HTML5 Geolocation API
Advanced Options in Geolocation
So far, we have learned to obtain the user's basic location using the Geolocation API. However, in some applications, it is necessary to control more precisely the accuracy, timeout, and update frequency of the location. In this chapter, we will explore the advanced options available to customize location requests and improve user experience.
Configuring Options with positionOptions
The Geolocation API allows adjusting the location request using an options object called positionOptions
. This object can be passed as a third parameter to the getCurrentPosition
or watchPosition
methods, and it allows configuring three important properties:
enableHighAccuracy
: If set totrue
, it indicates to the device that it should use the highest possible accuracy for the location. This can imply higher battery and data consumption.timeout
: Defines the maximum time in milliseconds that the API will wait before returning an error if it does not obtain the location.maximumAge
: Specifies the maximum time in milliseconds that a cached position is considered valid before requesting an update.
Advanced Configuration Example
Let's see how to implement positionOptions
for a high-accuracy request with a timeout of five seconds and without using cached positions.
javascript
Explanation of Each Parameter
enableHighAccuracy
When enableHighAccuracy
is activated, the device will attempt to obtain the location with the highest possible accuracy. This means it is likely to use GPS if available, instead of relying on Wi-Fi networks or IP data which are less precise. However, keep in mind that this setting may increase battery consumption.
javascript
timeout
The timeout
parameter specifies the maximum time the application will wait for a location response before failing. This value is useful in applications that require quick responses and cannot wait indefinitely.
javascript
maximumAge
The maximumAge
parameter defines how old a cached location can be before the API requests a new location. This parameter is useful in applications where real-time location accuracy is not needed, helping conserve battery.
javascript
Combining Options for a Customized Experience
The enableHighAccuracy
, timeout
, and maximumAge
options can be combined according to the application's needs. For example, in a real-time navigation application, it is useful to enable high accuracy and set a low maximumAge
to get the most up-to-date location data.
javascript
Advantages and Disadvantages of Using Advanced Options
Advantages
- Greater Control: Applications have more control over the accuracy and update frequency of the location.
- Better User Experience: Allows adjustment of the location request to specific needs, optimizing battery and data usage.
- Lower Resource Consumption: With
maximumAge
, location requests can be reduced, thus conserving the device's battery.
Disadvantages
- Higher Battery Consumption: Using high accuracy and frequent updates can drain the battery quickly, especially on mobile devices.
- Slow Response Time: In some cases, high accuracy can result in a longer response time, as the device searches for GPS signals.
Practical Example: Configuration for Different Scenarios
Let's see an example where we use different positionOptions
configurations depending on the scenario:
javascript
Chapter Summary
In this chapter, we learned to use advanced geolocation options, such as enableHighAccuracy
, timeout
, and maximumAge
, to customize location requests. By adapting these settings to the application's specific needs, it is possible to improve both the data accuracy and the user experience.
In the next chapter, we will address error handling in the Geolocation API, an important part of creating reliable and robust applications that can adequately respond to different issues that may arise during the geolocation process.
Let's move forward and learn how to handle geolocation errors in the next chapter!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
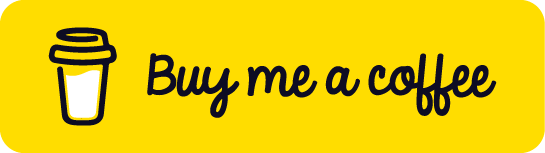
Chat with Chuck
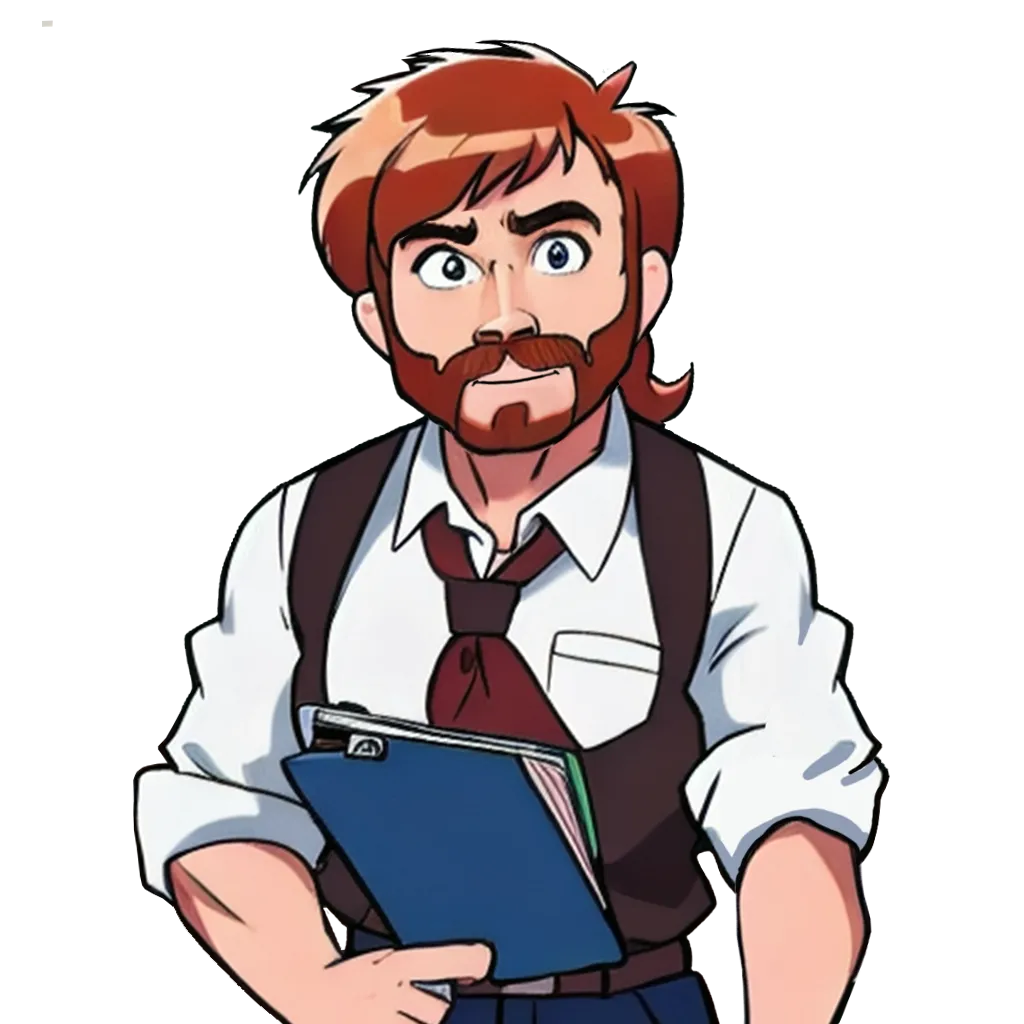
- Introduction to the Geolocation API
- Requesting User Location and Managing Permissions
- Retrieving Location Coordinates
- Advanced Options in Geolocation
- Handling Errors in the Geolocation API
- User Movement Tracking with `watchPosition`
- Integration of Geolocation with Maps
- Practical Project: Geofencing Implementation
- Troubleshooting Common Problems in Geolocation
- Conclusion and Next Steps