Middlewares in Node
Best Practices for Working with Middlewares
Best Practices for Working with Middlewares
Working with middlewares in Node.js can simplify and modularize your application if you follow a set of best practices. These practices not only improve code quality but also make it easier to maintain and scale. In this module, we will cover several best practices you should consider when working with middlewares.
Divide and Conquer
Avoid making your middlewares monolithic blocks of code. Split the logic into smaller, specific middlewares that perform a single task. This makes the code easier to understand, test, and maintain.
Example
Instead of having a middleware that both authenticates and logs requests, divide these responsibilities into two separate middlewares:
javascript
Error Handling
Always handle errors in a centralized and consistent manner. Use error-handling middlewares to catch and manage any errors that occur in the previous middlewares or routes.
Example
javascript
Log Middleware Flows
Implement detailed logging to track the flow through your middlewares. This can be invaluable for debugging and understanding how requests are processed by your application.
Example
javascript
Middleware Reusability
Leverage the modularity of middlewares to reuse common logic in different parts of your application. Use functions or external packages for middlewares that can be reused across multiple projects.
Example
Create an NPM package for your custom middleware:
javascript
Then install and use it in different projects:
sh
javascript
Document Your Middlewares
Maintain clear and concise documentation of what each middleware does, its dependencies, and how it should be used. This is essential for facilitating maintenance and team collaboration.
Example
You can use comments in the code or generate documentation using tools like JSDoc.
javascript
Chain Middlewares Efficiently
The order in which you apply the middlewares matters. Make sure middlewares are chained in the correct order to avoid unexpected behaviors.
Example
First, apply global middlewares, then router-specific middlewares, and finally error-handling middlewares:
javascript
[Insert image here: Diagram showing a chain of middlewares applied in the correct order.]
These best practices will help you create more efficient and maintainable middlewares. In the next module, we will conclude the course with a summary of what we have learned and some final thoughts.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
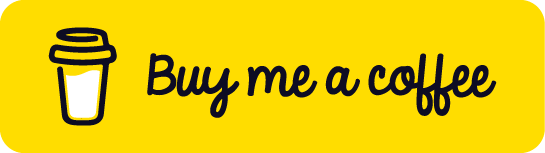
Chat with Chuck
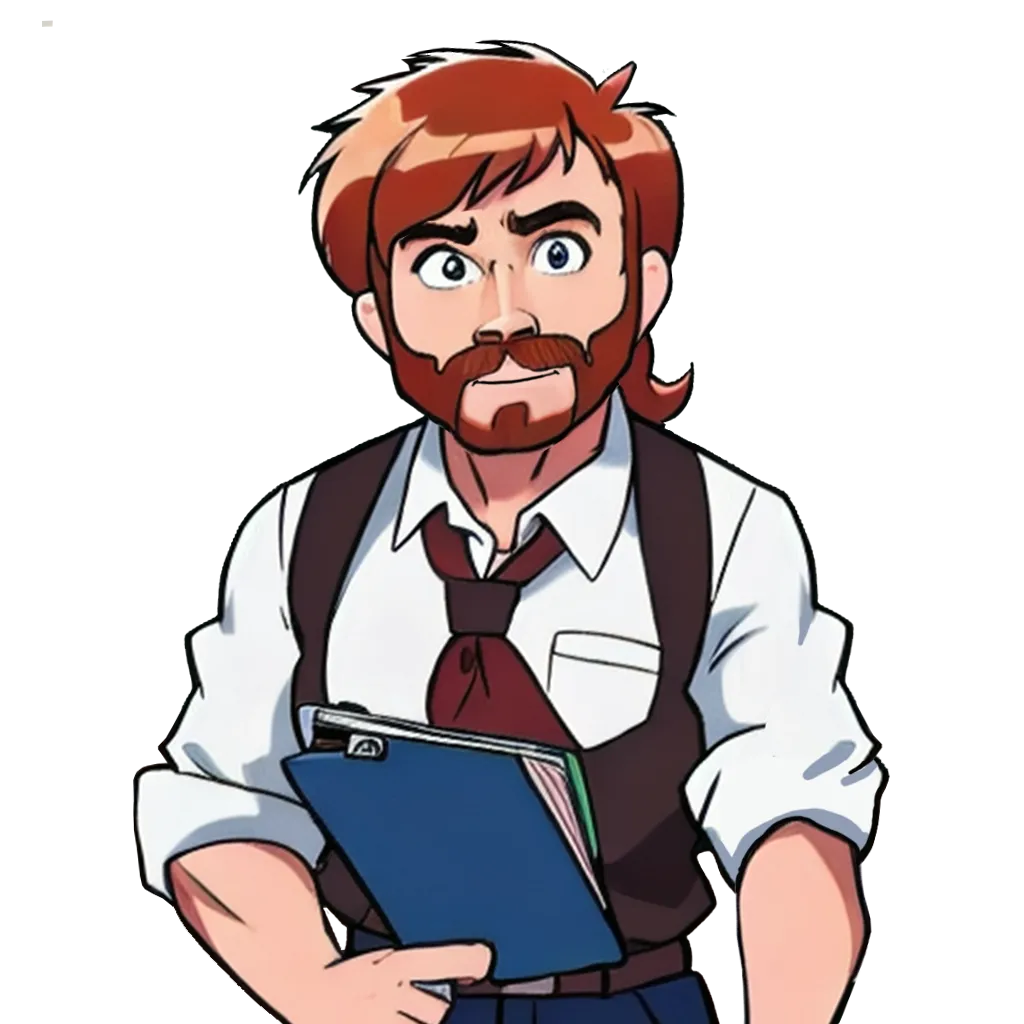
- Introduction to Middlewares in Node.js
- Types of Middleware in Node.js
- Creating Custom Middlewares
- Error Handling Middlewares
- Middlewares for Logging
- Middlewares for Authentication and Authorization
- Sensitive Data and Configuration Handling
- Advanced Middleware Optimization
- Security Middlewares
- Testing and Debugging Middlewares
- Best Practices for Working with Middlewares
- Conclusion of the Middleware Course in Node.js