Testing DOM with Mocha
Best Practices for Testing with Mocha
Adopting best practices in writing and organizing tests with Mocha can lead to greater efficiency, readability, and maintainability in your test suite. In this chapter, we will discuss some of the best practices to ensure that your tests are robust and easy to maintain over time.
Writing Clear and Concise Tests
Use Descriptive Names
Use clear and descriptive names for both describe
blocks and it
test cases. This allows anyone reading the tests to easily understand what is being tested.
javascript
Clear Test Structure (Given-When-Then)
Follow the Given-When-Then structure:
- Given: the initial context or system state
- When: the action being tested
- Then: the expected outcome
javascript
Test Isolation
Each test should be isolated and not depend on the state of other tests. This ensures that tests are independent and easier to debug.
Use Hooks for Setup and Teardown
Use hooks such as before
, beforeEach
, after
, and afterEach
to set up and tear down state before and after each test.
javascript
Placeholder for illustrative image on the use of hooks in tests: Diagram showing the execution sequence of before, beforeEach, afterEach, and after in a test cycle
Use of Mocks and Stubs
Use libraries like sinon
to create mocks and stubs. This helps isolate the system under test and simulate the behavior of external dependencies.
Mock Example with Sinon
javascript
Run Tests in Multiple Environments
Ensure that your tests run in multiple versions of Node.js or browser environments to guarantee compatibility. This can be easily configured in CI/CD tools like GitHub Actions and Jenkins.
Example with GitHub Actions
yaml
Placeholder for CI pipeline image in GitHub Actions: Screenshot of GitHub Actions dashboard showing a pipeline running tests in multiple Node.js versions
Test Maintenance
Regularly Review and Update
Tests should be reviewed and updated regularly to reflect changes in the codebase. Keeping the test suite up-to-date ensures it remains relevant and useful.
Remove Obsolete Tests
Tests that are no longer relevant or that test removed features should be removed to avoid confusion and unnecessary maintenance.
Use Linters and Formatters
Use tools like ESLint and Prettier to maintain code style consistency within your tests. This not only makes the code more readable but also reduces issues arising from style inconsistencies.
json
Report Generation and Analysis
Test Reports
Generate test reports in formats compatible with your CI/CD tools. Use reporters like mocha-junit-reporter
for JUnit.
json
Code Coverage Analysis
Use code coverage analysis tools like nyc
or istanbul
to get insights into how much of your code is being covered by your tests.
sh
json
Placeholder for code coverage analysis image: Screenshot of a code coverage report showing percentage coverage and highlighted code lines
Conclusion
Adopting and maintaining best practices in writing and organizing tests with Mocha ensures that your test suite is robust, maintainable, and effective. By following these practices, you can ensure that your code remains high quality and that issues are detected and corrected quickly and efficiently.
Placeholder for image summarizing best practices: Diagram illustrating the best practices discussed, from test organization to report generation
In the final chapter, we will conclude our course by reviewing key points and suggesting next steps for continuing your journey in testing with Mocha.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
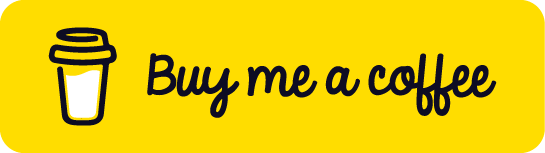
Chat with Chuck
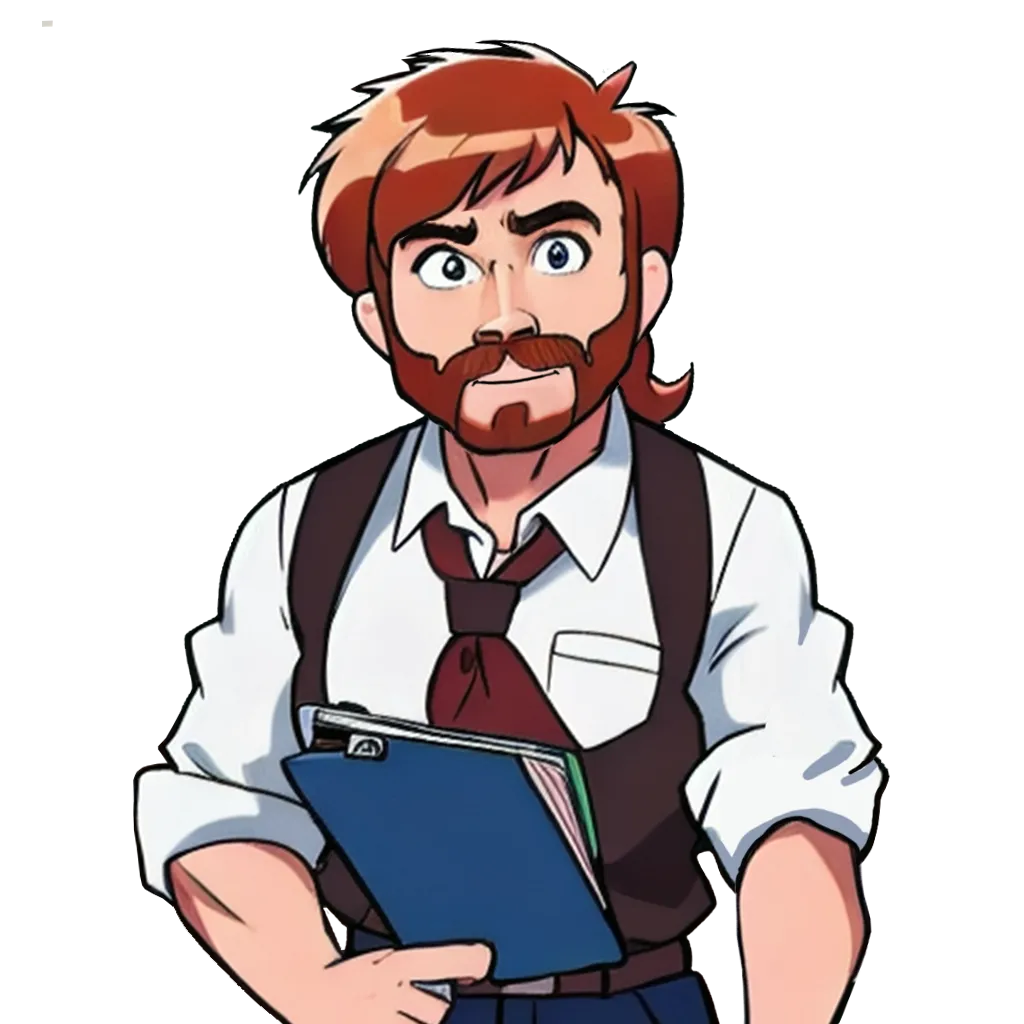
- Introduction to Testing in JavaScript with Mocha
- Fundamentals of the DOM
- Installation and Configuration of Mocha
- Writing Your First Unit Tests with Mocha
- Testing with Mocha and Chai
- DOM Component Testing with Mocha
- DOM Event Testing with Mocha
- Mocking and Stubbing with Sinon in Mocha
- User Interaction Testing with Mocha
- Accessibility Testing with Mocha
- Asynchronous Testing with Mocha
- Organization and Structure of Tests in Mocha
- Automatización de pruebas con CI/CD usando Mocha
- Best Practices for Testing with Mocha
- Conclusions and Next Steps in Testing with Mocha