Testing DOM with Mocha
Introduction to Testing in JavaScript with Mocha
Modern software development requires a constant guarantee of quality. One of the best ways to ensure the quality of your code is through automated testing. In this context, Mocha emerges as a powerful tool for testing JavaScript.
What is Mocha?
Mocha is a testing framework for JavaScript that runs on Node.js and in the browser, making it a versatile tool for developing both backend and frontend tests. It was designed to be simple and flexible, allowing developers to create unit and integration tests efficiently.
Advantages of Using Mocha
- Flexibility: Mocha allows integration with multiple assertion libraries such as Chai, Should.js, and many more.
- Editor-agnostic: It is not tied to any specific editor, so it can be used with your preferred development environment.
- Support for asynchronous testing: It offers native support for asynchronous tests, crucial in modern applications.
- Customizable report output: It allows the use of various "reporters" to obtain test results in different formats.
What can we test with Mocha?
Mocha is not limited to a single type of test; it can be used for a wide range of test cases, such as:
- Unit tests: Validate specific and independent functionalities of the code.
- Integration tests: Ensure that different modules work well together.
- DOM component tests: Verify that UI components behave as expected.
- Event and user interaction tests: Confirm that the system responds correctly to user interactions.
Next, let's look at basic examples to better understand how Mocha works.
Example 1: A basic test in Mocha
javascript
In this example, we use describe
to group a set of related tests, and it
to define what the test should do. assert.strictEqual
is an assertion that checks that the index of 4 in the array [1, 2, 3]
is -1, meaning it is not present.
Example 2: Asynchronous test in Mocha
javascript
In this case, we use done
to inform Mocha when the asynchronous test has finished. This is crucial for tests that involve waiting times or API calls.
Conclusion
Mocha is an essential tool for any JavaScript developer looking to improve the quality of their code through testing. In the following chapters, we will delve deeper into each of the mentioned concepts and learn to use Mocha in conjunction with other tools and advanced testing practices.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
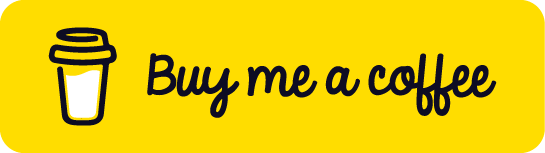
Chat with Chuck
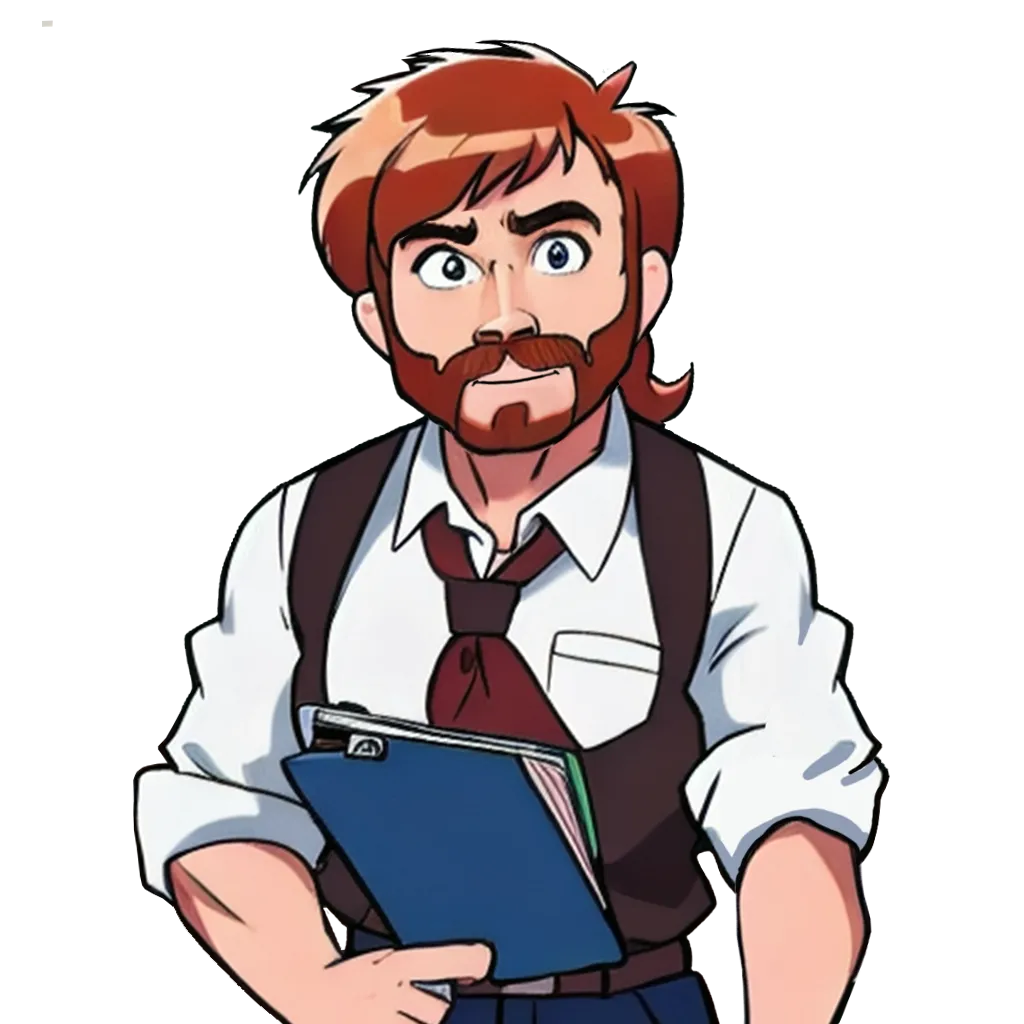
- Introduction to Testing in JavaScript with Mocha
- Fundamentals of the DOM
- Installation and Configuration of Mocha
- Writing Your First Unit Tests with Mocha
- Testing with Mocha and Chai
- DOM Component Testing with Mocha
- DOM Event Testing with Mocha
- Mocking and Stubbing with Sinon in Mocha
- User Interaction Testing with Mocha
- Accessibility Testing with Mocha
- Asynchronous Testing with Mocha
- Organization and Structure of Tests in Mocha
- Automatización de pruebas con CI/CD usando Mocha
- Best Practices for Testing with Mocha
- Conclusions and Next Steps in Testing with Mocha