Web Storage API in HTML5
Data Management with the Storage API
In this chapter, we will explore data management in localStorage
and sessionStorage
using the Storage API. We will see how to store, retrieve, and manipulate data efficiently, as well as best practices to avoid performance and security issues in our web applications.
Basic Principles of Data Management
Using localStorage
and sessionStorage
to manage data in the browser involves understanding some basic principles for storing and organizing data efficiently:
- Data Structure:
localStorage
andsessionStorage
store data as key-value pairs in text format, so it is important to choose a data structure that facilitates its handling. - Serialization and Deserialization: To store complex data (such as objects or arrays), we must convert them into text strings using
JSON.stringify()
before storing andJSON.parse()
to retrieve them. - Size Control: Ensuring that the storage does not contain unnecessary data is crucial, especially due to capacity limits (5-10 MB). This involves regularly deleting or updating data.
Basic Data Management Operations
Data Storage and Retrieval
Basic operations include storing and retrieving data as key-value pairs. These methods allow us to easily store information and access it when needed.
Example of Data Storage
javascript
Example of Data Retrieval
javascript
Data Update and Deletion
It is common to update and delete data in storage. We can use the same setItem()
method to update an existing value or removeItem()
to delete a specific data.
Update a Value
javascript
Delete a Value
javascript
Complete Data Clearance
The clear()
function is useful for deleting all data stored in localStorage
or sessionStorage
, completely emptying the storage.
javascript
Handling Complex Data with JSON
Since localStorage
and sessionStorage
only store data in text format, it is necessary to convert complex data (objects and arrays) into JSON strings to store them.
Example of Storing a Complex Object
javascript
Retrieval and Parsing of a Complex Object
javascript
Best Practices for Data Management in Storage
To make the most of HTML storage and avoid issues, follow these best practices:
- Avoid Storing Sensitive Data: Since HTML storage is accessible from JavaScript, avoid storing confidential information, such as passwords or access tokens.
- Clean Unnecessary Data: To prevent the accumulation of unnecessary data, remove data that is no longer relevant for the session or persistence.
- Control Data Size: Given that
localStorage
andsessionStorage
have a capacity limit, do not store large amounts of data. If it is necessary to store a lot of information, consider using a server-side database.
Security Considerations
Security is a critical aspect when using HTML storage:
- Vulnerability to XSS (Cross-Site Scripting): Since data in
localStorage
andsessionStorage
is available to any script on the same origin, it is important to avoid XSS vulnerabilities by validating and sanitizing all input data. - Limited Domain Access: Both
localStorage
andsessionStorage
respect the same-origin policy, which protects data from being accessed by other domains. - Use of Temporary Data in
sessionStorage
: When data is only needed temporarily, it is better to usesessionStorage
, as it will be automatically deleted when the tab or window is closed.
Conclusion
The Storage API provides powerful tools for managing data in the user's browser. By mastering basic operations, handling complex data with JSON, and following best practices, we can make the most of localStorage
and sessionStorage
in our applications. In the next chapter, we will explore advanced techniques to optimize and control storage, including the use of storage events and combining with other storage solutions in the browser.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
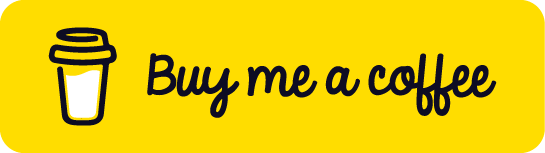
Chat with Chuck
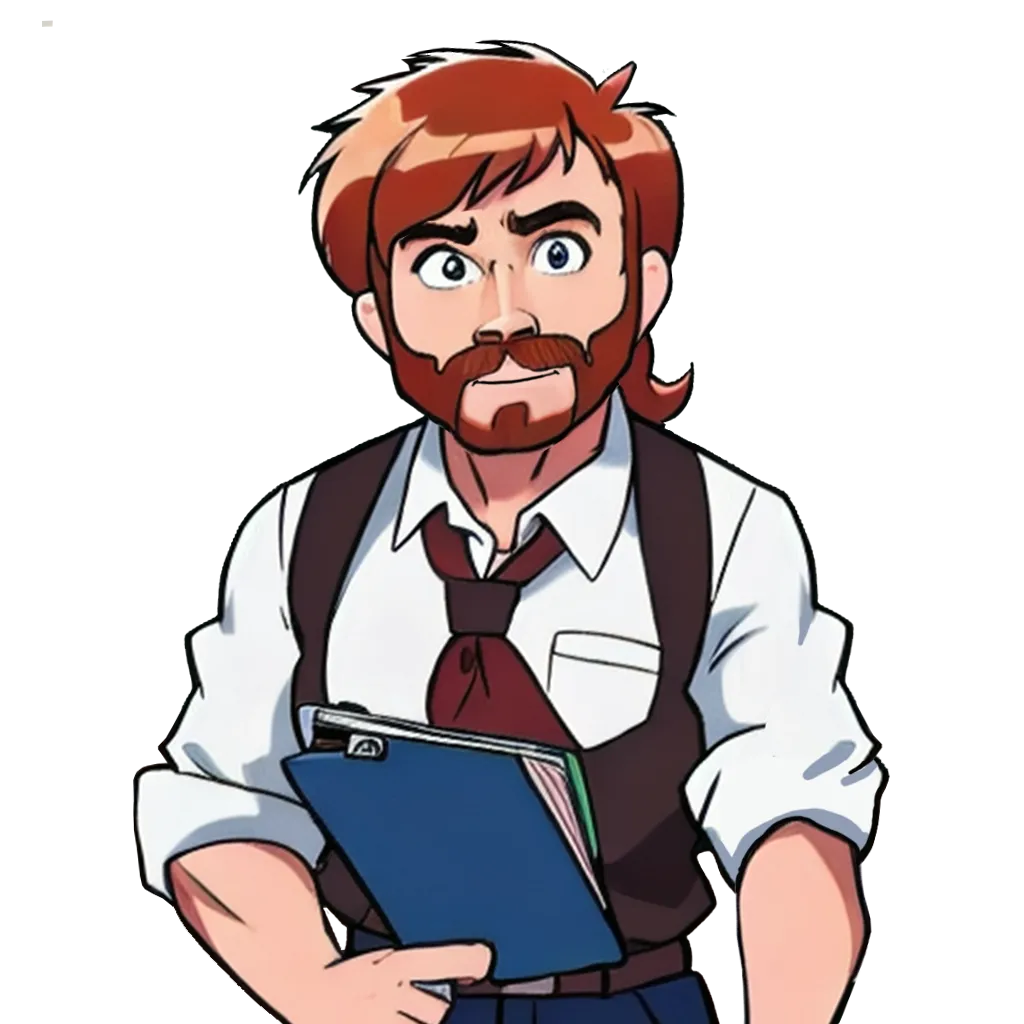