Middlewares in Node
Error Handling Middlewares
Error Handling Middlewares
Error handling is a crucial part of any web application. Node.js and Express make it easy to create error-handling middlewares that can intercept, manage, and respond to errors centrally. In this module, you will learn how to configure and use middlewares to handle errors efficiently.
What is an Error Handling Middleware?
An error-handling middleware is similar to any other middleware but is distinguished by having four parameters in its function: err
, req
, res
, and next
. Having the err
parameter at the beginning indicates to Express that this function is specifically for error handling.
Basic Structure
javascript
Capturing Errors in Routes
To capture an error in a route and pass it to the error-handling middleware, use the next
function, passing the error as an argument.
Example
javascript
In this example, any request to the /error
route will generate an intentional error and pass it to the error-handling middleware, which will then log the error and send a 500 status response.
Different Types of Errors
It's possible to handle different types of errors specifically. For example, validation errors, authentication errors, database connection errors, etc., can be handled differently based on their characteristics.
Example: Differentiated Error Handling
javascript
In this example, validation errors send a response with a 400 status, and authentication errors send a response with a 401 status. Other errors are handled generically.
[Insert image here: Diagram showing the flow of a request that encounters an error and how it is passed through the error-handling middleware.]
Handling errors correctly ensures a smoother experience for your users and a simpler way for you, as a developer, to debug and resolve issues. In the next module, we will discuss the utility and methods of logging in middlewares to improve the observability of your application.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
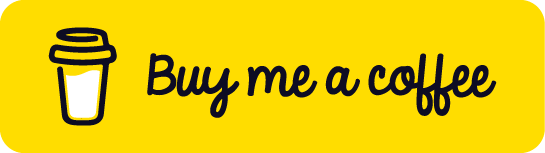
Chat with Chuck
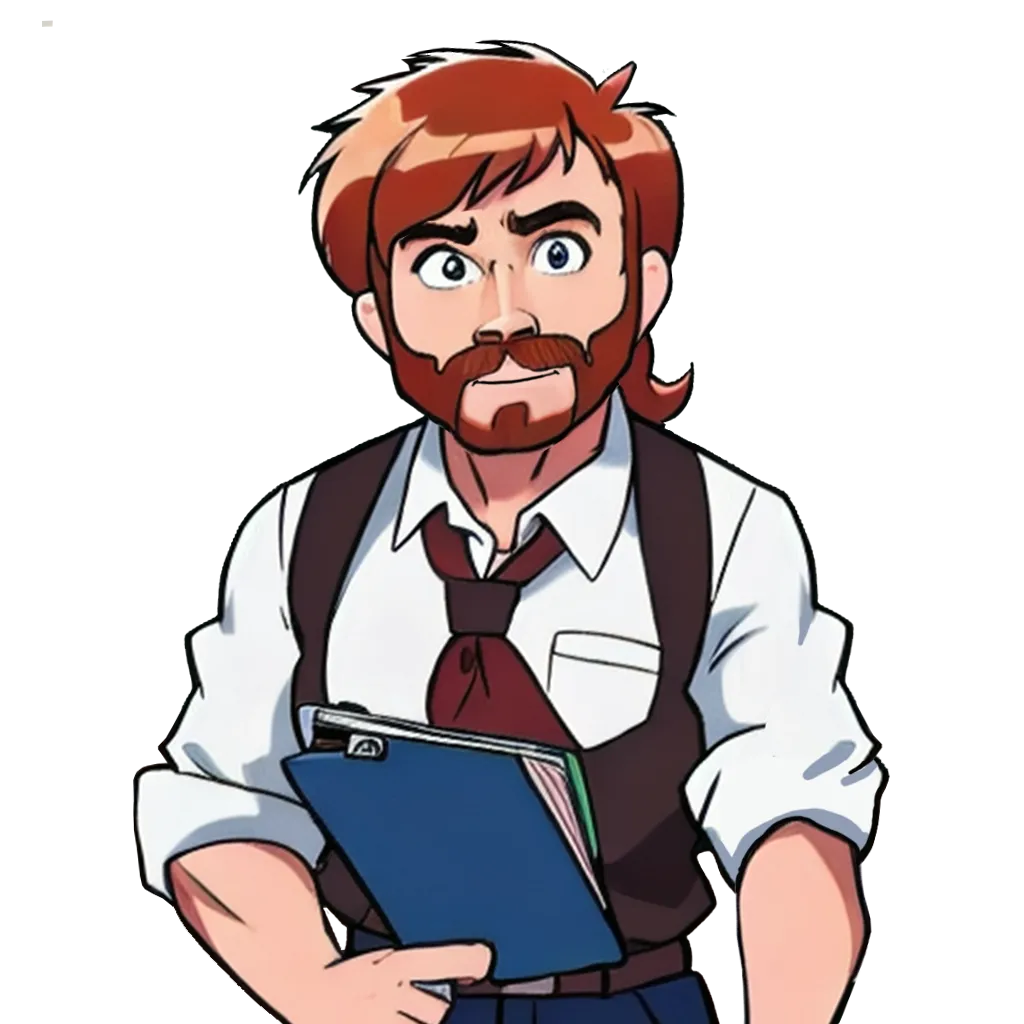
- Introduction to Middlewares in Node.js
- Types of Middleware in Node.js
- Creating Custom Middlewares
- Error Handling Middlewares
- Middlewares for Logging
- Middlewares for Authentication and Authorization
- Sensitive Data and Configuration Handling
- Advanced Middleware Optimization
- Security Middlewares
- Testing and Debugging Middlewares
- Best Practices for Working with Middlewares
- Conclusion of the Middleware Course in Node.js