HTML5 Geolocation API
Integration of Geolocation with Maps
Once we obtain the user's location data, a common way to use it is by displaying their position on a map. This integration is key in navigation applications, location services, and many other web applications. In this chapter, we will learn how to combine the Geolocation API with map services, such as Google Maps, to display the user's location in a visual environment.
Choosing a Map Service
There are several map services that can be integrated with the Geolocation API, each with its own advantages. Some of the most common services include:
- Google Maps: Offers a wide set of tools and a robust API to customize the map, add markers, and other elements.
- OpenStreetMap: An open-source map service providing a good free and customizable alternative.
- Mapbox: Offers a versatile and highly customizable API suitable for advanced applications.
For this chapter, we will use Google Maps as an example due to its extensive documentation and functionalities.
Setting Up Google Maps
Before we can integrate Google Maps into our application, we need an API key. You can get it by signing up at Google Cloud Platform. Once you have the key, add it to the Google Maps script in your HTML file.
html
Displaying the User's Location on the Map
With the Google Maps script added, we can create a map and place a marker at the user's location. First, we need a container for the map in our HTML.
html
Next, we initialize the map in JavaScript and center the view on the user's current location.
javascript
Integrating the Map with Geolocation
Now, let's combine this map function with the Geolocation API to automatically get the user's location and display it on the map.
javascript
Map Customization
Google Maps offers multiple customization options to make the map fit the application's design and needs. Here are some of the most common options:
- Zoom Control: Controls the map's zoom level when initialized.
- Map Styles: Google Maps allows applying custom styles to change the map's appearance.
- Map Types: Map types include
roadmap
,satellite
,hybrid
, andterrain
.
For example, here we customize the map to show a satellite view and remove some visual elements:
javascript
Continuous Location Update on the Map
For applications that require real-time tracking, such as navigation apps, we can use watchPosition
together with Google Maps to update the user's location as they move.
javascript
Integration with Other Map Functions
In addition to displaying the user's location, map services offer various useful functionalities to enhance the experience:
- Route Calculation: Services like Google Maps allow calculating routes between two locations.
- Points of Interest: Add nearby points like restaurants, gas stations, and stores.
- Geocoding: Convert addresses into geographic coordinates and vice versa.
Route Calculation Example
Using the Google Maps Directions API, we can calculate and display a route on the map from the user's location to a destination.
javascript
Chapter Summary
In this chapter, we learned how to integrate the Geolocation API with map services to visually display the user's location. We explored how to set up and customize Google Maps, as well as updating the location in real-time. We also saw how to use additional functions, such as route calculation, to enrich the user experience.
In the next chapter, we will develop a practical project to put all these concepts into practice, integrating geolocation and maps into a functional web application.
Let's move forward and apply everything we've learned in a final project in the next chapter!
Support Chuck’s Academy!
Enjoying this course? I put a lot of effort into making programming education free and accessible. If you found this helpful, consider buying me a coffee to support future lessons. Every contribution helps keep this academy running! ☕🚀
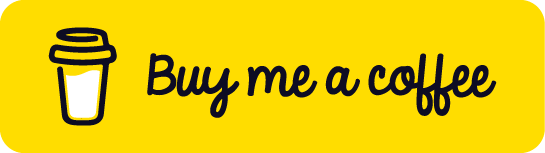
Chat with Chuck
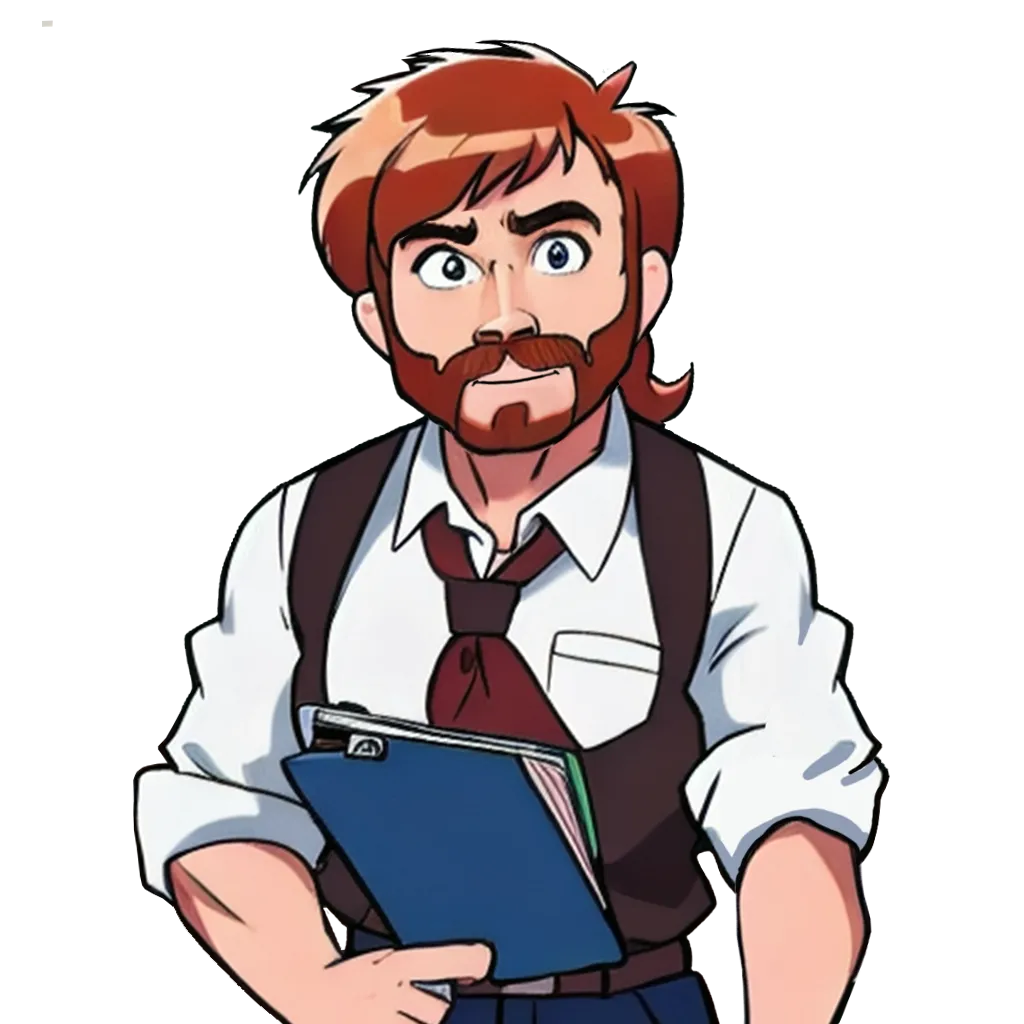
- Introduction to the Geolocation API
- Requesting User Location and Managing Permissions
- Retrieving Location Coordinates
- Advanced Options in Geolocation
- Handling Errors in the Geolocation API
- User Movement Tracking with `watchPosition`
- Integration of Geolocation with Maps
- Practical Project: Geofencing Implementation
- Troubleshooting Common Problems in Geolocation
- Conclusion and Next Steps