Testing DOM with Mocha
Installation and Configuration of Mocha
To start writing tests with Mocha, we first need to install and configure the framework. This chapter will guide you through the installation process, basic configuration, and running your first set of tests.
Prerequisites
Before we start, make sure you have Node.js and npm installed on your system. You can download Node.js from nodejs.org.
Installing Mocha
Mocha is easily installed using npm. There are two ways to install Mocha: globally or locally as a development dependency in your project. Local installation is generally the best practice as it ensures that the project uses the same version of Mocha regardless of the environment.
Local Installation
To install Mocha locally in your project, navigate to your project's root directory in the terminal and run the following command:
sh
This will download Mocha and add it to the development dependencies in your package.json
.
Global Installation
If you want to install Mocha globally, you can do so by running:
sh
Configuring Mocha
Project Structure
It is recommended to have an organized project structure to facilitate management of your tests. A typical structure might be:
Test Script Configuration
To simplify running the tests, it is useful to add a test script in the package.json
. Open your package.json
file and add the following script:
json
This will allow you to run your tests simply by using the command npm test
in your terminal.
Writing Your First Test
Test File
Create a test file named example.test.js
in the test/
directory.
javascript
Running the Tests
To run the tests, simply navigate to the root of your project in the terminal and execute:
sh
You should see an output in the terminal indicating that Mocha has run the tests. If everything is set up correctly, you should see something like this:
Advanced Configuration
Mocha also allows for more advanced configurations, such as defining a .mocharc.json
configuration file to customize Mocha's behavior.
Mocha Configuration File
You can create a configuration file mocharc.json
in the root of your project:
json
In this example, we are using Babel to transpile our code before running it.
Conclusion
You have now successfully set up and run your first test with Mocha. In the upcoming chapters, we will explore how to write more complex tests, how to integrate Mocha with other tools, and how to apply best practices to ensure the quality of your code.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
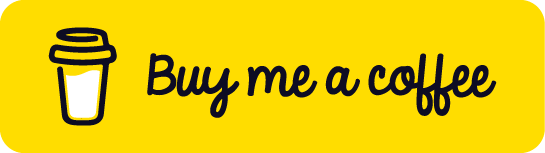
Chat with Chuck
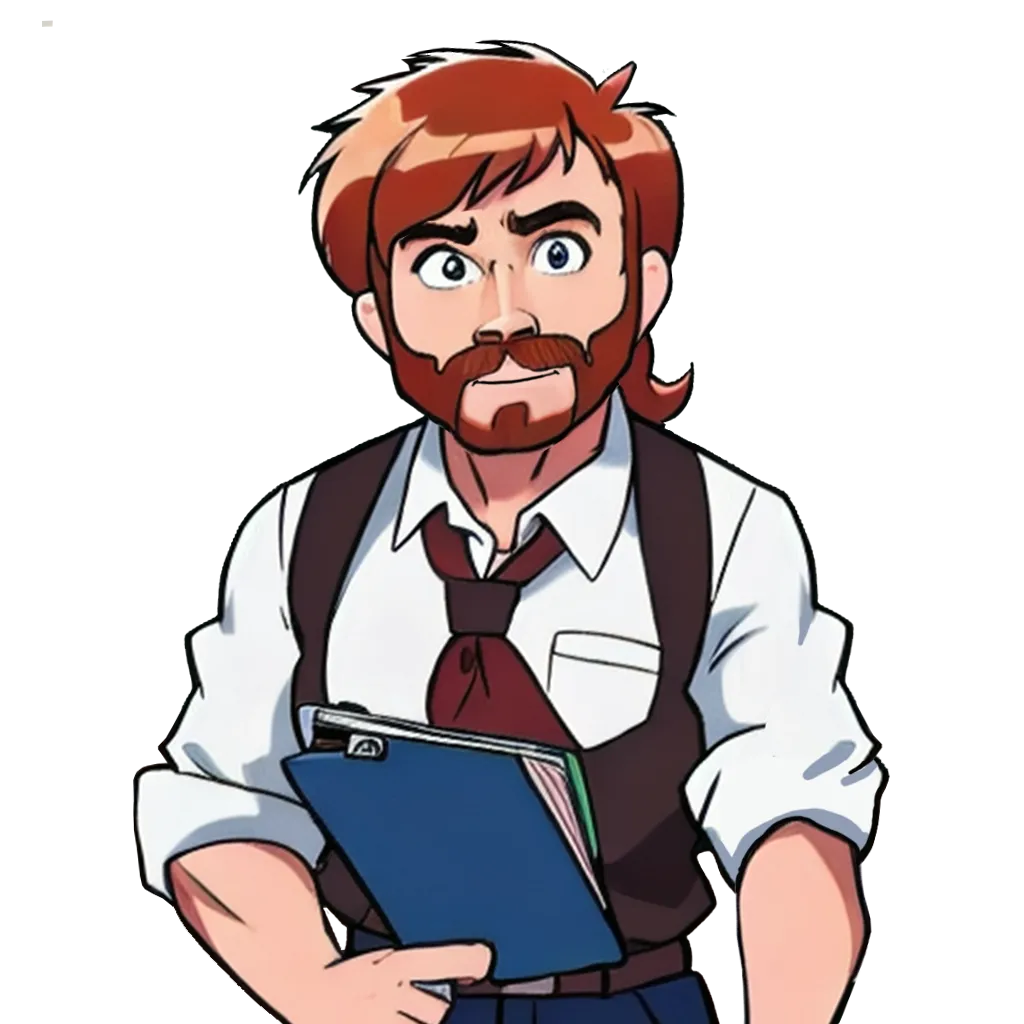
- Introduction to Testing in JavaScript with Mocha
- Fundamentals of the DOM
- Installation and Configuration of Mocha
- Writing Your First Unit Tests with Mocha
- Testing with Mocha and Chai
- DOM Component Testing with Mocha
- DOM Event Testing with Mocha
- Mocking and Stubbing with Sinon in Mocha
- User Interaction Testing with Mocha
- Accessibility Testing with Mocha
- Asynchronous Testing with Mocha
- Organization and Structure of Tests in Mocha
- Automatización de pruebas con CI/CD usando Mocha
- Best Practices for Testing with Mocha
- Conclusions and Next Steps in Testing with Mocha