Git and GitHub
Automation with Git hooks
In this module, we will explore Git hooks, which are scripts that Git automatically executes in response to certain repository events. Hooks are extremely useful for automating tasks such as code verification, running tests, and continuous deployment.
What are Git hooks?
Git hooks are scripts that Git automatically executes before or after important events, such as commits, pushes, and merges. There are two main types of hooks:
- Client-side hooks: Affect local operations like commits and merges.
- Server-side hooks: Run on the server during network operations like receiving pushes.
Location of hooks
Hooks are located in the .git/hooks
directory of your repository. Each hook is a script file (usually Shell, Python, Ruby, etc.) and must be executable for Git to run them.
Client-side hooks
Pre-commit
Runs before a commit is made. You can use it to validate the commit message, check code style, or run tests.
Example of pre-commit
that prevents commits with empty messages:
sh
- Create a file named
pre-commit
in the.git/hooks
directory. - Add the above code.
- Make the script executable:
bash
Commit-msg
Runs after the commit message is entered, but before the commit completes. It can be used to validate the commit message format.
Example of commit-msg
that validates that the commit message is not empty:
sh
- Create a file named
commit-msg
in the.git/hooks
directory. - Add the above code.
- Make the script executable:
bash
Server-side hooks
Pre-receive
Runs on the server before accepting a push. Useful for validating changes before accepting them into the remote repository.
Basic example of pre-receive
:
sh
- On the server, create a file named
pre-receive
in thehooks
directory of your remote repository. - Add the above code.
- Make the script executable:
bash
Post-receive
Runs after changes have been accepted on the server. Useful for continuous deployment or notifications.
Example of post-receive
that deploys the code to a web server:
sh
- On the server, create a file named
post-receive
in thehooks
directory of your remote repository. - Add the above code.
- Make the script executable:
bash
Practical examples
Pre-commit hook that checks code style with eslint
- First, install
eslint
in your project:bash - Create the
pre-commit
file in.git/hooks
:sh - Make the script executable:
bash
Post-receive hook to send Slack notifications
- Set up the Slack webhook.
- Create the
post-receive
file in thehooks
directory of the remote repository:sh - Make the script executable:
bash
Best practices
- Keep hooks versioned: Store your hook scripts in the repository (e.g., in a
.githooks
folder) so all collaborators can access them. - Document your hooks: Provide clear instructions on how to install and use the hooks.
- Testing in hooks: Ensure that the scripts in the hooks do not introduce faults in your workflow.
Configuring hooks through core.hooksPath
You can set up a custom directory for hooks:
- Create a folder to store your hooks (e.g.,
.githooks
). - Move your hook scripts to this folder.
- Configure
core.hooksPath
to use this folder:bash
With these techniques and examples, you can start automating repetitive tasks and integrating different tools into your development workflow using Git hooks. In the next module, we will learn about continuous integration with GitHub Actions.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
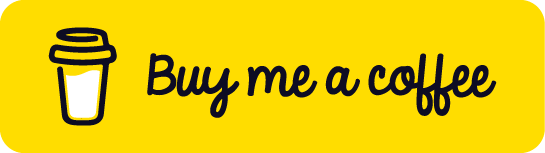
Chat with Chuck
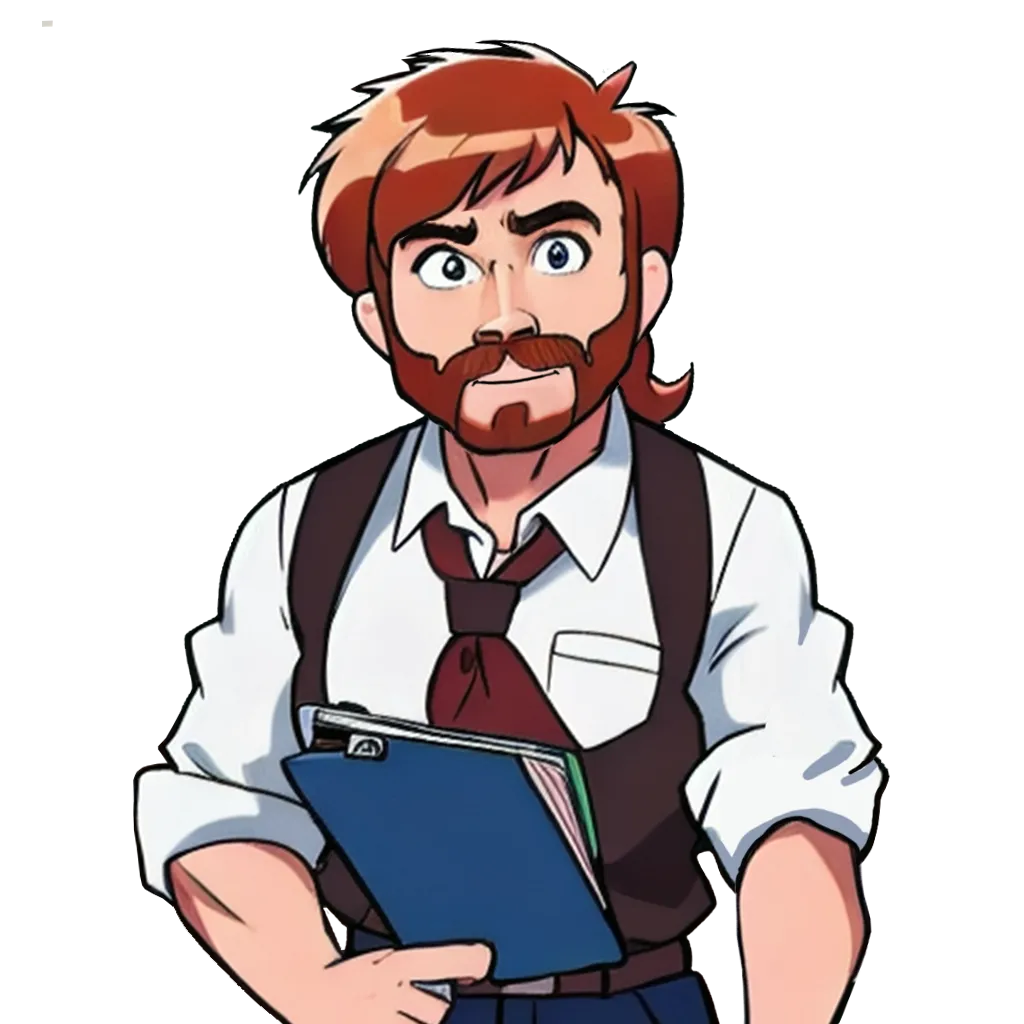
- Introduction to Git and GitHub
- Installation and Configuration of Git
- Version Control Fundamentals
- Repository Creation and Cloning
- Making Commits and Tracking Changes
- Branch Management (branching)
- Branch Merging (Merging)
- Conflict Resolution
- Collaborative Work on GitHub
- Pull Requests and Code Reviews
- Advanced Git Usage (rebase, cherry-pick, etc.)
- Automation with Git hooks
- Continuous Integration with GitHub Actions
- Version Management and Release Deployment
- Conclusions and Best Practices in Git and GitHub