Middlewares in Node
Sensitive Data and Configuration Handling
Sensitive Data and Configuration Handling
In any application, it is essential to handle sensitive data and configurations in a secure and efficient manner. These can include API keys, access tokens, database credentials, server configurations, and more. In this module, you will learn how to use middlewares to manage sensitive data and configurations in Node.js.
Environment Variables
A common practice is to use environment variables to manage sensitive data and configurations. Node.js can access environment variables through the process.env
object.
Setting Up Environment Variables
Use a .env
file to store configurations and sensitive data. Use the dotenv
library to load these configurations into process.env
at the start of the application.
Installation
First, install dotenv
:
sh
Example of a .env
File
env
Loading Environment Variables
Use dotenv
to load the environment variables at the start of your application:
javascript
In this example, the configuration data and database credentials are loaded from environment variables and used in the application.
Middleware for Configuration Validation
It is good practice to validate that all necessary environment variables are defined at the start of the application. This can be done through a middleware.
Example: Configuration Validation Middleware
javascript
In this example, the validateConfig
middleware checks that all necessary environment variables are defined. If any are missing, it throws an error and stops the application.
Secure Storage of Sensitive Data
It is crucial to store sensitive data securely and make it accessible only as needed. Avoid including sensitive data in source code or places where it can be exposed.
Example: Using a Secret Management Service
For more secure handling of secrets and credentials, you can use services like AWS Secrets Manager, HashiCorp Vault, or Azure Key Vault. Below is a basic example simulating the use of a secret management service.
javascript
In this example, a middleware loads secrets from a simulated service and adds them to the request object, making them accessible throughout the application.
[Insert image here: Diagram showing how environment variables and secret management services integrate into the application flow to provide sensitive data and configurations securely.]
Managing sensitive data and configurations securely is essential for the integrity and security of your application. In the next module, we will explore advanced techniques for middleware optimization.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
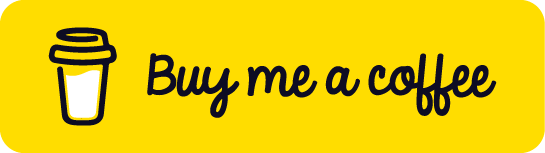
Chat with Chuck
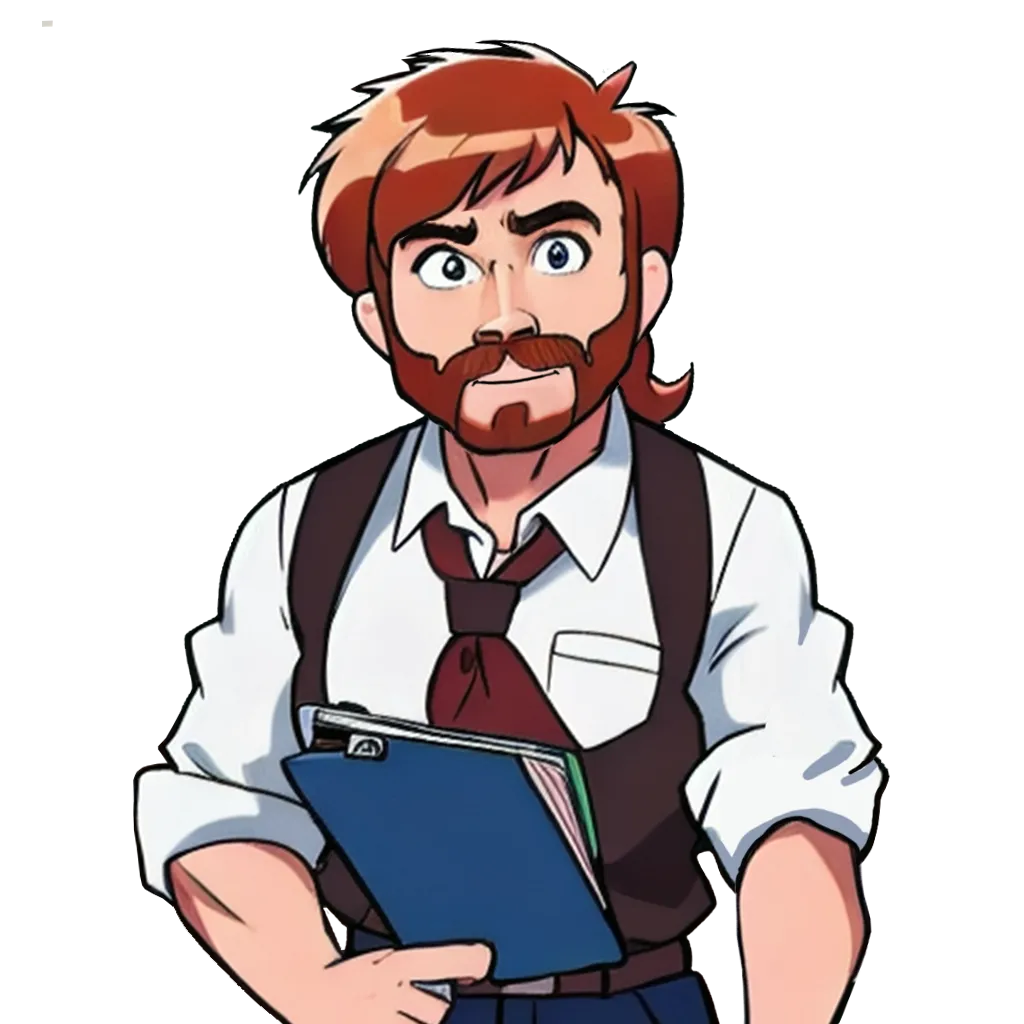
- Introduction to Middlewares in Node.js
- Types of Middleware in Node.js
- Creating Custom Middlewares
- Error Handling Middlewares
- Middlewares for Logging
- Middlewares for Authentication and Authorization
- Sensitive Data and Configuration Handling
- Advanced Middleware Optimization
- Security Middlewares
- Testing and Debugging Middlewares
- Best Practices for Working with Middlewares
- Conclusion of the Middleware Course in Node.js