HTML5 Geolocation API
User Movement Tracking with `watchPosition`
So far, we have learned to obtain the user's location at a specific moment using the getCurrentPosition
method. However, many applications require continuous tracking of the user's position, such as real-time navigation apps or those monitoring exercise routes. For this, the Geolocation API offers the watchPosition
method, which allows receiving constant location updates. In this chapter, we will learn how to implement continuous tracking and explore some practical use cases.
What is the watchPosition
Method?
The watchPosition
method allows the application to receive periodic user location updates. Unlike getCurrentPosition
, which obtains the location once, watchPosition
runs each time there is a change in the user's location, providing updated real-time location data.
watchPosition
Syntax
javascript
Basic Implementation Example of watchPosition
In this example, we will see how to use watchPosition
to update the user's latitude and longitude in real-time, displaying the information onscreen as the user moves.
javascript
Stopping Real-Time Tracking with clearWatch
Although watchPosition
is very useful for real-time tracking, it's important to stop it when no longer needed to save battery and device resources. To do this, we use the clearWatch
method, which takes the watchId
returned by watchPosition
and stops the location updates.
javascript
Complete Example with Start and Stop Buttons
A common approach in applications is to allow the user to turn location tracking on and off using start and stop buttons.
html
Performance and Battery Usage Considerations
Continuous location tracking can consume a lot of battery, especially if the app requests high accuracy and the device uses GPS constantly. Therefore, it is important to limit the use of watchPosition
to when it is strictly necessary. Here are some recommendations:
- Activate tracking only when needed by the user: Offer an option to turn tracking on/off instead of running it automatically.
- Reduce update frequency: Use the
maximumAge
option to reduce the number of updates requested. - Deactivate when not needed: Ensure to stop tracking when leaving the view or activity where it was being used.
Advanced Configuration Example in watchPosition
For apps that need continuous tracking but seek to optimize resource usage, watchPosition
can be configured with custom options that adjust accuracy and update frequency.
javascript
Common Uses of watchPosition
Continuous location tracking is a key feature in apps that depend on real-time location:
- Navigation: Update the location on a map while the user moves.
- Exercise Apps: Monitor the progress of a route in activities like running or cycling.
- Transport Services: Track moving vehicles, like taxis or deliveries.
These applications rely on updated location data to provide a dynamic and accurate experience.
Chapter Summary
In this chapter, we explored how to implement continuous location tracking with the watchPosition
method, a powerful tool for navigation and real-time tracking apps. We also covered how to optimize performance and stop tracking when not needed, maintaining a balance between precision and efficiency.
In the next chapter, we will see how to integrate geolocation data with map tools, allowing the user's location to be visually displayed on a map.
Let's move forward and discover how to integrate maps with the Geolocation API in the next chapter!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
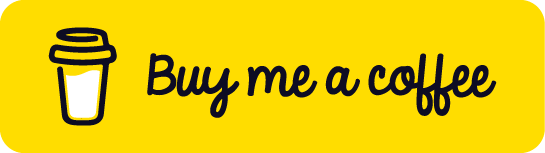
Chat with Chuck
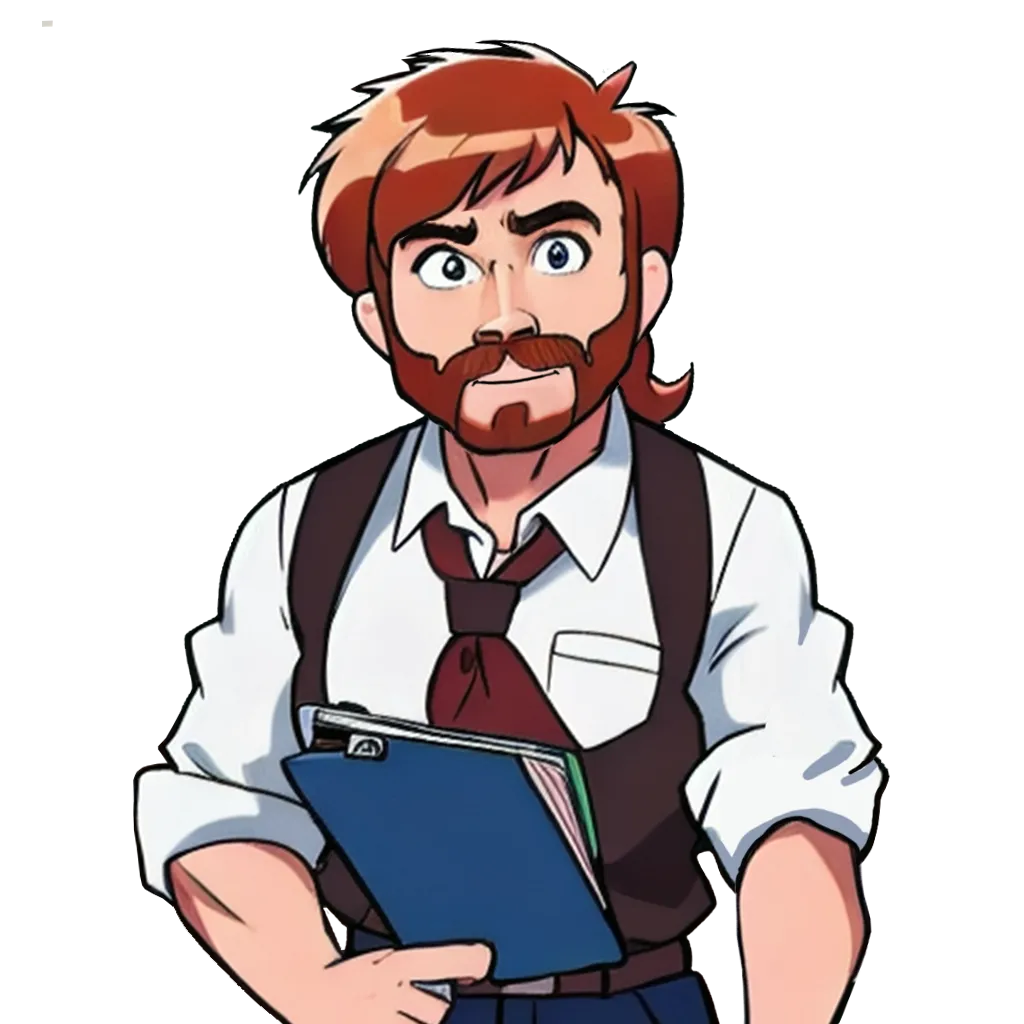
- Introduction to the Geolocation API
- Requesting User Location and Managing Permissions
- Retrieving Location Coordinates
- Advanced Options in Geolocation
- Handling Errors in the Geolocation API
- User Movement Tracking with `watchPosition`
- Integration of Geolocation with Maps
- Practical Project: Geofencing Implementation
- Troubleshooting Common Problems in Geolocation
- Conclusion and Next Steps