Testing DOM with Mocha
Mocking and Stubbing with Sinon in Mocha
When we write unit tests, it is important to isolate the code we are testing to ensure that the test does not depend on external parts or unpredictable behaviors.
This is where Sinon.js
comes into play, a powerful library for "mocking," "stubbing," and "spying" in JavaScript tests. In this chapter, we will learn how to use Sinon with Mocha to improve our tests.
Installing Sinon
First, we need to install Sinon. You can do this by running the following command at the root of your project:
sh
Stubbing with Sinon
"Stubbing" allows us to replace a function or method with a controlled and predictable version. This is useful when we want to avoid side effects or ensure that the code under test behaves in a specific way.
Function Stubbing Example
Consider the following code in usuario.js
:
javascript
We want to test a function that uses fetchUsuario
, but we don't want to make real API calls. We can stub fetchUsuario
using Sinon.
Writing the Test with Stubbing
javascript
Stubbing Asynchronous Functions
For asynchronous functions, we can return a resolved promise:
javascript
javascript
Mocking with Sinon
"Mocking" simulates a complete object and allows setting expectations on how it should be used. Mocks are useful for verifying specific interactions.
Mocking Example in Sinon
javascript
Writing the Test with Mocking
javascript
Using Spies with Sinon
"Spies" allow us to observe the behavior of functions without replacing them. This is useful to verify if a function has been called and with what arguments.
Spying Example
javascript
Conclusion
Sinon.js
provides powerful tools for stubbing, mocking, and spying, allowing us to write more robust and isolated unit tests. The combination of Sinon with Mocha and Chai allows us to cover a wide range of test cases and verify all expected interactions in our code.
In the next chapter, we will see how to perform user interaction tests with Mocha, implementing many of the concepts we learned here.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
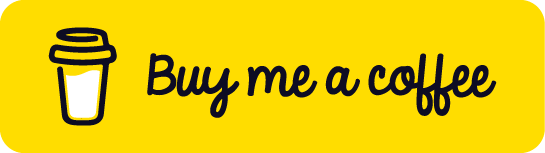
Chat with Chuck
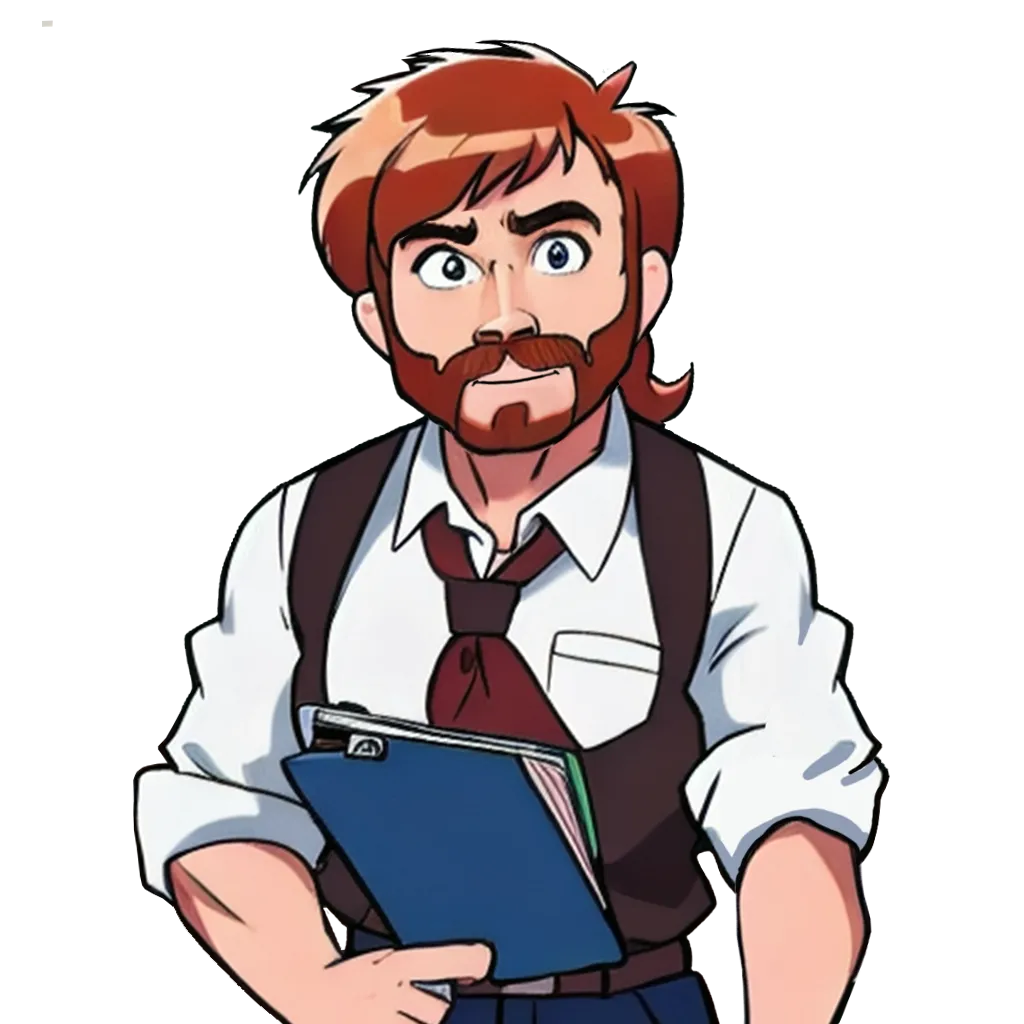
- Introduction to Testing in JavaScript with Mocha
- Fundamentals of the DOM
- Installation and Configuration of Mocha
- Writing Your First Unit Tests with Mocha
- Testing with Mocha and Chai
- DOM Component Testing with Mocha
- DOM Event Testing with Mocha
- Mocking and Stubbing with Sinon in Mocha
- User Interaction Testing with Mocha
- Accessibility Testing with Mocha
- Asynchronous Testing with Mocha
- Organization and Structure of Tests in Mocha
- Automatización de pruebas con CI/CD usando Mocha
- Best Practices for Testing with Mocha
- Conclusions and Next Steps in Testing with Mocha