HTML5 Geolocation API
Handling Errors in the Geolocation API
In the implementation of applications that use the Geolocation API, it is essential to properly handle errors that may occur when trying to obtain the user's location. Errors can occur for various reasons, such as connection issues, denial of permissions, or lack of access to location data. In this chapter, we will learn to identify and manage these errors to ensure a robust and user-friendly experience.
Types of Errors in Geolocation
The Geolocation API defines three main types of errors, which can be identified through the error code returned in the error handling function (errorCallback
):
- 1 -
PERMISSION_DENIED
: This error occurs when the user denies permission to access their location. - 2 -
POSITION_UNAVAILABLE
: This error indicates that the device cannot determine the current location, either due to connectivity issues or because no location data is available. - 3 -
TIMEOUT
: Occurs when the time specified intimeout
has elapsed without receiving a location response.
Basic Implementation of Error Handling
When implementing a location request, it is advisable to use an error handling function (errorCallback
) that contains a switch
to identify the type of error and respond appropriately.
javascript
Example of Custom Error Messages
It is possible to enhance the user experience by providing more detailed error messages or alternative solutions. For example, if the error is PERMISSION_DENIED
, we can offer instructions on how to grant permissions in the browser settings. For POSITION_UNAVAILABLE
, the user could be advised to check their network connection or enable GPS.
javascript
Logging Errors for Debugging
In complex applications, it is useful to log errors for debugging or monitoring purposes. This allows developers to analyze error patterns and improve application performance. A common practice is to log errors to the console or send them to an analytics server.
javascript
Best Practices in Geolocation Error Handling
To provide a smooth user experience and minimize frustration, consider the following best practices:
- Display Clear Messages: Users should easily understand why the error occurred and what steps to take.
- Offer Alternative Solutions: If the location cannot be obtained, allow the user to enter their location manually or proceed without it.
- Log Errors: Logging can help developers improve the application's functionality and reliability.
- Avoid Repetitive Requests: If the user has denied permissions, avoid repeatedly requesting location, as this can be bothersome.
Implementing a Recovery Strategy
Below is a complete example that implements an error recovery strategy. This strategy offers options to the user in case of location failures, allowing greater flexibility.
javascript
Chapter Summary
In this chapter, we learned how to identify and handle errors in the Geolocation API. We explored different error codes, strategies for customizing messages and offering alternative solutions, and how to log errors to improve debugging. These techniques enable the development of more user-friendly and resilient applications.
In the next chapter, we will see how to use the watchPosition
method to continuously track the user's location, an ideal tool for real-time applications such as navigation.
Let's move forward and discover how to perform real-time tracking with the Geolocation API!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
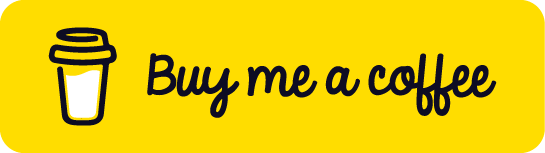
Chat with Chuck
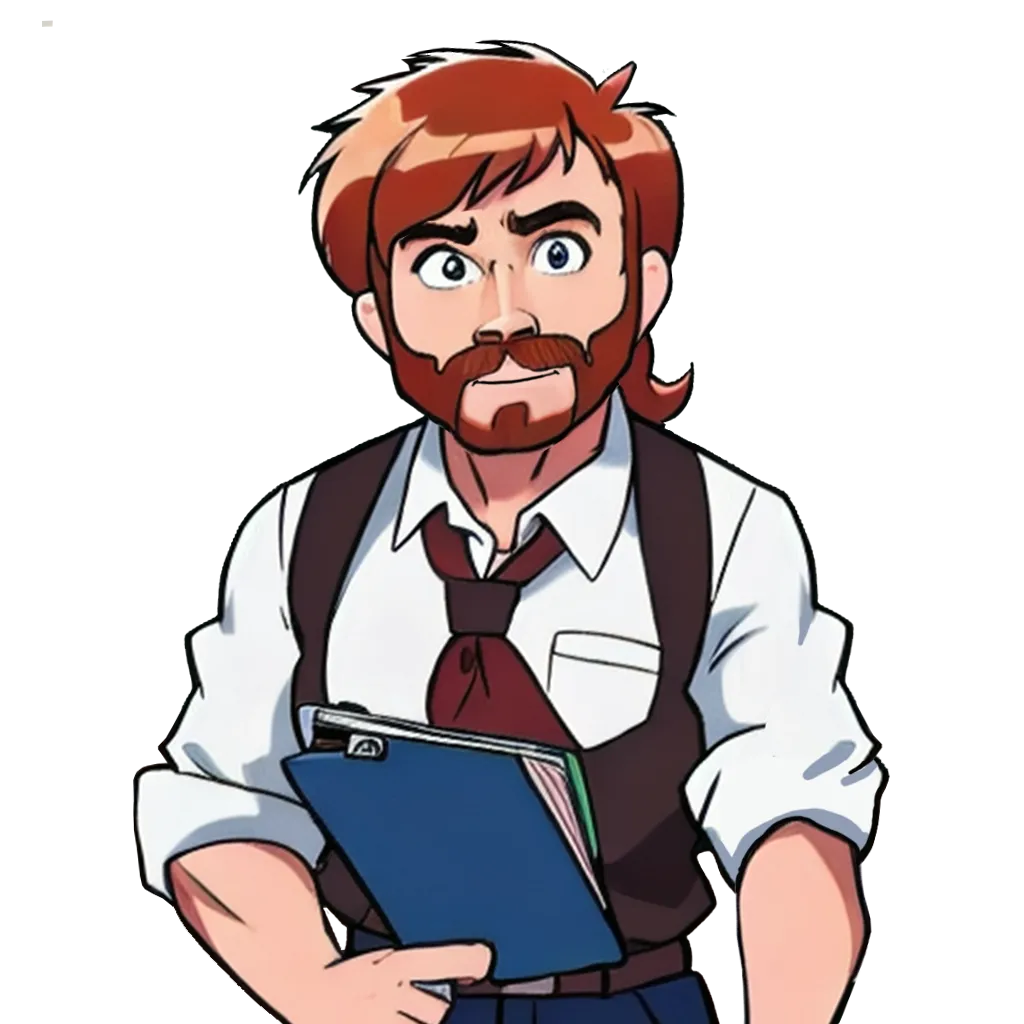
- Introduction to the Geolocation API
- Requesting User Location and Managing Permissions
- Retrieving Location Coordinates
- Advanced Options in Geolocation
- Handling Errors in the Geolocation API
- User Movement Tracking with `watchPosition`
- Integration of Geolocation with Maps
- Practical Project: Geofencing Implementation
- Troubleshooting Common Problems in Geolocation
- Conclusion and Next Steps