Web Storage API in HTML5
Advanced Techniques with Web Storage
In this chapter, we will explore advanced techniques to maximize the use of localStorage
and sessionStorage
. These techniques will help you manage data more effectively and improve the user experience in complex applications. We will discuss how to implement expiration logic, synchronize data between tabs and windows, and combine HTML storage with other solutions like IndexedDB to store complex data.
Implementing Expiration in localStorage
Although localStorage
does not have a built-in expiration capability, we can simulate it by adding a time logic to the stored data. This is useful when certain data needs to expire after a set time.
Example of Expiration Implementation
- Store Data with a Timestamp
javascript
- Retrieve Data and Check for Expiration
javascript
Data Synchronization Between Tabs and Windows
In web applications, it can be useful to synchronize the state of data between different tabs or browser windows. We can achieve this by leveraging the storage
event, which is triggered when localStorage
is modified in a tab.
Synchronization Example with the storage
Event
javascript
Combining localStorage
with IndexedDB
For applications that require storing large volumes of data or complex data, combining localStorage
with IndexedDB is an effective option. localStorage
is suitable for configuration and state data, while IndexedDB is ideal for storing large data collections and complex queries.
Combined Usage Example
javascript
Performance Optimization in Web Storage
When working with localStorage
and sessionStorage
, it's important to optimize performance so that data access and manipulation are efficient:
- Minimize
localStorage
Use for Large Data: WhilelocalStorage
is convenient, it is not ideal for large amounts of data. For massive data, consider alternatives like IndexedDB. - Batch Accesses to
localStorage
: Since each access tolocalStorage
involves I/O operations, it is a good practice to batch reads and writes when possible. - Data Compression: In cases where a significant amount of data is stored in
localStorage
, compressing the data strings can reduce the occupied space, although it requires the use of external libraries.
Security Considerations in Advanced Web Storage Usage
Security should be a priority when implementing advanced web storage techniques:
- Protection Against XSS: Ensure user inputs are sanitized before storing them in
localStorage
orsessionStorage
to prevent XSS attacks. - Avoid Sensitive Data: Do not store sensitive data in
localStorage
orsessionStorage
, as they may be accessible by malicious scripts if a vulnerability occurs. - Implement Expiration for Sensitive Data: If you need to store temporary data, ensure an expiration mechanism like the one we described earlier is implemented.
Conclusion
In this chapter, we explored advanced techniques to enhance the use of localStorage
and sessionStorage
, including data synchronization between tabs, combination with IndexedDB, and custom expiration implementation. These techniques can significantly improve the functionality and security of web applications. In the next chapter, we will work on practical projects to apply all these concepts in real-world situations and learn to manage data storage optimally.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
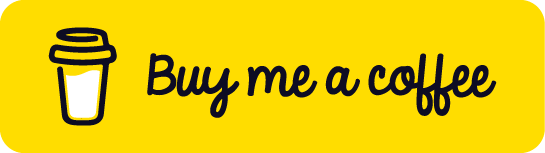
Chat with Chuck
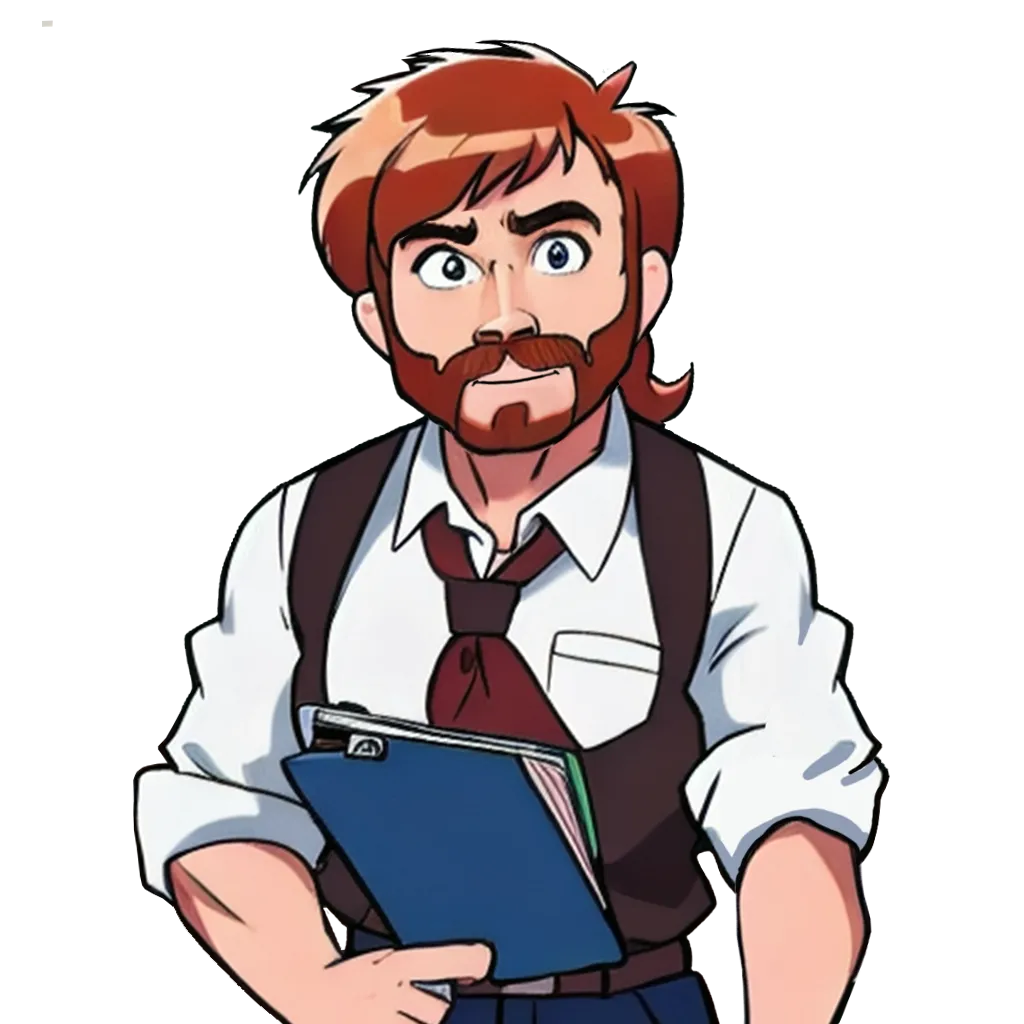