Web Storage API in HTML5
Security and Privacy in Web Storage
Security and privacy are critical aspects when using localStorage
and sessionStorage
in web applications. Since HTML storage allows data to be saved directly in the user's browser, precautions must be taken to protect that information and prevent vulnerabilities that could compromise user data. In this chapter, we will analyze the main security threats, such as Cross-Site Scripting (XSS) attacks, and discuss best practices to ensure the...
Main Security Threats in Web Storage
Cross-Site Scripting (XSS) Attacks
One of the biggest threats to localStorage
and sessionStorage
is Cross-Site Scripting (XSS). In an XSS attack, an attacker injects malicious scripts into a web application, which can then be executed in the user's browser. If those scripts have access to localStorage
, they can read sensitive data or alter stored data.
XSS Attack Example
Suppose an application stores an authentication token in localStorage
. If an attacker manages to inject a malicious script, that script could access the token and send it to an external server.
javascript
Accessibility of Storage in JavaScript
Since localStorage
and sessionStorage
are accessible from any script on the same domain, it is essential to avoid storing sensitive information that could be exploited by malicious scripts. Unlike cookies with the HttpOnly
option, HTML storage does not have similar protection limiting access to JavaScript.
Best Practices for Security in Web Storage
To protect data in localStorage
and sessionStorage
, it is important to follow a series of best practices that reduce the risk of security vulnerabilities:
Avoid Storing Sensitive Information
Never store sensitive information, such as passwords, access tokens, or confidential personal data, in localStorage
or sessionStorage
. Instead, use secure server-side mechanisms or, if necessary, secure cookies with the HttpOnly
option.
Implement Sanitization and Validation Measures for Inputs
To prevent XSS attacks, ensure to sanitize and validate all user inputs before storing them in localStorage
. This helps prevent malicious scripts from being executed in the user's browser.
javascript
Implement Expiration for Sensitive Data
If it is absolutely necessary to store temporary data, implement an expiration mechanism to reduce the time data remains in storage.
javascript
Monitoring Storage Events
The storage
event allows monitoring changes in localStorage
across different tabs or browser windows. This can be useful to detect if any critical data in storage has been modified.
javascript
Safer Alternatives to HTML Storage
If you need to store sensitive or high-priority information, consider safer alternatives:
- Cookies with
HttpOnly
andSecure
: Cookies can be configured to be accessible only by the server, limiting access from JavaScript. - Server-Side Storage: Whenever possible, store critical data on the server, minimizing client-side storage use.
- Session Management Servers: Session management servers store user information securely on the server side, keeping only a session identifier on the client.
Conclusion
In this chapter, we addressed the security risks associated with using localStorage
and sessionStorage
, as well as best practices to mitigate those risks. Protecting user data is essential, and applying best security practices can help prevent attacks like XSS. In the next chapter, we will close the course with a summary of key points and a guide to exploring advanced storage topics in web development.
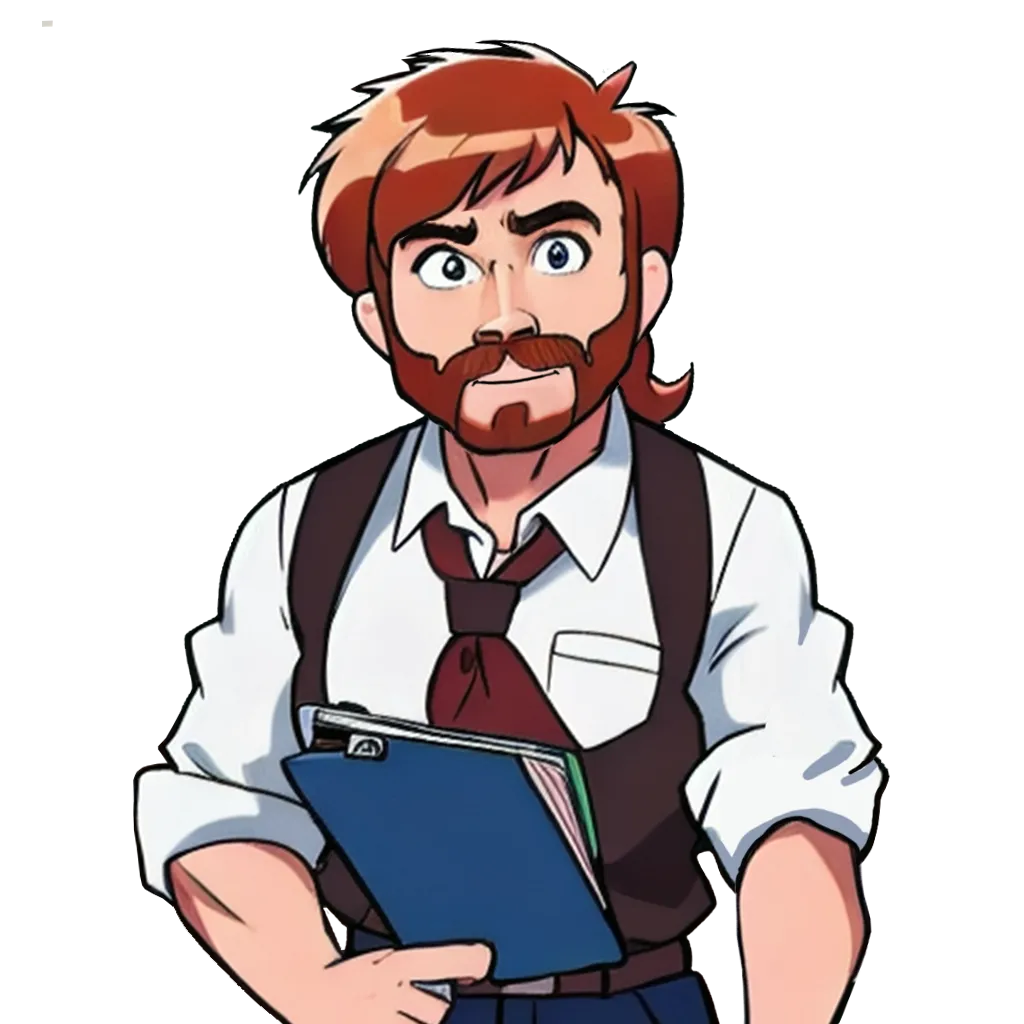