HTML Forms
Validation and Constraints in HTML Forms
Form validation is a fundamental part of ensuring that the data entered by the user is correct and in the expected format. HTML offers various tools to perform automatic validations without the need for JavaScript. In this chapter, we will address native HTML validation and the constraints we can apply to form fields.
Native Browser Validation
Modern browsers include form validation by default. If the form contains fields with attributes like required
, pattern
, min
, max
, among others, the browser will prevent the form from being submitted if the entered data does not meet the specified requirements.
html
Custom Validation Messages
When the data does not meet the validation requirements, the browser displays default error messages. However, we can customize these messages using the title
attribute, which specifies a message that appears when the field is invalid.
html
Pattern Validation with Regular Expressions
The pattern
attribute allows you to define a specific format for a field using a regular expression. This is useful for data like phone numbers, zip codes, or any other input with a predefined format.
html
Range Validation for Numeric Fields
For fields of type number
, date
, time
, and others, the min
, max
, and step
attributes allow control over the range and increments of values. These attributes can help prevent errors in entering numeric or date data.
html
Date and Time Constraints
Fields of type date
, time
, and datetime-local
allow setting date and time constraints using min
and max
. These attributes ensure that users enter dates within a specific range.
html
Blocking Automatic Submission: Validation with JavaScript
If you need custom validation or want to handle the form's behavior in detail, you can use JavaScript to intercept the form submission. By capturing the submit
event, you can verify each field and display specific messages when the form does not meet the requirements.
html
Complete Form Validation Example
To consolidate what we've learned, let's look at a full example that includes multiple types of validation in a form.
html
Chapter Closing
In this chapter, we explored the various options for validation and constraints in HTML forms, allowing us to ensure that the data entered by users is correct and meets the expected formats. In the next chapter, we will delve into advanced form elements, such as file uploads and the use of autocomplete lists, which extend functionality and enhance the user experience in more complex forms.
- Introduction to HTML Forms
- HTML Form Elements
- Attributes and Controls in HTML Forms
- Validation and Constraints in HTML Forms
- Advanced HTML Form Elements
- Form Submission and Data Handling
- Styling HTML Forms with CSS
- Best Security Practices in HTML Forms
- Interactive and Dynamic Forms
- Best Practices and Common Errors in HTML Forms
- Conclusion of the HTML Forms Course
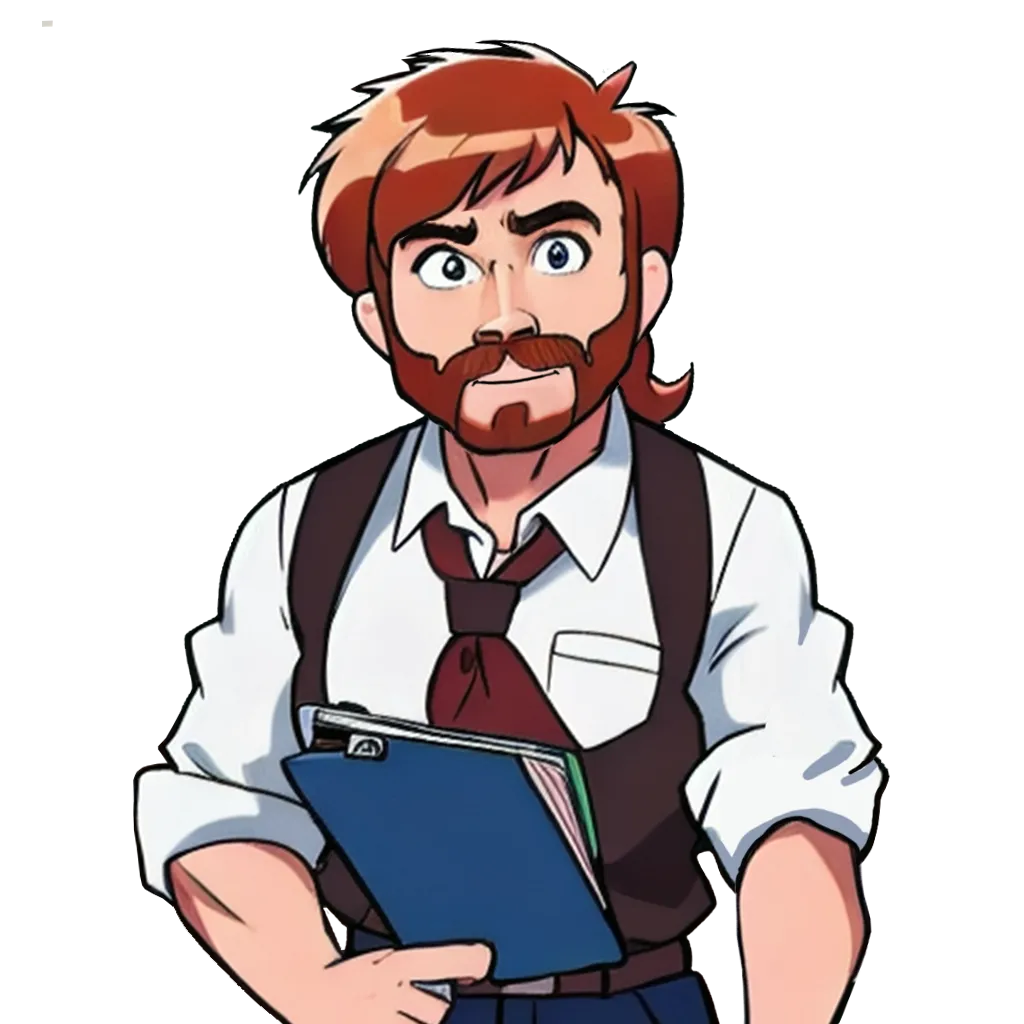