Git and GitHub
Branch Management (branching)
In this module, we'll explore how to manage branches in Git. Branches allow you to develop features, fix bugs, and experiment safely without affecting the main branch of the project. A solid understanding of branches is fundamental to using Git effectively.
What is a branch?
A branch in Git is an independent line of development. The main branch, which is created by default, is usually called main
or master
. Creating new branches allows you to work on different features or bug fixes without interfering with the main branch.
Using branches in Git
Create a new branch
To create a new branch and switch to it immediately, use:
bash
This creates a new branch from the current branch and switches you to that branch.
View existing branches
To list all branches in your repository, use:
bash
The command will show the list of current branches, with an asterisk (*) indicating the active branch.
Switch branches
To switch to an existing branch, use:
bash
Merge branches
To merge changes from one branch into another, first switch to the target branch:
bash
Then merge the desired branch:
bash
Typical workflow with branches
Step 1: Create and switch to a new branch
bash
Step 2: Make changes and commits in the new branch
bash
Step 3: Switch to the main branch and merge changes
bash
Step 4: Delete a branch
If the branch is no longer needed, you can delete it:
bash
Remote branches
Besides local branches, you can also work with remote branches, which exist in your repository on GitHub.
List remote branches
To list remote branches, use:
bash
Track a remote branch
To create a local branch that tracks a remote branch:
bash
Push a branch to the remote repository
To push a branch and create a remote branch, use:
bash
Delete a remote branch
To delete a remote branch, use:
bash
Branching strategies
Gitflow
Gitflow is a branching strategy that divides work into specific branches for development, release, and hotfixes. The main branches in Gitflow are:
main
: Contains the production code.develop
: Contains code ready for the next release.- Feature (
feature
), release (release
), and hotfix (hotfix
) branches.
Trunk-based development
Trunk-based development promotes continuous integration into a single main branch (main
or master
), with short-lived branches for small features or fixes.
Practical examples
-
Create a feature branch:
bash -
Make and commit changes in the new branch:
bash -
Merge changes into the main branch:
bash -
Delete the feature branch:
bash
With these techniques and workflows, you can effectively manage branches in your projects with Git. In the next module, we will learn how to merge branches and resolve conflicts.
- Introduction to Git and GitHub
- Installation and Configuration of Git
- Version Control Fundamentals
- Repository Creation and Cloning
- Making Commits and Tracking Changes
- Branch Management (branching)
- Branch Merging (Merging)
- Conflict Resolution
- Collaborative Work on GitHub
- Pull Requests and Code Reviews
- Advanced Git Usage (rebase, cherry-pick, etc.)
- Automation with Git hooks
- Continuous Integration with GitHub Actions
- Version Management and Release Deployment
- Conclusions and Best Practices in Git and GitHub
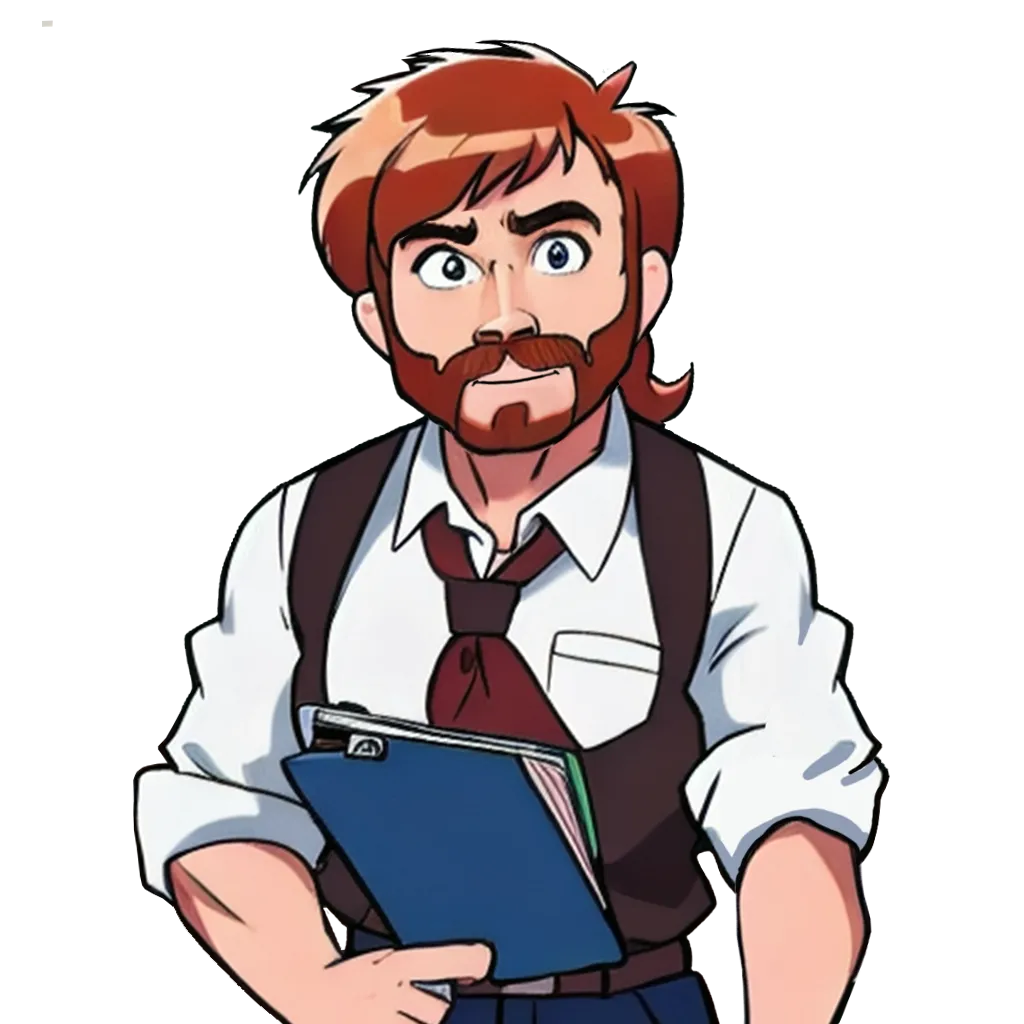