Git and GitHub
Advanced Git Usage (rebase, cherry-pick, etc.)
In this module, we will explore some advanced Git commands that will allow you to manage your workflow more efficiently and flexibly. These include rebase
, cherry-pick
, stash
, reset
, and bisect
.
Rebase
What is rebase?
Rebase is a way to integrate changes from one branch to another, applying your commits on top of the tip of another branch. Unlike merge
, rebase
rewrites the project history.
Basic usage of rebase
Suppose you have a feature
branch and you want to integrate the latest changes from main
:
bash
This command takes the changes from feature
and applies them immediately after the most recent commits in main
.
Interactive rebase
Interactive rebase allows you to rewrite commit history by combining, reordering, or editing commits. It is useful for cleaning up history before merging:
bash
Replace n
with the number of commits you want to rewrite. This will open a text editor and show a list of commits.
- pick: Use the commit as is.
- reword: Modify the commit message.
- edit: Edit the commit (content or message).
- squash: Combine the commit with the previous one.
Cherry-pick
What is cherry-pick?
cherry-pick
allows you to apply a specific commit from another branch to your current branch. It is useful when you need to apply a specific change without merging the entire branch.
Basic usage of cherry-pick
To apply a specific commit to the current branch:
bash
Replace <commit-hash>
with the identifier of the commit you want to apply.
Stash
What is stash?
The stash
command temporarily saves your work without committing it, allowing you to work on something else without losing your changes.
Basic usage of stash
To save your changes temporarily:
bash
To retrieve the saved changes:
bash
To see the list of stored stashes:
bash
To apply and delete the stash:
bash
Reset
What is reset?
reset
allows you to revert the repository to a previous state, modifying the commit history. There are three reset modes: --soft
, --mixed
, and --hard
.
Basic usage of reset
To reset to the specified commit:
bash
- --soft: Keeps your changes in the staging area.
- --mixed: Keeps your changes in the working directory.
- --hard: Discards all changes in the working directory.
Bisect
What is bisect?
bisect
helps find a specific commit that introduced a bug using a binary search.
Basic usage of bisect
- Start bisect:
bash
- Mark the bad commit:
bash
- Mark the good commit:
bash
Git will automatically search for the commit that introduced the bug.
Practical Examples
Using rebase to update a feature branch
-
Make changes and commits in
feature
:bash -
Update
feature
with the latest changes frommain
:bash
Using cherry-pick to apply a specific commit
- Find and apply a commit from
feature
tomain
:bash
Using stash to save temporary changes
-
Save your temporary changes:
bash -
Retrieve the changes when ready:
bash
Using bisect to find a problematic commit
-
Start the bisect:
bash -
Mark the commits as good or bad until you find the problematic commit.
Best Practices and Tips
- Use interactive rebase to clean up history before merging.
- Do cherry-pick only when you need to apply specific changes, not the entire branch.
- Use stash for temporary changes and keep the working directory clean.
- Use bisect to efficiently find problematic commits.
With these advanced techniques, you can better manage your workflow and resolve complex situations in Git. In the next module, you will learn about automation with Git hooks.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
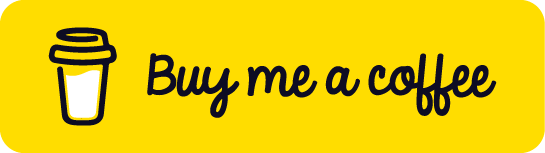
Chat with Chuck
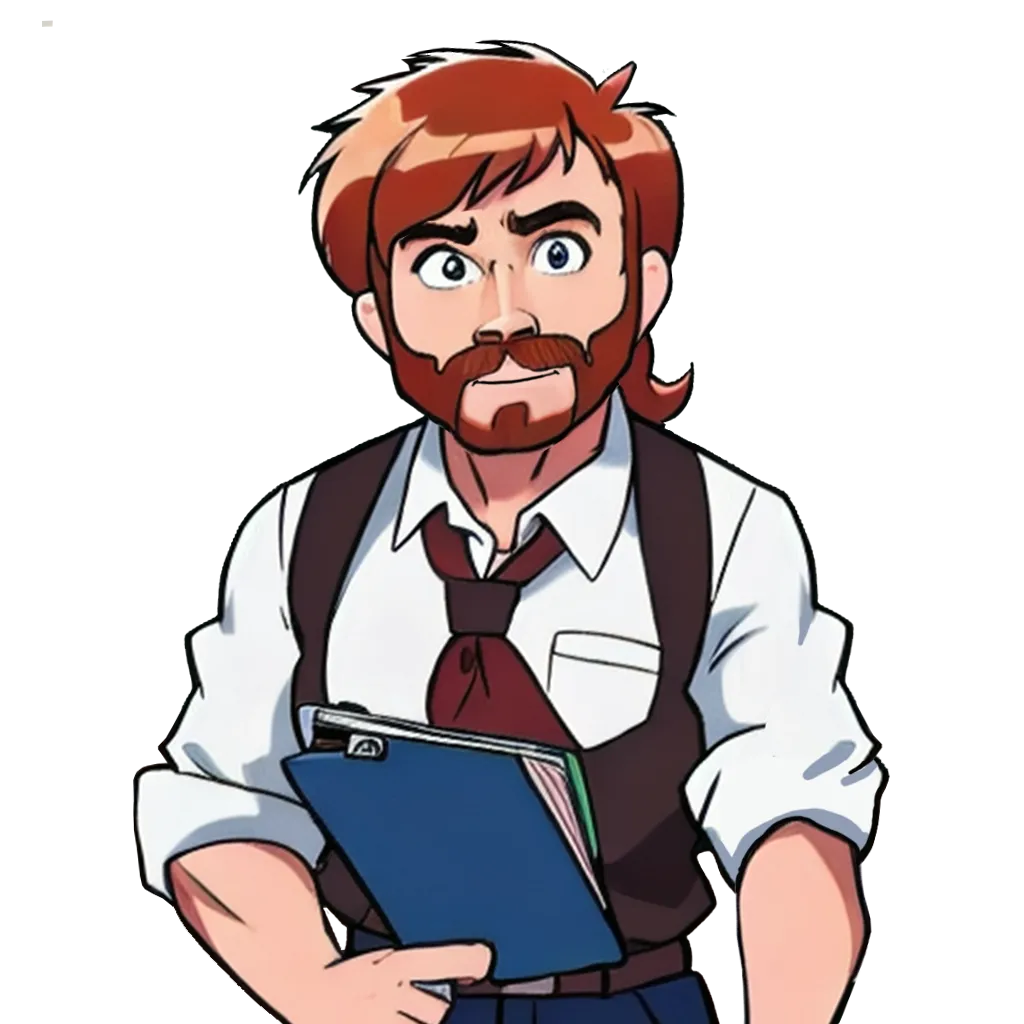
- Introduction to Git and GitHub
- Installation and Configuration of Git
- Version Control Fundamentals
- Repository Creation and Cloning
- Making Commits and Tracking Changes
- Branch Management (branching)
- Branch Merging (Merging)
- Conflict Resolution
- Collaborative Work on GitHub
- Pull Requests and Code Reviews
- Advanced Git Usage (rebase, cherry-pick, etc.)
- Automation with Git hooks
- Continuous Integration with GitHub Actions
- Version Management and Release Deployment
- Conclusions and Best Practices in Git and GitHub