Middlewares in Node
Testing and Debugging Middlewares
Testing and Debugging Middlewares
The development of effective middlewares not only involves their implementation but also their proper testing and debugging. Testing and debugging your middlewares ensure they work as expected and help identify and fix errors. In this module, you will learn how to test and debug middlewares in Node.js.
Unit Testing with mocha
and chai
Unit tests are crucial for verifying that each piece of your middleware works correctly in isolation. mocha
and chai
are popular libraries for testing in Node.js.
Installation
First, install mocha
and chai
using npm:
sh
Basic Configuration
Next, we set up a test file to test a simple middleware.
Middleware File (middleware.js
)
javascript
Test File (test.js
)
javascript
To run the tests, add a script to your package.json
:
json
Finally, run the tests with:
sh
Functional Tests with supertest
supertest
is another useful tool for testing routes and middlewares in Express applications.
Installation
First, install supertest
using npm:
sh
Functional Test Example
Test File (test-functional.js
)
javascript
Debugging Middlewares
The debugging process helps identify and fix problems in your middleware. Node.js offers several tools for debugging your code.
Using console.log
Although simple, console.log
is one of the most effective ways to debug issues. You can add console.log
statements at various points to track values and the execution flow.
Using node inspect
For more advanced debugging, you can use the node inspect
command to start an interactive debugging session.
sh
Then, open chrome://inspect
in Chrome to begin investigating.
Debugging with vscode
Visual Studio Code offers a powerful integrated debugging tool.
- Add a debug configuration in
.vscode/launch.json
:
json
- Click on the debug icon in the sidebar and select
Launch Program
. - Use breakpoints to pause execution and inspect the application state.
Insert image here: Screenshot of a debugging session in Visual Studio Code, with breakpoints set in the middleware.
Thoroughly testing and debugging your middlewares ensures the quality and robustness of your application. In the next module, we will discuss some best practices and final tips for working with middlewares in Node.js.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
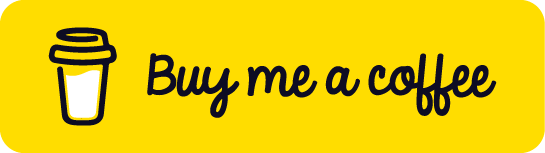
Chat with Chuck
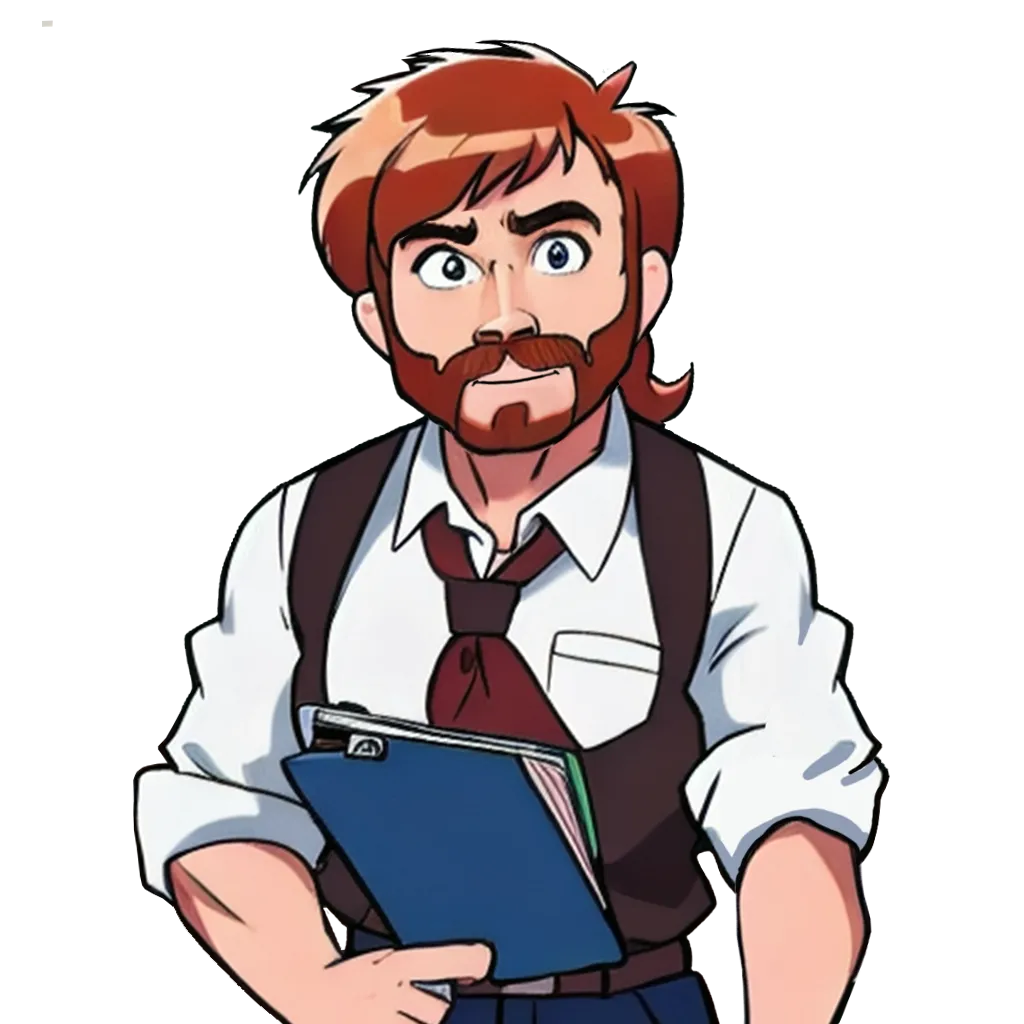
- Introduction to Middlewares in Node.js
- Types of Middleware in Node.js
- Creating Custom Middlewares
- Error Handling Middlewares
- Middlewares for Logging
- Middlewares for Authentication and Authorization
- Sensitive Data and Configuration Handling
- Advanced Middleware Optimization
- Security Middlewares
- Testing and Debugging Middlewares
- Best Practices for Working with Middlewares
- Conclusion of the Middleware Course in Node.js