Git and GitHub
Continuous Integration with GitHub Actions
In this module, we will explore how to set up and implement Continuous Integration (CI) using GitHub Actions. GitHub Actions is a CI/CD service integrated into GitHub that allows you to automate workflows such as testing, building, and deploying applications.
What is Continuous Integration (CI)?
Continuous Integration (CI) is a software development practice where developers regularly integrate their code into a shared repository, followed by automatic execution of tests and builds. This helps identify issues early and improves software quality.
Configuring GitHub Actions
Create a workflow file
GitHub Actions workflows are defined in YAML files located in the .github/workflows
directory of your repository. Each file defines a set of actions that run in response to specific events.
Basic workflow example
Let's create a simple example that runs automated tests every time a push is made to the repository.
-
Create the
.github/workflows
directory if it doesn't exist yet:bash -
Create a YAML file named
ci.yml
inside the.github/workflows
directory and add the following:yaml
Workflow Components
name
Defines the name of the workflow. In this example, the workflow is called "CI".
on
Specifies the events that trigger the workflow. Here it runs on every push
and pull_request
to the main
branch.
jobs
A collection of jobs to be run in this workflow. Each job includes steps that define specific tasks:
runs-on
: The environment in which the job will run (e.g.,ubuntu-latest
).steps
: The actions to execute.
Reusing Actions
Use community actions
GitHub Actions has a vast marketplace with actions you can reuse. For example, to run linters, builds, and even deployments.
Example of using a linting action:
yaml
Variables and Secrets
Using secrets
You can store sensitive information (like API tokens) in GitHub secrets and access them in your workflows.
- Navigate to your repository on GitHub.
- Go to "Settings" -> "Secrets".
- Add a new secret and assign it a name (for example,
API_TOKEN
).
Then, use the secret in your YAML file:
yaml
Practical Example of a Complete Workflow
Setting up a CI pipeline for a Node.js application
Suppose we have a Node.js application and want to set up a CI pipeline that:
-
Installs dependencies.
-
Runs tests.
-
Lints the code.
-
Builds the application.
-
Create the YAML file in
.github/workflows/ci.yml
:yaml
Continuous Deployment
Deploying to Heroku
Suppose you want to automatically deploy your application to Heroku every time there is a change in main
.
- Add the secret
HEROKU_API_KEY
in the GitHub secrets configuration. - Configure the workflow to include the deployment step:
yaml
Best Practices
- Keep your workflows simple and specific: One YAML file for each set of related tasks.
- Use secrets for sensitive information.
- Leverage community actions: Reduce redundancy by using pre-existing actions in the marketplace.
- Divide and conquer: Break down complex tasks into more manageable, understandable steps.
With these techniques, you can set up a robust CI/CD pipeline using GitHub Actions to improve the quality and efficiency of your software development. In the next module, we will learn about version management and release deployments.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
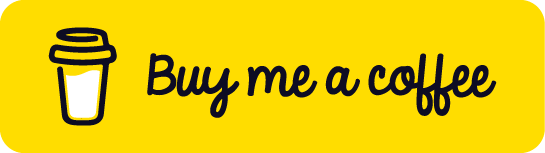
Chat with Chuck
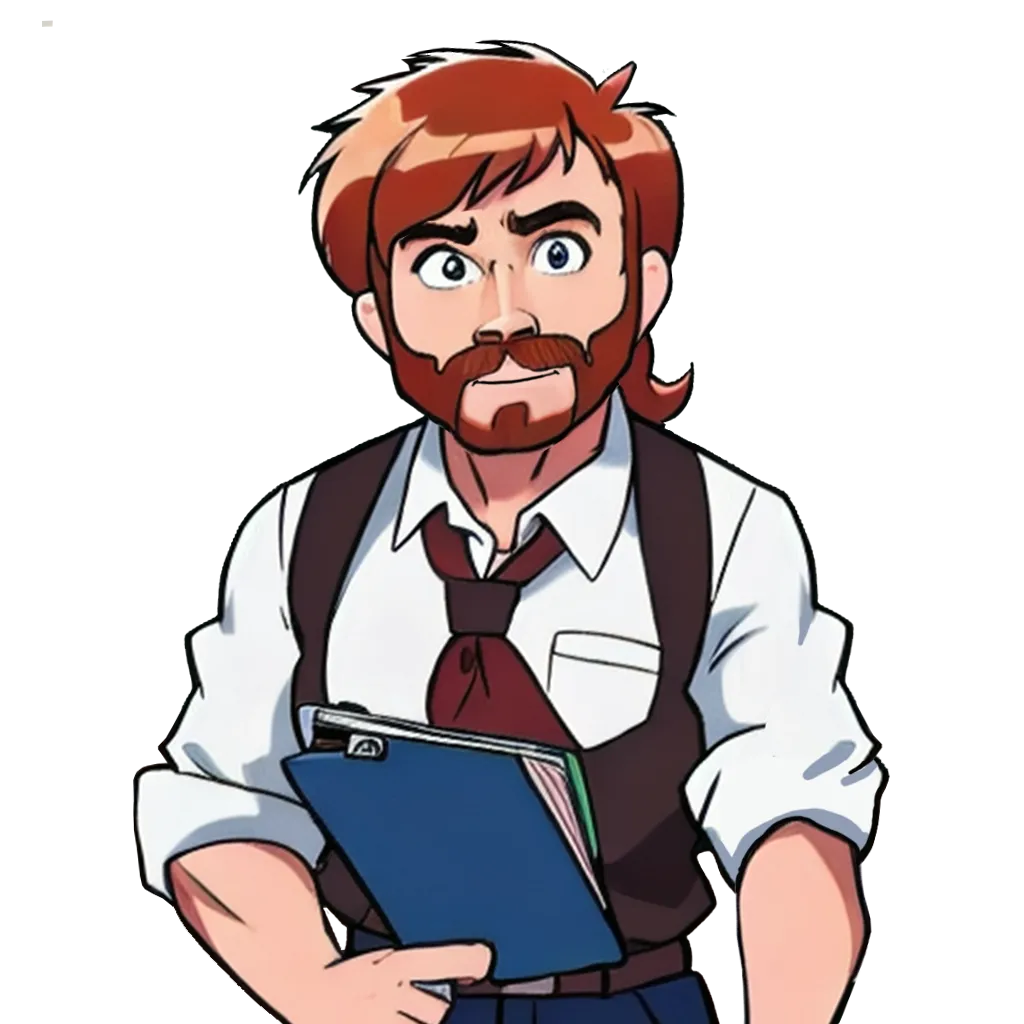
- Introduction to Git and GitHub
- Installation and Configuration of Git
- Version Control Fundamentals
- Repository Creation and Cloning
- Making Commits and Tracking Changes
- Branch Management (branching)
- Branch Merging (Merging)
- Conflict Resolution
- Collaborative Work on GitHub
- Pull Requests and Code Reviews
- Advanced Git Usage (rebase, cherry-pick, etc.)
- Automation with Git hooks
- Continuous Integration with GitHub Actions
- Version Management and Release Deployment
- Conclusions and Best Practices in Git and GitHub