Git and GitHub
Repository Creation and Cloning
In this module, we will learn how to create new repositories and clone existing ones in both Git and GitHub. These are the fundamental initial steps to start working with Git and collaborating using GitHub.
Creating a Local Repository
Step 1: Create a Directory
First, create a new directory for your project:
bash
Step 2: Initialize Git
Inside the new directory, initialize a new Git repository:
bash
This command creates a .git
subdirectory that contains all the necessary files for the repository.
Step 3: Verify Initialization
You can verify that the repository has been initialized correctly with:
bash
Adding Files to the Repository
-
Create a new file:
bash -
Add the file to the staging area:
bash -
Make the first commit:
bash
Creating a GitHub Repository
Step 1: Sign in to GitHub
Log in to your GitHub account. If you don't have an account, create one at GitHub.
Step 2: Create a New Repository
-
Click on the
New
button orNew repository
. -
Complete the required fields:
- Repository name: repository-name
- Description (optional): Brief explanation of the repository.
- Privacy: Choose between a public or private repository.
- Additional options: You can add a README, a
.gitignore
file, or choose a license.
-
Click on
Create repository
.
Step 3: Connect the Local Repository with GitHub
To connect your local repository with the newly created repository on GitHub, use:
bash
Pushing Your Code to the Remote Repository
- Push the commits:
bash
Cloning a Repository
Cloning a repository allows you to get a full copy of a remote repository on your local machine.
Step 1: Get the Repository URL
On the repository page on GitHub, copy the repository URL (HTTPS, SSH, or GitHub CLI).
Step 2: Clone the Repository
Use the git clone
command followed by the repository URL:
bash
Step 3: Navigate to the cloned repository directory
bash
Best Practices
- README.md: Include a README file to provide a project description.
- .gitignore: Add a
.gitignore
file to exclude files and directories you don't want to include in the repository.
Summary of Key Commands
- Initialize a repository:
git init
- Add files to the staging area:
git add .
- Make a commit:
git commit -m "message"
- Add a remote:
git remote add origin URL
- Push changes:
git push -u origin master
- Clone a repository:
git clone URL
In the next module, we will learn how to make commits and track changes in your files using Git.
- Introduction to Git and GitHub
- Installation and Configuration of Git
- Version Control Fundamentals
- Repository Creation and Cloning
- Making Commits and Tracking Changes
- Branch Management (branching)
- Branch Merging (Merging)
- Conflict Resolution
- Collaborative Work on GitHub
- Pull Requests and Code Reviews
- Advanced Git Usage (rebase, cherry-pick, etc.)
- Automation with Git hooks
- Continuous Integration with GitHub Actions
- Version Management and Release Deployment
- Conclusions and Best Practices in Git and GitHub
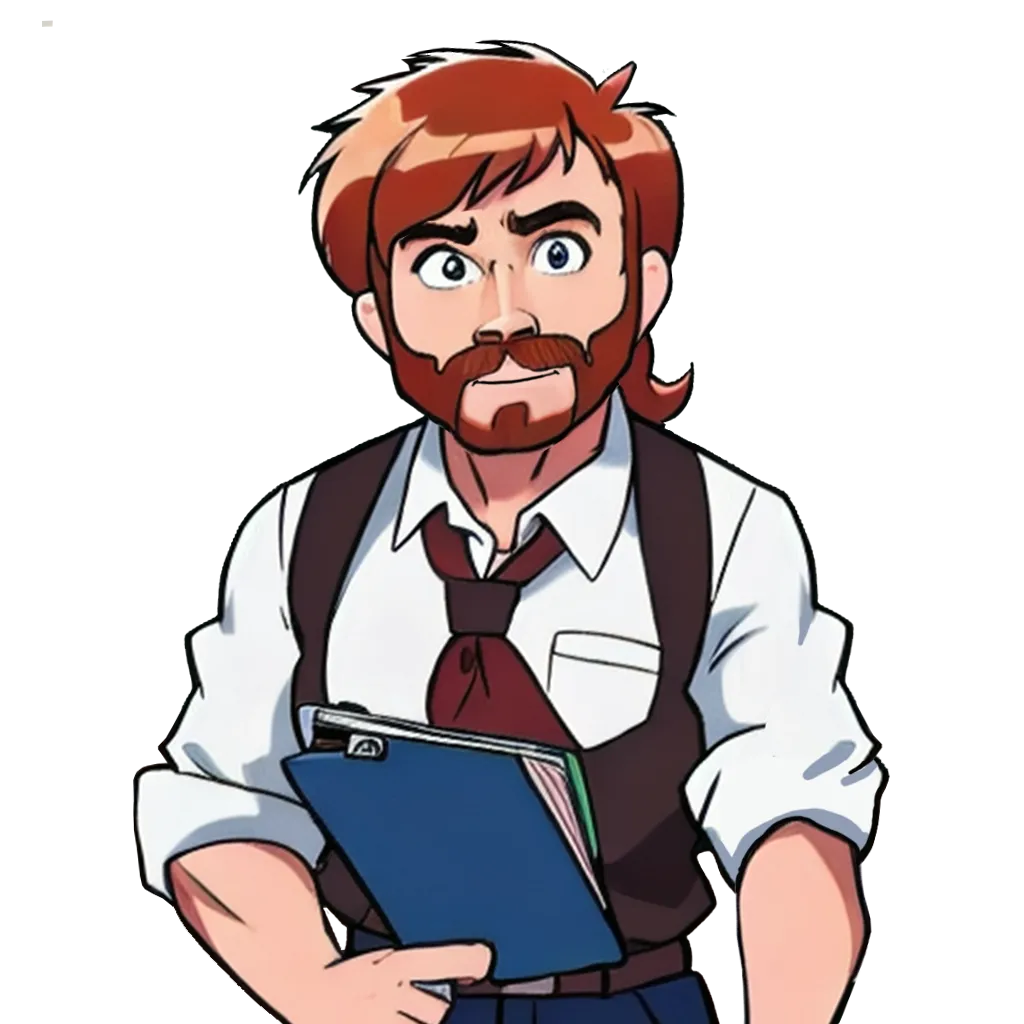