Middlewares in Node
Security Middlewares
Security Middlewares
Security is one of the major concerns when developing web applications. Node.js and Express provide tools and techniques to protect your application against common threats. In this module, we will discuss how to use middlewares to enhance your application's security.
Basic Security with helmet
helmet
is a collection of middlewares that helps protect your application from some common web vulnerabilities by properly setting HTTP headers.
Installation
First, install helmet
using npm:
sh
Basic Usage
Next, we configure helmet
to improve the security of our application.
javascript
In this example, the helmet
middleware applies a series of standard protections by securely setting HTTP headers.
Preventing XSS Attacks
Cross-site scripting (XSS) attacks are a common vulnerability in web applications. xss-clean
is a library that can help prevent these attacks by sanitizing input data:
Installation
First, install xss-clean
using npm:
sh
Basic Usage
Next, we configure xss-clean
to protect our application from XSS attacks.
javascript
In this example, the xss
middleware sanitizes the input data, preventing potential XSS attacks.
SQL Injection Protection
SQL injection attacks are another common threat. Although database connection libraries often provide mechanisms to prevent these injections, a middleware can add an additional layer of protection by validating and sanitizing input data.
Example: SQL Injection Protection Middleware
javascript
In this example, the sanitize
middleware sanitizes the input data to prevent potential SQL injections.
CORS Configuration
Using CORS (Cross-Origin Resource Sharing) is essential to allow or restrict requests from different domains. cors
is a library that allows you to configure these rules easily.
Installation
First, install cors
using npm:
sh
Basic Usage
Allowing CORS requests from any domain (not recommended in production):
javascript
Advanced Configuration
Restricting allowed origins:
javascript
In this example, only requests from the domain https://my-secure-domain.com
are allowed.
[Insert Image Here: Diagram illustrating the application of various security middlewares in the lifecycle of a request in a Node.js application.]
Implementing these security middlewares is crucial to protecting your application against multiple types of attacks and vulnerabilities. In the next module, we'll discuss how to test and debug your middlewares to ensure they function correctly.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
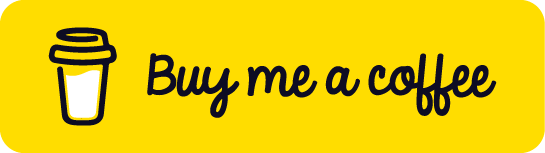
Chat with Chuck
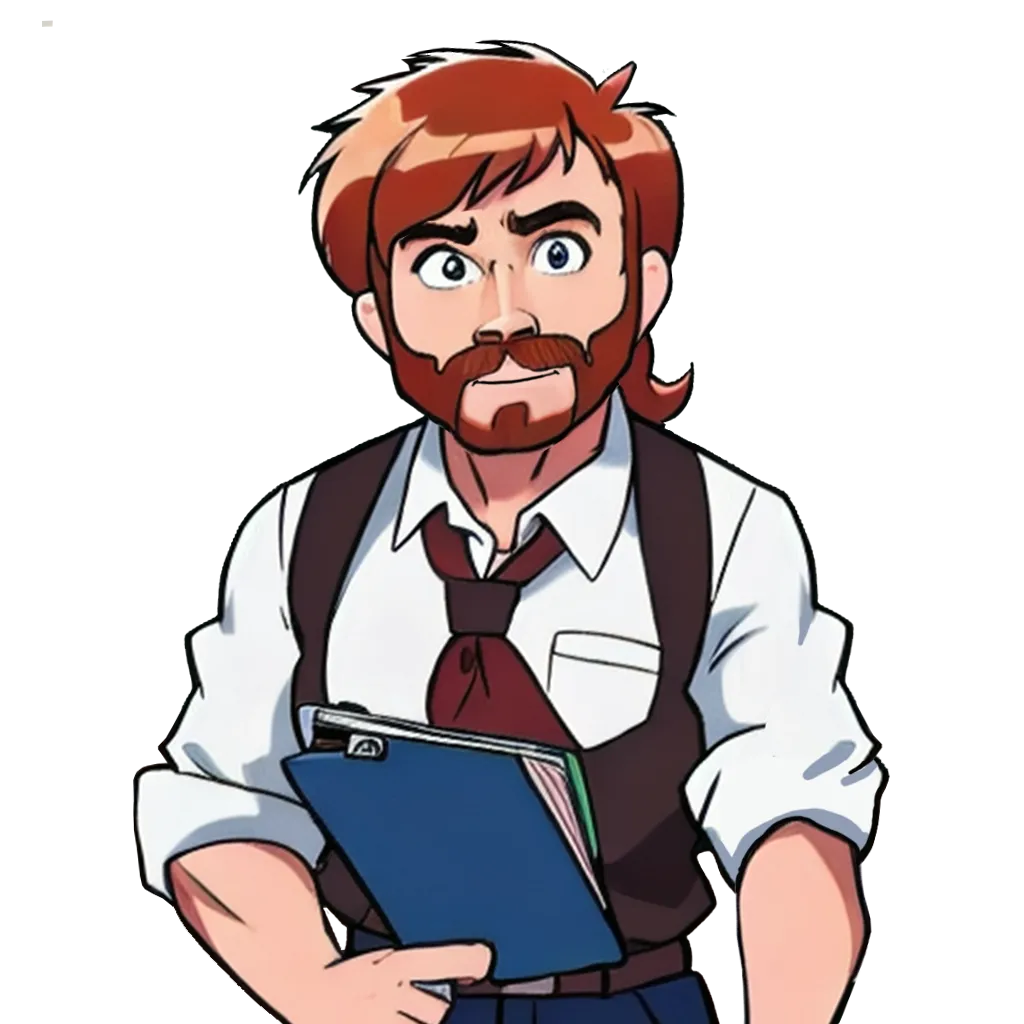
- Introduction to Middlewares in Node.js
- Types of Middleware in Node.js
- Creating Custom Middlewares
- Error Handling Middlewares
- Middlewares for Logging
- Middlewares for Authentication and Authorization
- Sensitive Data and Configuration Handling
- Advanced Middleware Optimization
- Security Middlewares
- Testing and Debugging Middlewares
- Best Practices for Working with Middlewares
- Conclusion of the Middleware Course in Node.js