Web Storage API in HTML5
Understanding `localStorage`
In this chapter, we will explore localStorage
, one of the two storage options in HTML designed to store data persistently in the user's browser. localStorage
allows data to be stored on the client side without being sent to the server, making it ideal for saving configurations, preferences, and other information that the user needs to retain between browsing sessions.
What is localStorage
?
localStorage
is a browser storage API that allows web applications to store data in key-value pairs. This data remains in the browser even after the browser is closed or the device is turned off, until it is manually deleted or through a programmed action.
Main Features of localStorage
- Long-Term Persistence: Unlike
sessionStorage
, data inlocalStorage
is not deleted when the browser window or tab is closed. It remains in the browser until it is manually removed by the user or through code. - Domain Scope: Data stored in
localStorage
is only accessible within the same domain (same combination of protocol and domain name) that created it. This means data cannot be accessed from a different domain, enhancing security. - Storage Capacity:
localStorage
allows storing up to approximately 5-10 MB, depending on the browser, which is significantly larger than the 4 KB limit of cookies.
Basic Methods of localStorage
The localStorage
API offers several methods to easily manipulate data:
setItem(key, value)
: Saves a key-value pair inlocalStorage
.getItem(key)
: Retrieves the stored value associated with a specific key.removeItem(key)
: Removes the key-value pair fromlocalStorage
.clear()
: Deletes all data stored inlocalStorage
.
Examples of Using Basic Methods
Storing Data in localStorage
javascript
Retrieving Data from localStorage
javascript
Deleting Data from localStorage
javascript
Clearing All Content from localStorage
javascript
Using JSON
with localStorage
Since localStorage
only stores data as text strings, it's common to use JSON
to store complex objects. This is achieved by converting objects to JSON strings using JSON.stringify()
and retrieving them later with JSON.parse()
.
Example of Storing an Object
javascript
Retrieving an Object from localStorage
javascript
Practical Examples of Using localStorage
Storing User Preferences
A common use of localStorage
is to store user preferences, such as the page theme or the selected language. This allows the site to remember the user's settings between visits.
javascript
Storing Form Data
localStorage
can also be useful for storing form data, so users don't lose information if the page is reloaded accidentally.
javascript
Best Practices and Limitations of localStorage
Although localStorage
is extremely useful, it also has limitations and important considerations:
- Avoid Storing Sensitive Data: Due to its client-side accessibility,
localStorage
is not suitable for sensitive data such as passwords or access tokens. This is especially important because storage is vulnerable to Cross-Site Scripting (XSS) attacks. - Use JSON for Complex Data: Since
localStorage
only stores data in text string format, it is advisable to use JSON to store and retrieve complex data like objects or arrays. - Limited Capacity: Although the
localStorage
limit is larger than that of cookies, it is still relatively small compared to server storage. It is recommended not to uselocalStorage
for large amounts of data.
Conclusion
In this chapter, we have explored how localStorage
works and is used to store data persistently in the user's browser. We have seen how to use the main methods of localStorage
and how to store and retrieve complex data using JSON. In the next chapter, we will learn about sessionStorage
, another HTML storage option that allows storing temporary data that only persists during the browsing session.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
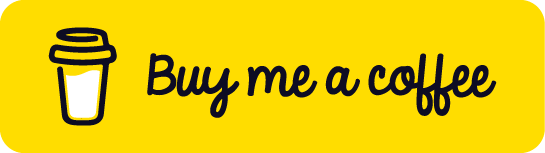
Chat with Chuck
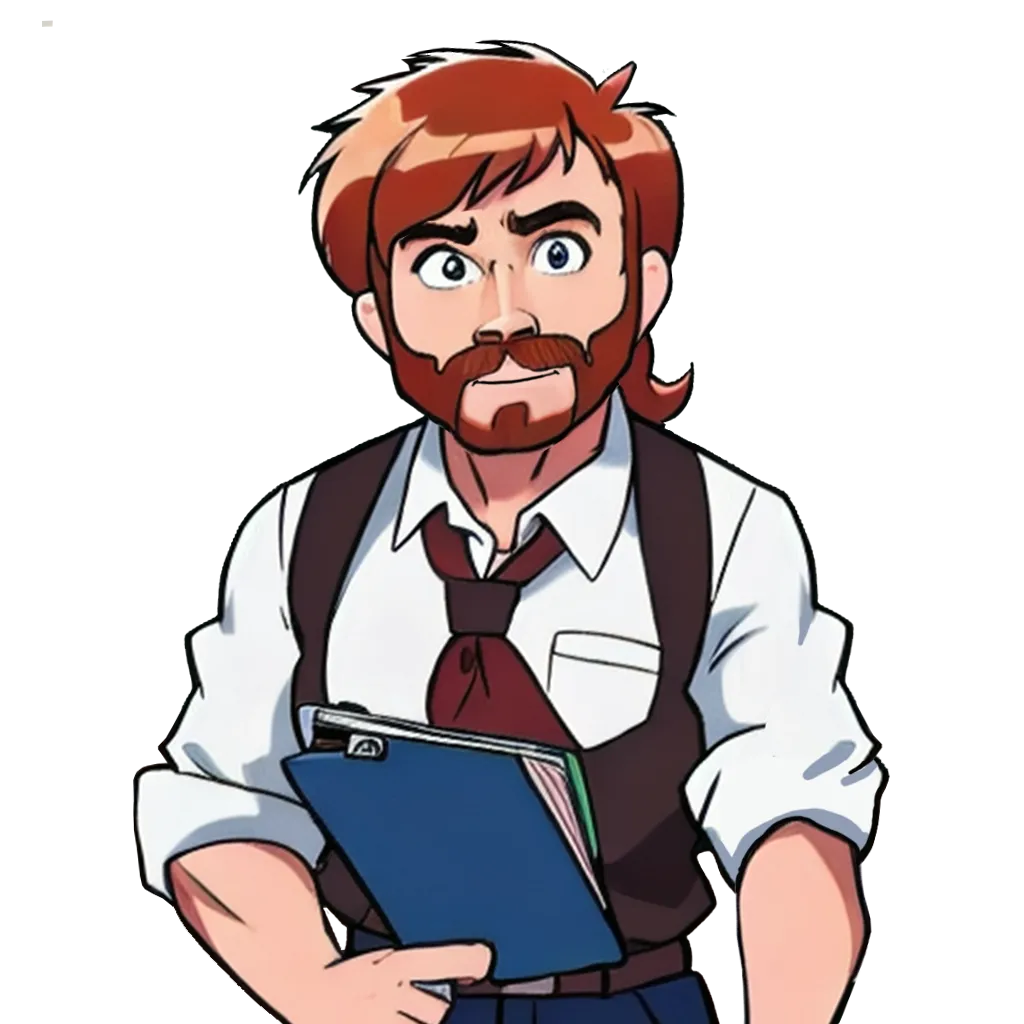