Testing DOM with Mocha
Fundamentals of the DOM
The DOM (Document Object Model) is a programming interface that allows programming languages to interact with HTML and XML documents. It functions as a structured representation of the document and defines methods that enable scripts to modify its content, structure, and style.
What is the DOM?
The DOM represents an HTML document as a hierarchy of nodes, where each node corresponds to a part of the document. Nodes can be elements, attributes, texts, comments, and more. This hierarchical structure allows developers to access and manipulate HTML elements programmatically.
DOM Nodes
The different types of nodes in the DOM include:
- Element Nodes: Represent HTML tags (e.g.,
<div>
,<p>
). - Text Nodes: Contain the text within elements.
- Attribute Nodes: Represent the attributes of HTML elements.
- Comment Nodes: Contain comments in the HTML.
DOM Manipulation
JavaScript provides various methods to manipulate the DOM. Here are some common examples:
Accessing Elements
javascript
Modifying Content
javascript
Manipulating Styles
javascript
Creating and Removing Elements
javascript
DOM Events
Events are an integral part of interacting with the DOM. You can use JavaScript to listen to and react to different types of events, such as mouse clicks, key presses, and more.
Event Example
javascript
Removing Event Listeners
javascript
Conclusion
Understanding the fundamentals of the DOM is crucial for any web developer. The ability to manipulate the DOM allows us to create dynamic and interactive web applications. In the upcoming chapters, we will see how this DOM manipulation can be applied in the context of automated testing using Mocha.
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
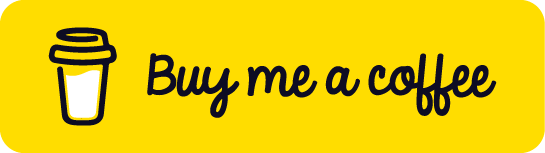
Chat with Chuck
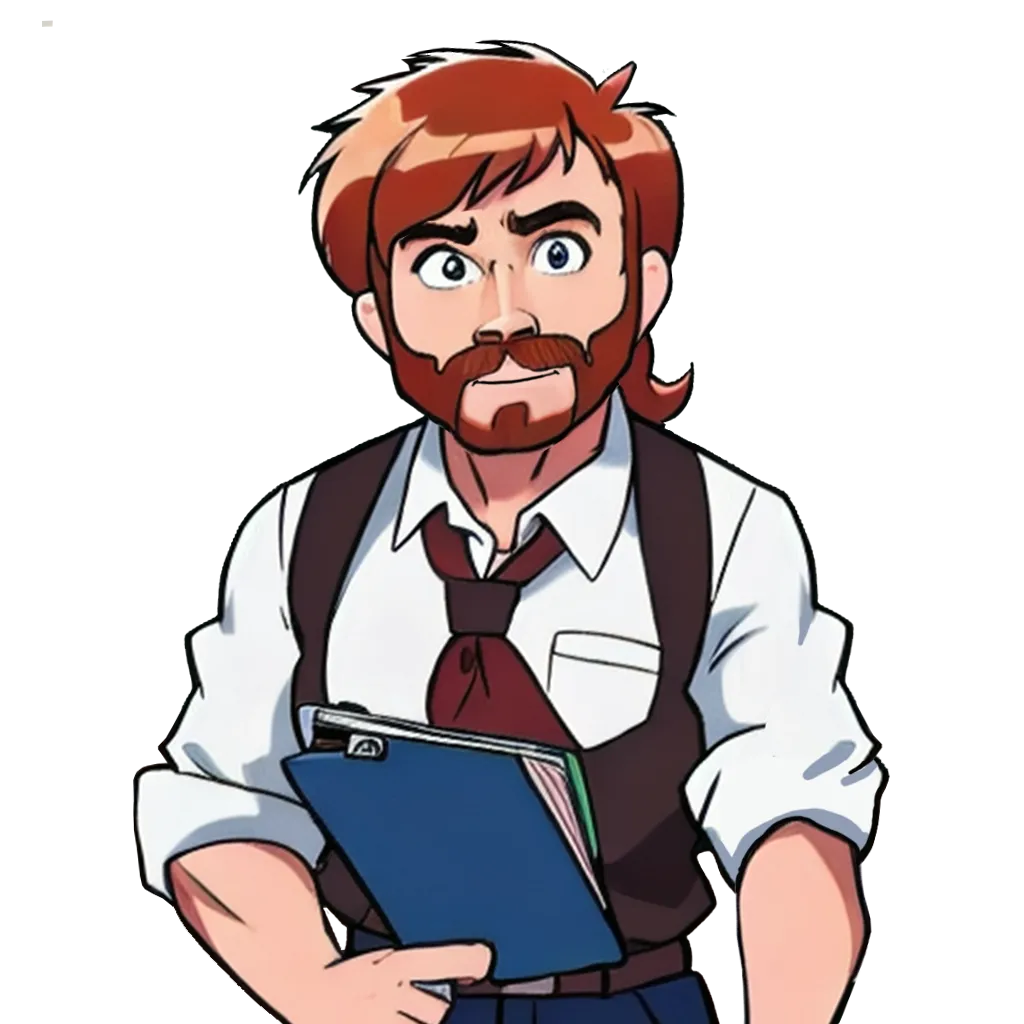
- Introduction to Testing in JavaScript with Mocha
- Fundamentals of the DOM
- Installation and Configuration of Mocha
- Writing Your First Unit Tests with Mocha
- Testing with Mocha and Chai
- DOM Component Testing with Mocha
- DOM Event Testing with Mocha
- Mocking and Stubbing with Sinon in Mocha
- User Interaction Testing with Mocha
- Accessibility Testing with Mocha
- Asynchronous Testing with Mocha
- Organization and Structure of Tests in Mocha
- Automatización de pruebas con CI/CD usando Mocha
- Best Practices for Testing with Mocha
- Conclusions and Next Steps in Testing with Mocha