Middlewares in Node
Advanced Middleware Optimization
Advanced Middleware Optimization
In large-scale applications, optimizing middlewares can significantly improve performance and efficiency. In this module, we will discuss various advanced techniques for optimizing middlewares in Node.js, including the use of conditional middlewares, asynchronous middlewares, and middleware grouping.
Conditional Middlewares
Conditional middlewares allow you to execute middlewares only under certain conditions, which can improve performance by avoiding unnecessary executions.
Example: Conditional Middleware
Suppose you want to apply a logging middleware only to GET requests.
javascript
In this example, the conditionalLogging
middleware logs the request only if it is a GET method, avoiding unnecessary execution for other HTTP methods.
Asynchronous Middlewares
Asynchronous middlewares allow handling asynchronous operations, such as database calls or external API requests, without blocking the execution thread.
Example: Asynchronous Middleware
Let's create a middleware that simulates token verification in a database asynchronously.
javascript
In this example, the verifyToken
middleware performs an asynchronous verification and proceeds only if the token is valid.
Middleware Grouping
Grouping related middlewares can improve the organization and performance of your application. You can use express.Router
to group middlewares and routes that share common logic.
Example: Middleware Grouping
Let's group middlewares and routes related to authentication and authorization.
javascript
In this example, we have grouped authentication and authorization rules under the router for the /private
route, simplifying the structure and improving middleware management.
[Insert image here: Diagram showing how conditional, asynchronous, and grouped middlewares optimize the request flow in a Node.js application.]
Optimizing your middlewares not only improves the performance of your application but also its maintainability and scalability. In the next module, we will discuss how to use middlewares to enhance the security of your applications.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
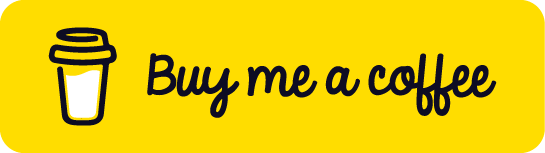
Chat with Chuck
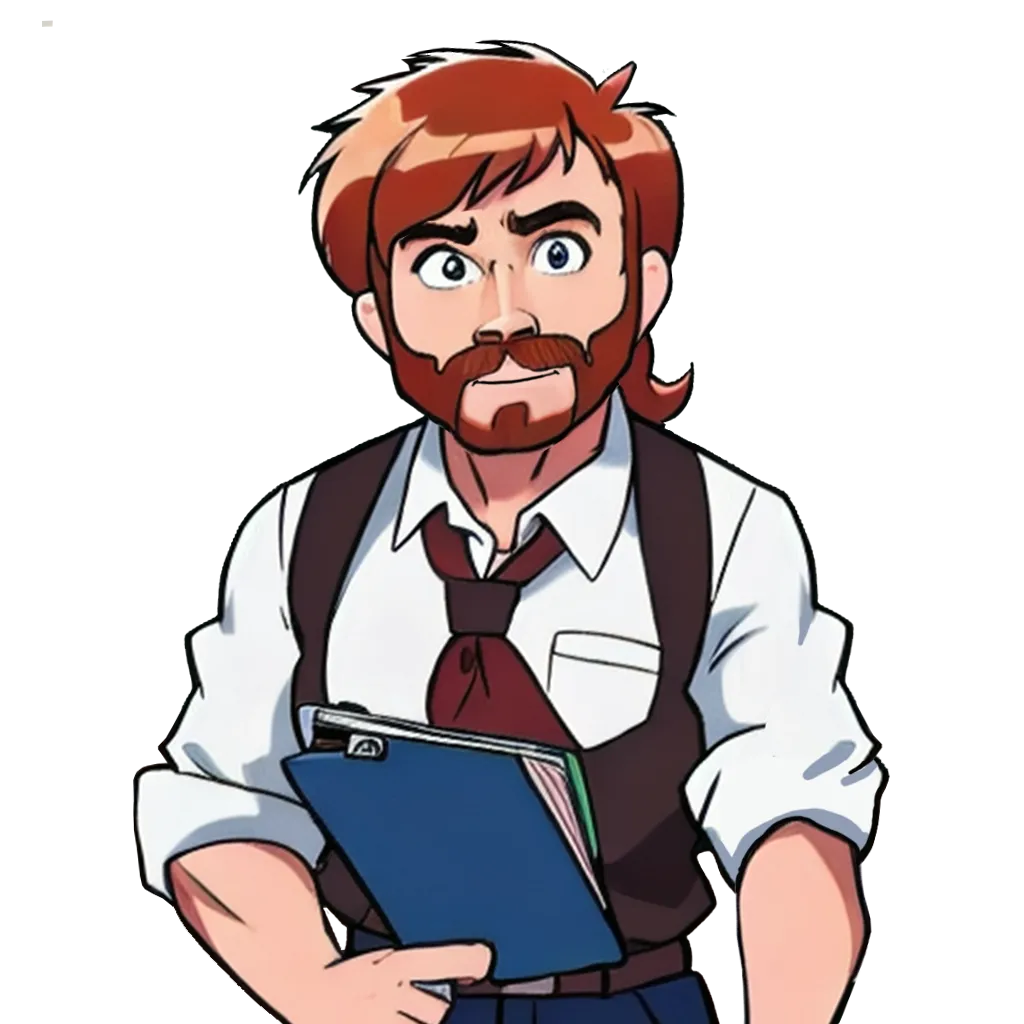
- Introduction to Middlewares in Node.js
- Types of Middleware in Node.js
- Creating Custom Middlewares
- Error Handling Middlewares
- Middlewares for Logging
- Middlewares for Authentication and Authorization
- Sensitive Data and Configuration Handling
- Advanced Middleware Optimization
- Security Middlewares
- Testing and Debugging Middlewares
- Best Practices for Working with Middlewares
- Conclusion of the Middleware Course in Node.js