Integration of TypeScript in Existing Projects
Migration of JavaScript Files to TypeScript
Migration of JavaScript Files to TypeScript
Once we have set up TypeScript in our project, the next step is to gradually start migrating JavaScript files to TypeScript. In this section, we will address strategies and best practices to successfully carry out this migration.
Migration Strategy
Migrating an entire project to TypeScript at once can be daunting, especially for large projects. Here are some approaches to make the transition gradually:
- Module-based Migration: Start by converting one module or functionality at a time.
- File-based Migration: Convert a JavaScript file to TypeScript and then perform the corresponding tests.
- Type-based Migration: Add types to key functions and variables first before converting the entire file to TypeScript.
Migrating a Simple File
Imagine we have a JavaScript file called src/utils.js
that contains the following functions:
js
To migrate this file to TypeScript:
- Rename the file from
utils.js
toutils.ts
. - Add types to the functions.
- Adjust any syntax that is not compatible with TypeScript.
ts
Migrating a More Complex File
Here we have a more complex JavaScript file that handles a list of users:
js
To migrate this file:
- Rename the file from
users.js
tousers.ts
. - Add an interface for users and types to the functions.
- Adjust the export to TypeScript style.
ts
Type Checking
TypeScript offers type-checking tools that help identify errors before code execution. This feature is especially useful when migrating files, as you can spot discrepancies during development.
To check types in your project, run the command tsc
with no additional options. This will compile and verify all TypeScript files in your project according to the configuration in your tsconfig.json
.
sh
Handling JavaScript Modules
During the migration, you might need to work with JavaScript modules that haven't been migrated yet. To handle these cases, you can include // @ts-ignore
declarations or create temporary type definitions. However, it is best to try to migrate complete modules to get all the benefits of TypeScript.
ts
As you become more comfortable with TypeScript, the migration will become smoother. In the next section, we will address how to handle libraries and dependencies in a TypeScript environment.
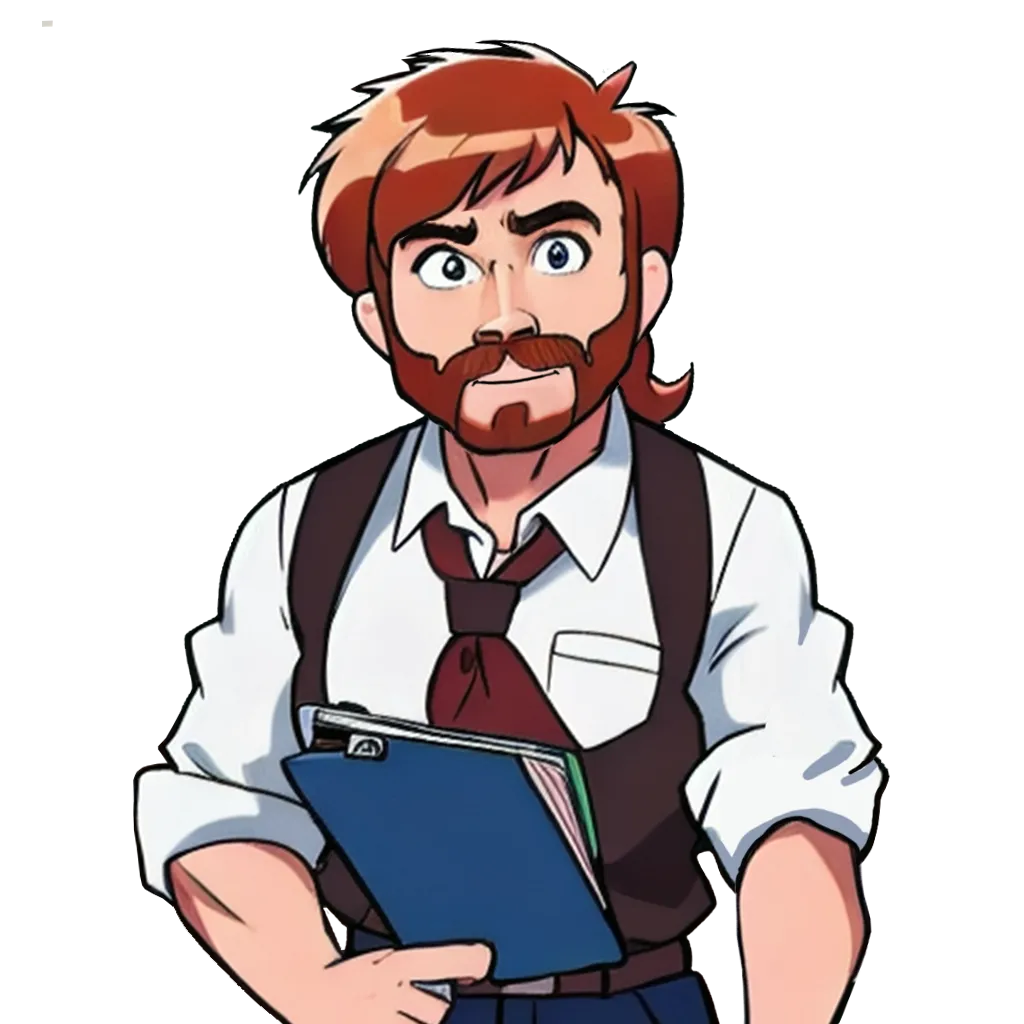