanced types that allow us to write more robust and expressive code. The most common include generic types, union types, intersection types, and literal types.
Generic Types
Generic types allow functions, classes, and interfaces to work with types that will be determined later when they are instantiated.
Example of a generic function:
ts
Union and Intersection Types
Union types allow a variable to have one of several types. Intersection types allow combining multiple types.
Example of union types:
ts
Example of intersection types:
ts
Refactoring Existing Code
Refactoring is an essential process to improve the quality of your code. With TypeScript, you can refactor your code to make it easier to maintain and less error-prone.
Refactoring Functions
Consider the following example of a JavaScript function that processes data:
js
When converting this function to TypeScript, we can leverage static typing to make it safer and clearer:
ts
Refactoring Classes
Consider a JavaScript class that manages users:
js
When converting this class to TypeScript, we can add types to the properties and methods, making it more consistent and safe:
ts
Using Type Inference
TypeScript is very good at inferring types automatically, which can simplify your code. However, in some cases, explicitly declaring types can make your intention clearer.
Example without Inference:
ts
Example with Inference:
ts
Improving Code Readability and Maintainability
Using interfaces and custom types can improve the readability and maintainability of your code.
Example without Interface:
ts
Example with Interface:
ts
By following these refactoring and type improvement practices, you will not only improve the quality of your code but also make the most out of TypeScript. In the next module, we will conclude the course and discuss the next steps to deepen your understanding of TypeScript.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
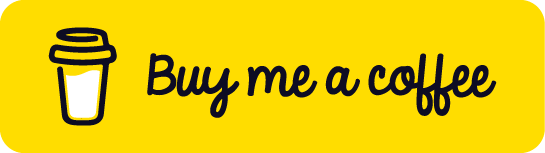
Chat with Chuck
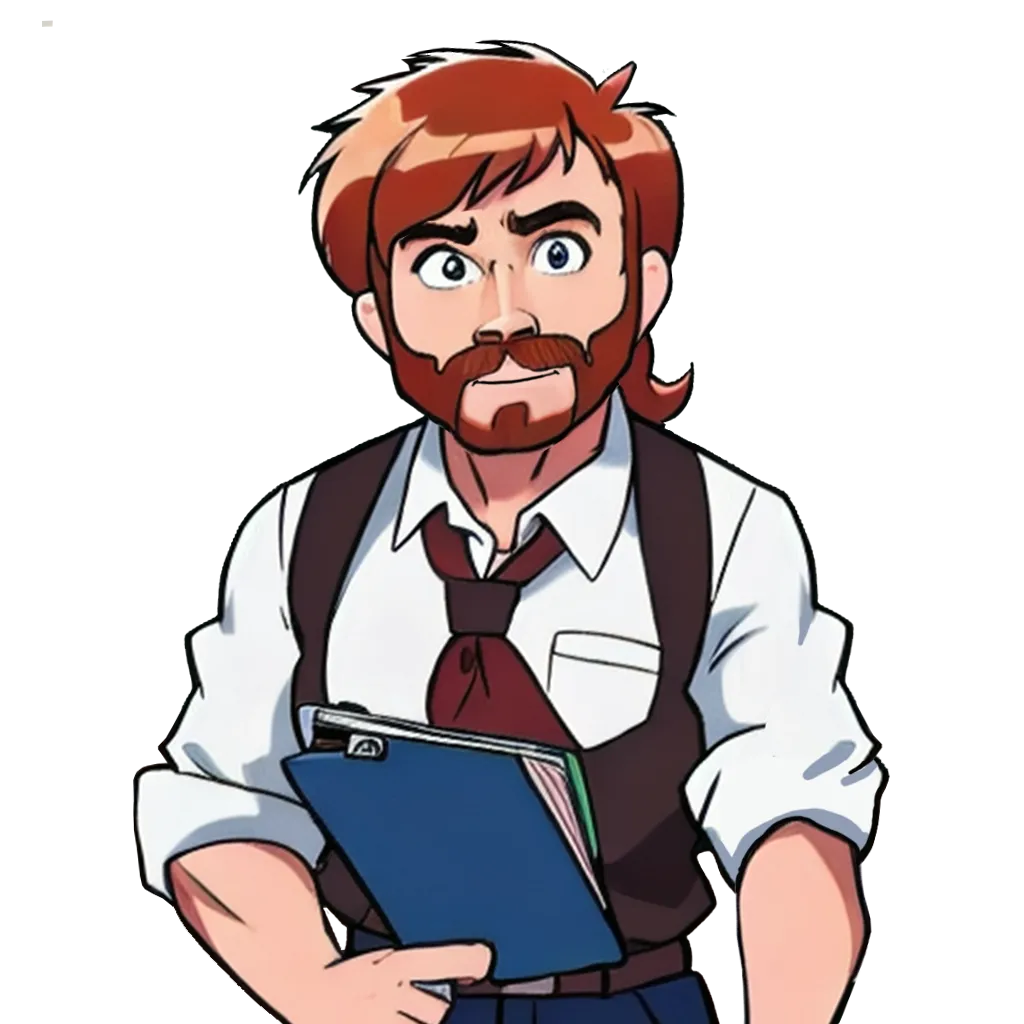