Testing DOM with Mocha
Asynchronous Testing with Mocha
Asynchronous testing is an essential part of testing in modern applications, where we frequently interact with APIs, databases, and other operations that are not immediate. Mocha offers several ways to handle these situations, facilitating the writing of reliable tests for asynchronous code.
Handling Asynchronous Tests with Mocha
Callbacks
Mocha allows you to specify a done
parameter in the test function. This parameter is a function that should be called when the asynchronous code is complete.
Example with Callbacks
Suppose we have a function that fetches data asynchronously and takes a callback:
javascript
We can write a test using callbacks as follows:
javascript
Promises
Mocha also supports promises natively. If the test function returns a promise, Mocha will wait for it to resolve or reject.
Example with Promises
Suppose we have a function that returns a promise:
javascript
We can write a test using promises as follows:
javascript
Async/Await
The async/await
style is a more modern and readable way to handle asynchronous code and is fully supported in Mocha.
Example with Async/Await
We will use the same example as before, but this time with async/await
:
javascript
Handling Errors in Asynchronous Tests
It's important to ensure that Mocha captures any errors that occur during asynchronous operations.
Rejected Promises
To handle rejected promises, you can use .catch
and make sure to pass the error to Mocha:
javascript
Async/Await with try/catch
When using async/await
, you can use try/catch
to handle errors:
javascript
Testing Asynchronous Functions Dependent on Third Parties
Often, our asynchronous functions integrate third-party services. Using sinon
to stub these dependencies can help keep our tests isolated.
Example with Sinon and Promises
Suppose we have a function that fetches data from a third-party API:
javascript
We can stub the API call with sinon
for our tests:
javascript
Conclusion
Mocha makes it easy to handle asynchronous tests using callbacks, promises, and async/await. These tools are essential for testing the behavior of modern applications that rely heavily on asynchronous operations. Additionally, combining Mocha with tools like Sinon can help you achieve more robust and controlled tests.
In the next chapter, we will delve into how to structure and organize your tests in Mocha to maintain clean and manageable test code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
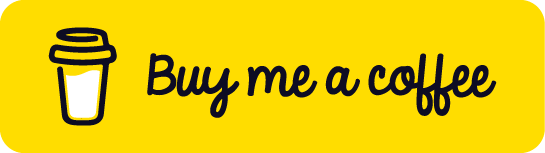
Chat with Chuck
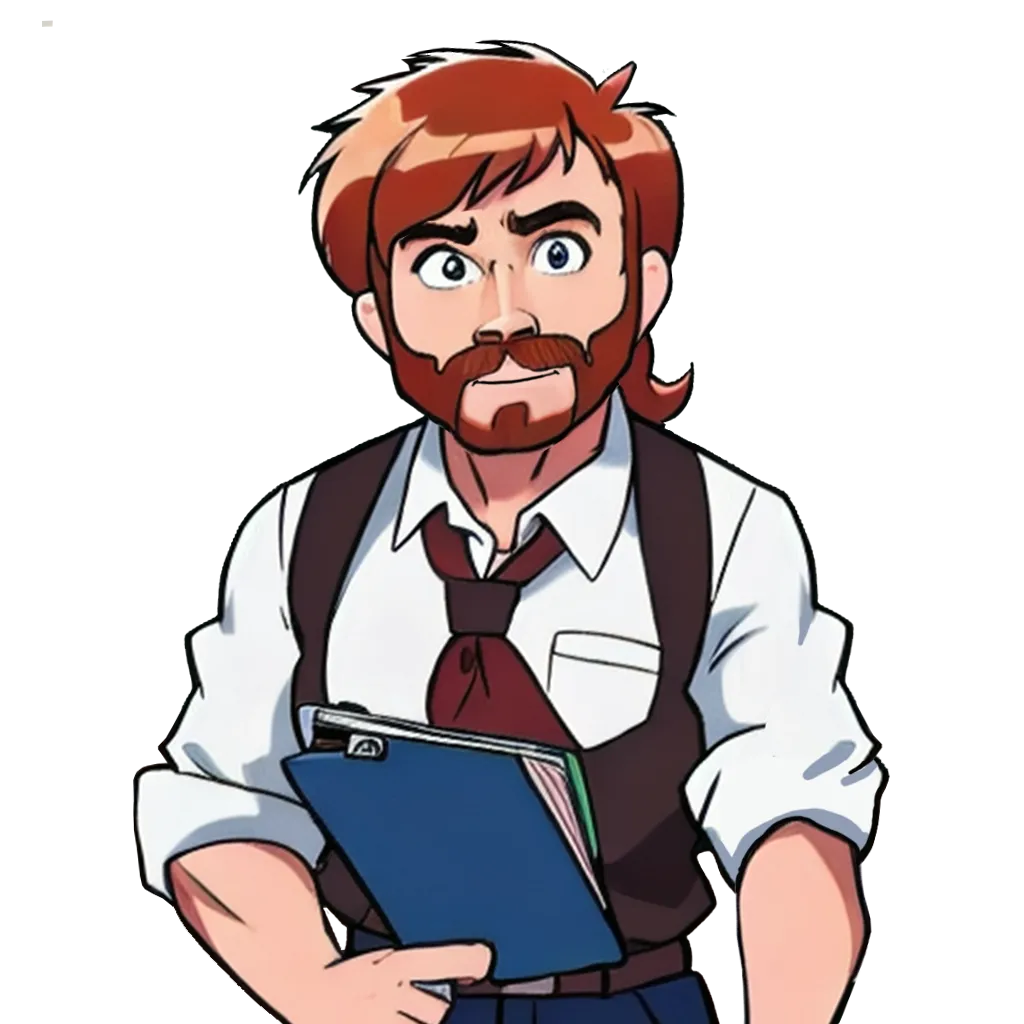
- Introduction to Testing in JavaScript with Mocha
- Fundamentals of the DOM
- Installation and Configuration of Mocha
- Writing Your First Unit Tests with Mocha
- Testing with Mocha and Chai
- DOM Component Testing with Mocha
- DOM Event Testing with Mocha
- Mocking and Stubbing with Sinon in Mocha
- User Interaction Testing with Mocha
- Accessibility Testing with Mocha
- Asynchronous Testing with Mocha
- Organization and Structure of Tests in Mocha
- Automatización de pruebas con CI/CD usando Mocha
- Best Practices for Testing with Mocha
- Conclusions and Next Steps in Testing with Mocha