Testing DOM with Mocha
Writing Your First Unit Tests with Mocha
Writing unit tests is an essential part of quality software development. In this chapter, we will learn how to write our first unit tests using Mocha. We will explore the basic concepts and look at practical examples.
What is a Unit Test?
A unit test is a form of validation that focuses on small units of code, which are usually specific functions or methods. The goal is to ensure that each unit of code behaves as expected in various scenarios.
Basic Structure of a Test in Mocha
Mocha provides a clear and concise structure for defining and grouping tests. The key components are:
- describe(): Groups multiple related tests.
- it(): Defines an individual test.
- assert: Assertion library (you can use others like Chai).
Here is a basic outline of what a test looks like in Mocha:
javascript
Writing Your First Unit Test
Consider a JavaScript file calculator.js
with a simple function:
javascript
Let's write a unit test for this function.
Set Up the Test File
Create a test file called calculator.test.js
in your test
folder:
javascript
Run the Test
Run the test using the command:
sh
You should see output indicating that both tests have passed, similar to this:
More Examples of Unit Tests
Testing a Function with Conditional Behavior
Suppose we want to add a new function that only sums positive numbers:
javascript
We can write a unit test to ensure this function behaves correctly:
javascript
Testing Asynchronous Functions
To test asynchronous functions, you can use done
to indicate the end of a test:
javascript
javascript
Tests with Assertion Libraries
Mocha is compatible with various assertion libraries like Chai. Later, we will see in detail how to combine them to improve the readability and functionality of your tests.
Conclusion
You now have a basic understanding of how to write unit tests with Mocha. In the following chapters, we will delve into the combined use of Mocha with other tools and advanced techniques to further enrich your tests.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
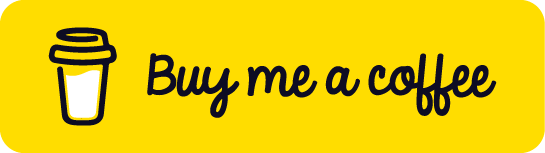
Chat with Chuck
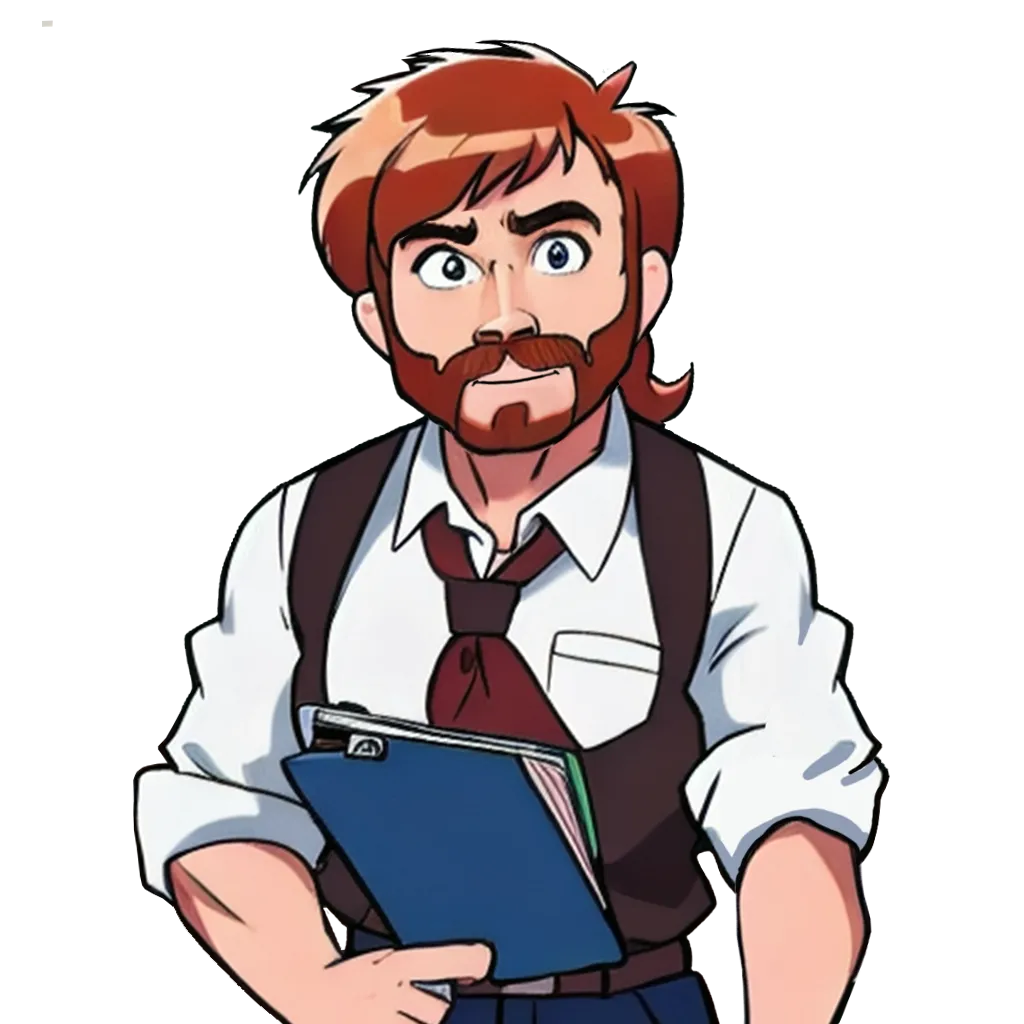
- Introduction to Testing in JavaScript with Mocha
- Fundamentals of the DOM
- Installation and Configuration of Mocha
- Writing Your First Unit Tests with Mocha
- Testing with Mocha and Chai
- DOM Component Testing with Mocha
- DOM Event Testing with Mocha
- Mocking and Stubbing with Sinon in Mocha
- User Interaction Testing with Mocha
- Accessibility Testing with Mocha
- Asynchronous Testing with Mocha
- Organization and Structure of Tests in Mocha
- Automatización de pruebas con CI/CD usando Mocha
- Best Practices for Testing with Mocha
- Conclusions and Next Steps in Testing with Mocha